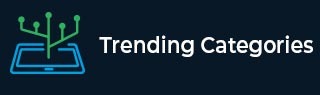
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

528 Views
We are required to write a JavaScript function that takes in an array of Numbers. The function should construct a new array based on the original array. Each corresponding element of the new array should be the product of all the elements of the original array including that element.For example −If the input array is −const arr = [1, 2, 3, 4, 5];Then the output array should be −const output = [120, 60, 40, 30, 24];We have to achieve this in linear time and constant space (obviously excluding the space used up in constructing the new array).ExampleFollowing is the code ... Read More

88 Views
In this problem statement we have to find the largest difference between elements with a twist with the help of Javascript functionalities. So we will use basic Javascript to get the desired result. Understanding the problem The problem at hand is to find the largest difference between two items in an array. So we will add a twist to the problem. So we will not simply find the difference between two items but we will find the largest difference between an item and any smaller item that has appeared before in the array. And we will not rearrange ... Read More

380 Views
In the given problem statement our task is to find the greatest product of three numbers with the help of Javascript functionalities. So we will use sorting technique first to get the last three highest items of the array and then calculate the product of those three elements to get the desired result. Understanding the Problem The problem at hand is to find the greatest product of three items or numbers present in the given array in Javascript. So we will have an array of integers and we will identify three numbers whose product is the largest among ... Read More

173 Views
In this given statement our task is to determine whether we can form string2 by removing some characters from the string1 without changing the order of the characters of any string with the help of Javascript functionalities. Understanding the problem The problem at hand is to check that the second string can be formed using the first string without changing the order of both the strings. For example suppose we have two strings as: s1 = "apple" s2 = "ale" Output - true So the output for the above strings should be true because the ... Read More

129 Views
In the given problem we are required to find out the numbers from a given string with the help of Javascript functionalities. So we will use regular expressions and some predefined functions of Javascript. Understanding the Problem The problem at hand is to find the numbers from the given string in Javascript. This task can be useful sometimes. To accomplish this task we will use regular expressions and also some string manipulation functions of Javascript. So in simple terms we will be given a string in this string there will be some integer or other type of number ... Read More

619 Views
In the given problem statement we have to find all duplicate numbers in an array with multiple duplicates with the help of Javascript functionalities. So we will use basic Javascript tot solve this problem. Understanding the Problem The problem at hand is to find the duplicate numbers in an array with the help of JavaScript. So we will basically extract all the numbers which are appearing more than once in the given array. And in response we will get the array of repeated numbers. For example, suppose we have an array as [1, 1, 4, 8, 2, 2, ... Read More

682 Views
In the given problem statement we have to calculate the cumulative sum at each index with the help of Javascript functionalities. So we will use basic Javascript syntax and functions to solve this problem. What is Cumulative sum ? The cumulative sum is also known as running sum or prefix sum. So this sum is the calculation of the sum of a series of numbers up to a given index or position. In this process the numbers iteratively add each number in the series to the sum of the previous items. So the resultant new series in ... Read More

4K+ Views
In the given problem statement we have to find the largest and smallest number in an unsorted array of integers with the help of Javascript functionalities. So we will use an array of integers and find the smallest and largest number from the array. Understanding the Problem The problem we have is to find the largest and smallest values in an unsorted array of integers. So in this task we will see a solution in Javascript. With the help of it we can determine the largest and smallest numbers in an array without sorting the array. For example ... Read More

445 Views
In the given problem statement we are required to factorize the given number with the help of Javascript functionalities. So we will use loops and basic mathematics to factorize the given number. Understanding the Problem The problem at hand is to factorize the given number with the help of Javascript. So the factorization means we will have to find all the prime factors of a number. The prime factors are the prime numbers which can divide the given number without leaving a remainder. So with the help of finding the prime factors we will be able to ... Read More

182 Views
In this article we will see how to find a pair of items in an array which has sum equals to a specific target number. So we will use JavaScript to implement the algorithm. Understanding the Problem The problem at hand is to find the pair of numbers in the array whose sum is equal to the given target value. And the target value should also be there in the array. Or we can say that we have to identify pairs (a, b) where a + b = c and c is also present in the array. ... Read More