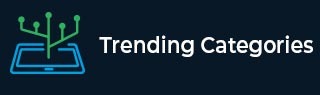
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

512 Views
Annotations are a tag (metadata) which provides info about a program. Annotations in Java Start with the symbol ‘@’. They are used by the compiler to detect errors. Software tools to generate code. They are used to show attributes of an element: e.g. @Deprecated, @Override. Annotation are used to describe the purpose of an element of the framework, e.g. @Entity, @TestCase, @WebService Annotations describe the behaviour of an element: @Statefull, @Transaction. Example Live Demo class Sample{ public void display(){ System.out.println(" "); } } public ... Read More

152 Views
In Aggregation relationship among classes by which a class (object) can be made up of any combination of objects of other classes. It allows objects to be placed directly within the body of other classes.A composition is also a type of aggregation where the relationship is restrictive i.e. If two objects are in composition, the composed object will not exist without the other.

1K+ Views
VM first looks for the main method (at least the latest versions) and then, starts executing the program including static block. Therefore, you cannot execute a static block without main method. Example public class Sample { static { System.out.println("Hello how are you"); } } Since the above program doesn’t have a main method, If you compile and execute it you will get an error message. C:\Sample>javac StaticBlockExample.java C:\Sample>java StaticBlockExample Error: Main method not found in class StaticBlockExample, please define the main method as: public static ... Read More

2K+ Views
The java.io.PrintStream.println() method prints an array of characters and then terminate the line. This method behaves as though it invokes print(char[]) and then println(). Using this method you can print the data on the console. import java.io.*; public class PrintStreamDemo { public static void main(String[] args) { char[] c = {'a', 'b', 'c'}; // create print stream object PrintStream ps = new PrintStream(System.out); // print an array and change line ps.println(c); ps.print("New Line"); // flush the stream ps.flush(); } } Output abc New Line

382 Views
You can declare an array just like a variable −int myArray[];You can create an array just like an object using the new keyword −myArray = new int[5];You can initialize the array by assigning values to all the elements one by one using the index −myArray [0] = 101; myArray [1] = 102;You can access the array element using the index values −System.out.println("The first element of the array is: " + myArray [0]); System.out.println("The first element of the array is: " + myArray [1]); Alternatively, you can create and initialize an array using the flower braces ({ }): Int [] myArray = {10, 20, 30, 40, 50}

291 Views
You can declare an array just like a variable −int myArray[];You can create an array just like an object using the new keyword −myArray = new int[5];You can initialize the array by assigning values to all the elements one by one using the index −myArray [0] = 101; myArray [1] = 102;You can access the array element using the index values −System.out.println("The first element of the array is: " + myArray [0]); System.out.println("The first element of the array is: " + myArray [1]); Alternatively, you can create and initialize an array using the flower braces ({ }): Int [] myArray = {10, 20, 30, 40, 50}

1K+ Views
While declaring the constructors you should keep the following points in mind.A constructor does not have a return type.The name of the constructor is same as the name of the class. A class can have more than one constructor.Examplepublic class Sample { int num; public Sample() { num = 30; } public Sample(int value) { num = value; } }

171 Views
The this is a keyword in Java which is used as a reference to the object of the current class, within an instance method or a constructor. Using this you can refer the members of a class such as constructors, variables, and methods. Example Live Demo public class This_Example { // Instance variable num int num = 10; This_Example() { System.out.println("This is an example program on keyword this"); } This_Example(int num) { ... Read More

2K+ Views
A Java file contains only one public class with a particular name. If you create another class with same name it will be a duplicate class. Still if you try to create such class then the compiler will generate a compile time error. Example public class Example { } public class Example{ public void sample(){ System.out.println("sample method of the Example class"); } public void demo(){ System.out.println("demo method of the Example class"); } ... Read More