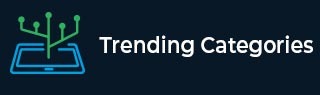
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

318 Views
In this problem statement our aim is to write an algorithm to remove duplicate items from an array with a function with the help of Javascript functionalities. Understanding the problem statement In the above problem statement we have to create a function by which we can accept an array as a parameter and give a new array to store the unique items from the given input array and without including the identical values. For example - suppose we have an array as [0, 0, 2, 2, 3, 3] so after removing the duplicate numbers we will have ... Read More

235 Views
In this article we will discuss algorithm and complexity for converting string with separator to array of objects with the help of Javascript functionalities. For doing this task we will use split and map functions of Javascript. Understanding the problem statement The problem statement says to write a function which can convert a given string with the given separator into an array of objects in Javascript. For example, if we have a string "name1, name2, name3" and a separator ", " then we have to convert these strings into an array of objects which looks like: [{value: ... Read More

2K+ Views
In the provided problem statement, our aim is to get all substrings of a string recursively with the help of Javascript. So here we will be creating a recursive function that can generate all possible substrings of a given input string. Understanding the problem statement The problem statement is asking us to create a recursive function which can generate all the possible substrings of a given input string with the help of Javascript. A substring is any continuous sequence of characters within the input string. For example: input strings “xy" so the possible substrings are "x", "y", and "xy". Algorithm ... Read More

5K+ Views
In the given problem we are required to retrieve the key and values from an object in an array with the help of Javascript. So here we will retrieve the keys and values from an object in an array with the help of two methods provided by the object class in Javascript. Understanding the Problem Statement The problem statement is to retrieve the keys and values from a given object in an array with the help of Javascript. So in Javascript the object is a collection of properties in which every property is a key and value pair. ... Read More

193 Views
In the given problem statement our task is to get the partial sum in an array of arrays with the help of Javascript functionalities. So we will calculate the sum of rows and give the result. Understanding the Problem The problem at hand is to compute the partial sum of an array of arrays. So first understand what an array of arrays is! Array of arrays means each item represents an array itself. For example see the below array of arrays: [ [11, 12, 13], [14, 15, 16], [17, 18, 19] ] The partial sum at ... Read More

170 Views
We are required to write a JavaScript function that takes in two dates in the 'YYYY-MM-DD' format as the first and second argument respectively. The function should then calculate and return the number of days between the two dates.For example −If the input dates are −const str1 = '2020-05-21'; const str2 = '2020-05-25';Then the output should be −const output = 4;Exampleconst str2 = '2020-05-25'; const daysBetweenDates = (str1, str2) => { const leapYears = (year, month) => { if (month (year * 365) + leapYears(year, month) + monthDays[month] + d; let p = days(...str1.split('-').map(Number)); ... Read More

310 Views
In this problem statement, our task is to write the function for getting the total subarrays with sum K with the help of Javascript. So for doing this task we will use basic functionality of Javascript. Understanding the problem statement The problem statement is to create a function which will take an array of integers and a target sum K. So after processing the calculation it will return the total number of subarrays in the array with a sum of K. A subarray can be defined as a continuous series of items in the array. For example suppose ... Read More

538 Views
Given a m * n matrix of distinct numbers, we have to return all lucky numbers in the 2-D array (matrix) in any order.A lucky number is an element of the matrix such that it is the minimum element in its row and maximum in its column.For example − If the input array is −const arr = [ [3, 7, 8], [9, 11, 13], [15, 16, 17] ];Then the output should be −const output = [15];because 15 is the only lucky number since it is the minimum in its row and the maximum in its column.ExampleThe code ... Read More

248 Views
Suppose, we have a sorted (increasing order) array of Numbers like this −const arr = [2, 5, 7, 8, 9];We are required to write a JavaScript function that takes in one such array. The function should construct a new subarray for each element of the input array.The sub-array should contain the difference (difference between that very element and the succeeding elements one by one) elements.Therefore, for the first array element, the differences are −5 - 2 = 3 7 - 2 = 5 8 - 2 = 6 9 - 2 = 7Therefore, the subarray for the first element should ... Read More

3K+ Views
In this problem statement, our task is to add a unique id for every object entry in a JSON object with the help of Javascript. So for doing this task we will use a loop and a variable to store the id for each object in the JSON object. Understanding the problem statement The problem statement is to write a function in Javascript by which we can add a unique id to every item in the given JSON object. For updating every JSON object by adding new id to every object we will use basic Javascript functionality. For example we ... Read More