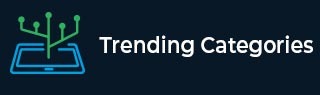
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

14K+ Views
You can access the private methods of a class using java reflection package.Step1 − Instantiate the Method class of the java.lang.reflect package by passing the method name of the method which is declared private.Step2 − Set the method accessible by passing value true to the setAccessible() method.Step3 − Finally, invoke the method using the invoke() method.Exampleimport java.lang.reflect.Method; public class DemoTest { private void sampleMethod() { System.out.println("hello"); } } public class SampleTest { public static void main(String args[]) throws Exception { Class c = Class.forName("DemoTest"); Object obj ... Read More

225 Views
Wan import statement in Java is used to − Import user defined classes/Interfaces Whenever you need to access a class which is not in the current package of the program you need to import that particular class using the import statement. Example In the following example we are using the Math class to find the square root of a number therefore, first of all w should import this class using the import statement. Live Demo import java.lang.Math.*; public class Sample{ public static void main(String args[]){ System.out.println(Math.sqrt(169)); ... Read More

497 Views
As import statement allows to use a class without its package qualification, static import allows to access the static members of a class without class qualifications. For Example, to access the static methods you need to call the using class name − Math.sqrt(169); But, using static import you can access the static methods directly. Example Live Demo import static java.lang.Math.*; public class Sample{ public static void main(String args[]){ System.out.println(sqrt(169)); } } Output 13.0

3K+ Views
No, java.lang package is a default package in Java therefore, there is no need to import it explicitly. i.e. without importing you can access the classes of this package. If you observe the following example here we haven’t imported the lang package explicitly but, still we are able to calculate the square root of a number using the sqrt() method of the java.lang.Math class. Example Live Demo public class LangTest { public static void main(String args[]){ int num = 100; double result = ... Read More

656 Views
As per dictionary, abstraction is the quality of dealing with ideas rather than events. For example, when you consider the case of e-mail, complex details such as what happens as soon as you send an e-mail, the protocol your e-mail server uses are hidden from the user. Therefore, to send an e-mail you just need to type the content, mention the address of the receiver, and click send. Abstraction is a process of hiding the implementation details from the user, only the functionality will be provided to the user. In other words, the user will have the information on what ... Read More

181 Views
Passing Parameters by Value means calling a method with a parameter. Through this, the argument value is passed to the parameter. Example public class SwappingExample { public static void swapFunction(int a, int b) { int c = a+b; System.out.println("Sum of the given numbers is ::"+c); } public static void main(String[] args) { int a = 30; int b = 45; swapFunction(a, b); } } Output Sum of the given numbers is ::75

161 Views
Recursion is where which a method class itself.Examplepublic class RecursionExample { private static long factorial(int n) { if (n == 1) return 1; else return n * factorial(n-1); } public static void main(String args[]) { RecursionExample obj = new RecursionExample(); long result = obj.factorial(5); System.out.println(result); } }

1K+ Views
Typecasting is converting one data type to another.Up-casting − Converting a subclass type to a superclass type is known as up casting.Exampleclass Super { void Sample() { System.out.println("method of super class"); } } public class Sub extends Super { void Sample() { System.out.println("method of sub class"); } public static void main(String args[]) { Super obj =(Super) new Sub(); obj.Sample(); } }Down-casting − Converting a superclass type to a subclass type is known as downcasting.Exampleclass Super { void Sample() { ... Read More