- Trending Categories
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 34469 Articles for Programming

119 Views
In computer science we define decimal numbers to be the numbers which utilize base10 notations. For example 12, 4, 5.7, 99 are decimal numbers because they utilize base 10 notation. We can define the length of a decimal number as the number of digits present in the decimal number. More precisely, the number of significant figures present in the decimal number includes both digits after and before the decimal parts. This article will explore several methods to find the k−length decimal places like the round method, String formatting, Global precisions, etc. Using round Method One of the ... Read More

996 Views
Consecutive characters are those characters that appear one after the other. k length consecutive characters mean the same character appearing k times consecutively. In this article, we will adopt several methods to achieve it. We will start with brute force by using loop statements. Next, we will perform the same using regular expressions, sliding window techniques, etc. A sliding window is a better and optimized way to find the k-length consecutive characters. Numpy Library also offers us methods to adopt similar techniques. Using the Brute Force Method Brute force is a simple algorithm that we can think about without ... Read More

228 Views
K length combinations from given characters mean the combinations of characters that we can create with the given characters, which are exactly of length k. In this article, we will explore several methods to achieve this, like recursion, map and lambda function, itertools library, etc. While recursion and lambda functions are custom functions, the itertools provide the in-built method to generate the combinations. Using Recursion Recursion is one of the traditional programming techniques. In this technique, we try to break a large problem into smaller chunks of problems, and our motive is to solve the smaller problems to solve ... Read More

1K+ Views
Understanding function signatures in Python is essential for unraveling the inner workings of functions. The inspect module offers a range of methods to retrieve signatures, allowing you to analyze parameters, access data types, and determine default values. By exploring these techniques, you can gain valuable insights that enhance code readability, maintainability, and documentation. So, dive into the world of function signatures and unlock a deeper understanding of your Python code. Using inspect Method The inspect method provides a powerful way to retrieve the function signatures. This method enables us to access detailed information about a function's parameters, data types, default ... Read More

152 Views
The Tuple is one of the most important data types in Python. They are exclusively used as the data structures to pass sequential data as the parameters of any method. Indexing refers to accessing the elements of the sequential data through the index. In this article, we will learn how to get an even index in elements of Tuple. Use The List And range Expression. The mutable lists sequences of data separated by ", " and enclosed by "[ ]". Python allows the conversion between the lists and the Tuples through the in-built methods. We can traverse the lists and ... Read More
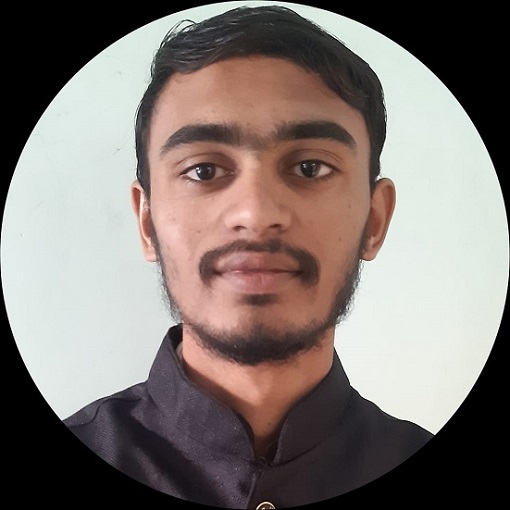
4K+ Views
In C++, the string is a collection of various alphanumeric and special characters. We can create a string using the ‘string’ data type in C++. Problem Statement We have given a length of the string and one single character, and we require to generate a string of a given length containing the single character. In C++, we can define the string of a particular length by hard coding the values, but when we require to generate a string of different lengths and use the given character, we require to use the below approaches. Sample Examples Here are the sample ... Read More

159 Views
One of the most frequent tasks in web automation and testing is finding objects on a webpage. There are several ways to locate components with Selenium WebDriver, a potent tool for programmatically managing a web browser. The many locator strategies in Selenium Python will be covered in this post, with examples to help you along the way. Introduction The first step in using Selenium WebDriver to interact with a web element on a webpage, such as clicking a button or typing text into a textbox, is to locate the element. Eight alternative locators, or methods, are supported by selenium for ... Read More

119 Views
Strings are important data types in Python. Strings can be defined as the sequence of characters. Replacing the kth word in a String is an operation in which we want to replace the word which appears kth times in a String. In this article we will follow several methods to achieve it. We would look into the brute force method like using the loop statement, modules and libraries like re etc. Using Loop and split Method Loops are very common statements in most of the modern programming languages. We can either use the for or while loop to iterate over ... Read More

197 Views
Selenium is a powerful automation tool that enables programmatic control of a web browser. It is crucial for automating web applications for various functions, including testing. Finding items on a webpage is an essential Selenium feature. In this article, we'll look at utilising Python to find specific Selenium bits. Introduction to Selenium A well-known open-source web-based automation tool is Selenium. Because of its adaptability, developers can create scripts using a variety of computer languages, including Python, Java, C#, and others. Selenium makes it possible to automate actions that you would ordinarily perform with a browser, like clicking, typing, choosing, and ... Read More
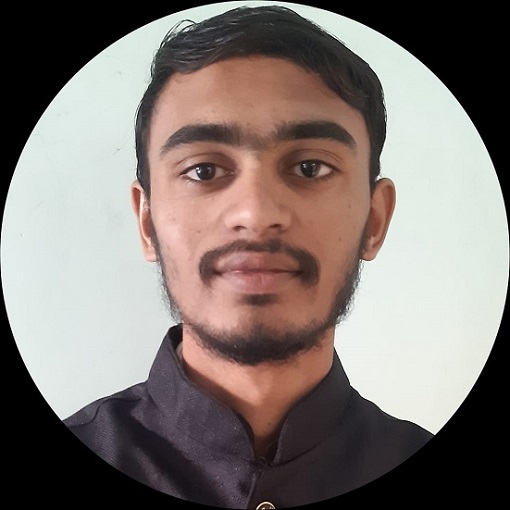
128 Views
Problem Statement We have given a string ‘str’, containing the alphabetical characters in uppercase or lowercase. We require to check if uppercase characters are used correctly in the string. Followings are the correct way to use uppercase characters in the string. If only the first character is in uppercase, the other characters are in lowercase. If all characters of a string are in lowercase. If all characters of the string are in uppercase. Sample examples Input "Hello" Output "valid" Explanation In "Hello", only the first character is in uppercase, and others are in lowercase, so it ... Read More