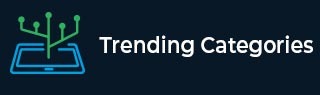
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

173 Views
Apache Commons provides a library named org.apache.commons.lang3 and, following is the maven dependency to add the library to your project. org.apache.commons commons-lang3 3.0 This package provides a class named ArrayUtils. Using the toPrimitive() method of this class you can convert An object array to an array of primitive types:Exampleimport java.util.Arrays; import org.apache.commons.lang3.ArrayUtils; public class ArraysToPrimitives { public static void main(String args[]) { Integer[] myArray = {234, 76, 890, 27, 10, 63}; int[] primitiveArray = ArrayUtils.toPrimitive(myArray); System.out.println(Arrays.toString(primitiveArray)); } }Output[234, 76, 890, 27, 10, 63]

9K+ Views
A 2d array is an array of one dimensional arrays to read the contents of a file to a 2d array –Instantiate Scanner or other relevant class to read data from a file. Create an array to store the contents.To copy contents, you need two loops one nested within the other. the outer loop is to traverse through the array of one dimensional arrays and, the inner loop is to traverse through the elements of a particular one dimensional array.Create an outer loop starting from 0 up to the length of the array. Within this loop read each line trim and ... Read More

13K+ Views
Using the copyOfRange() method you can copy an array within a range. This method accepts three parameters, an array that you want to copy, start and end indexes of the range.You split an array using this method by copying the array ranging from 0 to length/2 to one array and length/2 to length to other.Exampleimport java.util.Arrays; import java.util.Scanner; public class SplittingAnArray { public static void main(String args[]) { Scanner s =new Scanner(System.in); System.out.println("Enter the required size of the array ::"); int size = s.nextInt(); int [] myArray ... Read More

747 Views
The interface Set does not allow duplicate elements, therefore, create a set object and try to add each element to it using the add() method in case of repetition of elements this method returns false − If you try to add all the elements of the array to a Set, it accepts only unique elements − Example import java.util.Arrays; import java.util.HashSet; import java.util.Scanner; import java.util.Set; public class CountingUniqueElements { public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.println("Enter the size ... Read More

3K+ Views
You can find numbers in an array that are greater than, less than, or equal to a value as:ExampleLive Demopublic class GreaterOrLess { public static void main(String args[]) { int value = 65; int[] myArray = {41, 52, 63, 74, 85, 96 }; System.out.println("Elements of the array that are equal to the given value are::"); for(int i = 0; i

3K+ Views
Create an array to which you want to store the existing array with the same length. A 2d array is an array of one dimensional arrays therefore, to copy (or, to perform any operation on) the elements of the 2d array you need two loops one nested within the other. Where, the outer loop is to traverse through the array of one dimensional arrays and, the inner loop is to traverse through the elements of a particular one dimensional array.Examplepublic class Copying2DArray { public static void main(String args[]) { int[][] myArray = {{41, 52, 63}, {74, ... Read More

3K+ Views
Yes, we can overload the main method in Java, but When we execute the class JVM starts execution with public static void main(String[] args) method. Example Live Demo public class Sample{ public static void main(){ System.out.println("This is the overloaded main method"); } public static void main(String args[]){ Sample obj = new Sample(); obj.main(); } } Output This is the overloaded main method

2K+ Views
Overloading is the mechanism of binding the method call with the method body dynamically based on the parameters passed to the method call. If you observe the following example, it contains two methods with same name, different parameters and if you call the method by passing two integer values the first method will be executed and, if you call by passing 3 integer values then the second method will be executed.It is not possible to decide to execute which method based on the return type, therefore, overloading is not possible just by changing the return type of the method. Example ... Read More

2K+ Views
When super class and sub class contains same method including parameters and if they are static. The method in the super class will be hidden by the one that is in the sub class. This mechanism is known as method hiding. Example Live Demo class Demo{ public static void demoMethod() { System.out.println("method of super class"); } } public class Sample extends Demo { public static void demoMethod() { System.out.println("method of sub class"); } public static void main(String args[] ) { Sample.demoMethod(); } } Output method of sub class

1K+ Views
When super class and the sub class contains same instance methods including parameters, when called, the super class method is overridden by the method of the sub class. WIn this example super class and sub class have methods with same signature (method name and parameters) and when we try to invoke this method from the sub class the sub class method overrides the method in super class and gets executed. Example Live Demo class Super{ public void sample(){ System.out.println("Method of the Super class"); } } public ... Read More