- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10711 Articles for Web Development
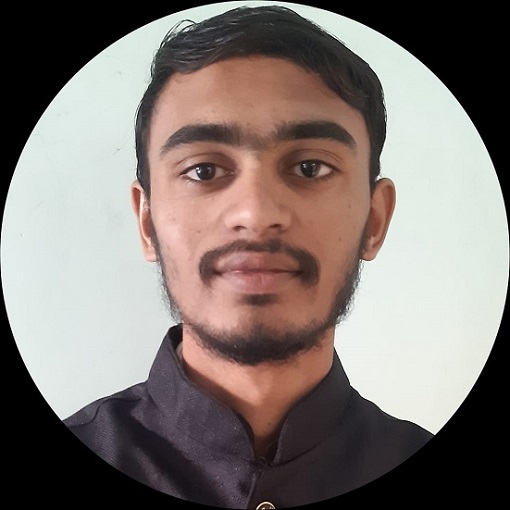
4K+ Views
In this tutorial, let us discuss how to validate a given number in JavaScript. Users can follow the syntax below each method to use it. Using regular expression The method tests or matches the regular expression with the input. Syntax /\D/.test(input); input.match(/^[0-9]*$/); If the input is a number, the test returns true. Example The program succeeds in all cases except for "30", [], and "". Number validation using regular expression Validate ... Read More

537 Views
To add a number of months to a date, first get the month using getMonth() method and add a number of months.ExampleYou can try to run the following code to add a number of monthsLive Demo var d, e; d = new Date(); document.write(d); e = d.getMonth()+1; document.write("Incremented month = "+e);

153 Views
Function DeclarationThe “function” keyword declares a function in JavaScript. To define a function in JavaScript use the “function” keyword, followed by a unique function name, a list of parameters (that might be empty), and a statement block surrounded by curly braces.Here’s an example −function sayHello(name, age) { document.write (name + " is " + age + " years old."); }Function ExpressionFunction Expression should not start with the keyword “function”. Functions defined can be named or anonymous.Here are the examples −//anonymous function expression var a = function() { return 5; }Or//named function expression var a = function bar() { return 5; }

81 Views
Double equals (==) is abstract equality comparison operator, which transforms the operands to the same type before making the comparison. For example,5 == 5 //true '5' == 5 //true 5 == '5' //true 0 == false //trueTriple equals (===) are strict equality comparison operator, which returns false for different types and different content.For example,5 === 5 // true 5 === '5' // false var v1 = {'value':'key'}; var v2 = {'value': 'key'}; v1 === v2 //false

541 Views
ExampleTo parse JSON object in JavaScript, implement the following code − myData = JSON.parse('{"event1":{"title":"Employment period","start":"12\/29\/2011 10:20 ","end":"12\/15\/2013 00:00 "},"event2":{"title":"Employment period","start":"12\/14\/2011 10:20 ","end":"12\/18\/2013 00:00 "}}') myArray = [] for(var e in myData){ var dataCopy = myData[e] for(key in dataCopy){ if(key == "start" || key == "end"){ dataCopy[key] = new Date(dataCopy[key]) } } myArray.push(dataCopy) } document.write(JSON.stringify(myArray));

464 Views
The conditional operator or ternary operator first evaluates an expression for a true or false value and then executes one of the two given statements depending upon the result of the evaluation.S.NoOperator & Description1? : (Conditional )If Condition is true? Then value X: Otherwise value YExampleYou can try to run the following code to understand how Ternary Operator works in JavaScriptLive Demo var a = 10; var b = 20; var linebreak = ""; document.write ("((a > b) ? 100 : 200) => "); result = (a > b) ? 100 : 200; document.write(result); document.write(linebreak); document.write ("((a < b) ? 100 : 200) => "); result = (a < b) ? 100 : 200; document.write(result); document.write(linebreak);

1K+ Views
To pass a parameter to setTimeout() callback, use the following syntax −setTimeout(functionname, milliseconds, arg1, arg2, arg3...)The following are the parameters −function name − The function name for the function to be executed.milliseconds − The number of milliseconds.arg1, arg2, arg3 − These are the arguments passed to the function.ExampleYou can try to run the following code to pass a parameter to a setTimeout() callbackLive Demo Submit function timeFunction() { setTimeout(function(){ alert("After 5 seconds!"); }, 5000); } Click the above button and wait for 5 seconds.

121 Views
To execute an anonymous function you need to use the leading bang! or any other symbol −!function(){ // do stuff }();You can also write it like −+function(){ // do stuff }();Even the following is an acceptable syntax −~function(){ // do stuff return 0; }( );

66 Views
To determine if an argument is sent to function, use “default” parameters.ExampleYou can try to run the following to implement itLive Demo function display(arg1 = 'Tutorials', arg2 = 'Learning') { document.write(arg1 + ' ' +arg2+""); } display('Tutorials', 'Learning'); display('Tutorials'); display();

1K+ Views
To call multiple JavaScript functions in on click event, use a semicolon as shown below −onclick="Display1();Display2()"ExampleLive Demo function Display1() { document.write ("Hello there!"); } function Display2() { document.write ("Hello World!"); } Click the following button to call the function