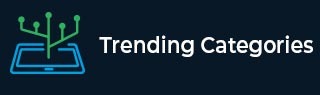
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 463 Articles for PowerShell

4K+ Views
Consider the below example, we have created Advanced function to get the specific process information like Process Name, Process ID (PID), Start Time, Responding status, etc.function Get-ProcessInformation{ [cmdletbinding()] param( [Parameter(Mandatory=$True)] [string[]]$name ) Begin{ Write-Verbose "Program started" } Process{ Write-Verbose "Extracting Process Inforamtion" Get-Process $name | Select Name, ID, StartTime, Responding | ft - AutoSize } End{ Write-Verbose "Function ends here" } }Above is the advanced function example. When we run the above command, it will give ... Read More

3K+ Views
AllowEmptyString() attribute works with the string variable and AllowEmptyCollection() work with the array of different data types (Collection).Consider the example below. Here, we are using a mandatory variable $name which is a string and $stringarray which is a string array.function print_String{ [cmdletbinding()] param( [parameter(Mandatory=$True)] [string]$name, ) Write-Output "Writing a single string" $name }If we get the output of the above variable it will generate an error below.PS C:\WINDOWS\system32> print_String cmdlet print_String at command pipeline position 1 Supply values for the following parameters: name: print_String : Cannot bind argument to ... Read More

343 Views
We have an example of PowerShell advanced function below and we will try to understand how mandatory parameter works.function math_Operation{ [cmdletbinding()] param([int]$val1, [int]$val2) Write-Host "Multiply : $($val1*$val2)" Write-Host "Addition : $($val1+$val2)" Write-Host "Subtraction : $($val1-$val2)" Write-Host "Divide : $($val1+$val2)" }When you execute the above example and don’t supply values then the script won’t ask you for the values, by default it will take the values and execute the program. See the execution below.PS C:\WINDOWS\system32> math_Operation Multiply : 0 Addition : 0 Subtraction : 0 Divide : 0Even if you have mentioned two variables ($val1, ... Read More

525 Views
Before starting the Advance PowerShell function, assuming we know about the PowerShell function. You can check the explanation on the PowerShell function below.https://www.tutorialspoint.com/explain-the-powershell-functionHere, we will take the math function example that calculates the different types of operations. We already have a code with the simple function as shown below.function math_Operation{ param([int]$val1, [int]$val2) Write-Host "Multiply : $($val1*$val2)" Write-Host "Addition : $($val1+$val2)" Write-Host "Subtraction : $($val1-$val2)" Write-Host "Divide : $($val1+$val2)" }The above example is of the simple function. When you run the above code and run the function from the terminal and you can notice you will ... Read More

17K+ Views
You can pass the parameters in the PowerShell function and to catch those parameters, you need to use the arguments. Generally, when you use variables outside the function, you really don’t need to pass the argument because the variable is itself a Public and can be accessible inside the function. But in some cases we need to pass the parameters to the function and below is the example explains how you can write the code for it.The single parameter passed in function, function writeName($str){ Write-Output "Hi! there .. $str" } $name = Read-Host "Enter Name" writeName($name)Here, we are passing ... Read More

214 Views
Generally, when the variable is declared as a Public variable or outside the function in the script (not in any other variable or condition), you don’t need to pass that value to function because the function retains the value of the variable when the variable is initialized before the function is called.Examplefunction PrintOut{ Write-Output "your Name is : $name" } $name = Read-Host "Enter your Name" PrintOutIn the above example, $name variable is declared outside the function called PrintOut. So as the variable can be read inside the function, you can directly use the variable by its name.OutputEnter your ... Read More

763 Views
Function in a PowerShell is to reduce the redundancy of the repeated codes this means codes which are repeating, bind them into a function and call the function whenever necessary, so you don’t need to write codes multiple times.ExampleLet assume, you want to perform arithmetic operations (multiply, sum, divide and subtraction) of two values 5 and 4 in one go, so you will write different operations for two values or you will assign values to the variable called $val1 and $val2 and perform various operations on them as shown below in the example.$val1 = 5 $val2 = 4 $val1 ... Read More

2K+ Views
Measure-Object in PowerShell is used to measure the property of the command. There are various measurement parameters are available. For example, Average, Count, sum, maximum, minimum and more.ExampleGet-Process | Measure-ObjectOutputPS C:\WINDOWS\system32> Get-Process | Measure-Object Count : 278 Average : Sum : Maximum : Minimum : Property :Here, in the above output, there are total 278 processes are running. If you want to check the maximum memory usage then you can use WorkingSet property with − Maximum Parameter.Get-Process | Measure-Object -Property WorkingSet -MaximumOutputPS C:\WINDOWS\system32> Get-Process | Measure-Object ... Read More

619 Views
As the name suggests, Group-Object is useful to group similar properties.ExampleGet-Service | Group-Object StatusOutputCount Name Group ----- ---- ----- 160 Stopped {AarSvc_8f3023, AdobeFlashPlayerUpdateSvc, AJR outer, ALG...} 130 Running {AdobeARMservice, Appinfo, AudioEndpointBuilder, Audiosrv...}The above output is grouped with the Status (“Stopped” and “Running”). There is a total of 160 services are in Stopped status and 130 are in Running status.Similarly, you can filter the group with the starttype property.Get-Service | Group-Object StartTypeOutputPS C:\WINDOWS\system32> Get-Service | Group- Object StartType Count Name ... Read More

10K+ Views
To sort the output in the PowerShell you need to use Sort-Object Pipeline cmdlet. In the below example, we will retrieve the output from the Get-Process command and we will sort the, according to memory and CPU usage.ExampleGet-Process | Sort-Object WorkingSet | Select -First 10OutputHandles NPM(K) PM(K) WS(K) CPU(s) Id SI ProcessName ------- ------ ----- ----- ------ -- -- ----------- 0 0 60 8 0 ... Read More