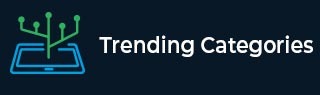
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

3K+ Views
A linked list is a sequence of data structures, which are connected together via links.To create an array of linked lists, create required linked lists and, create an array of objects with them.ExampleLive Demoimport java.util.LinkedList; public class ArrayOfLinkedList { public static void main(String args[]) { LinkedList list1 = new LinkedList(); list1.add("JavaFX"); list1.add("Hbase"); LinkedList list2 = new LinkedList(); list2.add("OpenCV"); list2.add("Mahout"); LinkedList list3 = new LinkedList(); list3.add("WebGL"); list3.add("CoffeeScript"); Object[] obj = {list1, list2, list3}; for (int i=0; i

2K+ Views
In the loop check, the result of i%2 operation on each element if 0 the element is even else the element is odd.ExampleLive Demopublic class OddNumbersInAnArray { public static void main(String args[]) { int[] myArray = {23, 93, 56, 92, 39}; System.out.println("Even numbers in the given array are:: "); for (int i=0; i

12K+ Views
An array is a data structure/container/object that stores a fixed-size sequential collection of elements of the same type. The size/length of the array is determined at the time of creation. The position of the elements in the array is called as index or subscript. The first element of the array is stored at the index 0 and, the second element is at the index 1 and so on. Each element in an array is accessed using an expression which contains the name of the array followed by the index of the required element in square brackets. You can access an ... Read More

13K+ Views
To convert an object to byte arrayMake the required object serializable by implementing the Serializable interface.Create a ByteArrayOutputStream object.Create an ObjectOutputStream object by passing the ByteArrayOutputStream object created in the previous step.Write the contents of the object to the output stream using the writeObject() method of the ObjectOutputStream class.Flush the contents to the stream using the flush() method.Finally, convert the contents of the ByteArrayOutputStream to a byte array using the toByteArray() method.Exampleimport java.io.ByteArrayOutputStream; import java.io.ObjectOutputStream; import java.io.Serializable; class Sample implements Serializable { public void display() { System.out.println("This is a sample class"); } } public ... Read More

12K+ Views
To move an element from one position to other (swap) you need to –Create a temp variable and assign the value of the original position to it.Now, assign the value in the new position to original position.Finally, assign the value in the temp to the new position.ExampleLive Demoimport java.util.Arrays; public class ChangingPositions { public static void main(String args[]) { int originalPosition = 1; int newPosition = 4; int [] myArray = {23, 93, 56, 92, 39}; int temp = myArray[originalPosition]; myArray[originalPosition] = myArray[newPosition]; myArray[newPosition] = temp; System.out.println(Arrays.toString(myArray)); } }Output[23, 39, 56, 92, 93]

682 Views
The Arrays class of the java.util package provides toString() methods for all primitive data types and object. These methods accept an array and return its string representation.Therefore, to convert an array to string pass the required array to this method.ExampleLive Demoimport java.util.Arrays; public class ArrayToString { public static void main(String args[]) throws Exception { int[] myArray = {23, 93, 56, 92, 39}; String str = Arrays.toString(myArray); System.out.println(str); } }Output[23, 93, 56, 92, 39]

18K+ Views
Java provides ImageIO class for reading and writing an image. To convert an image to a byte array –Read the image using the read() method of the ImageIO class.Create a ByteArrayOutputStream object.Write the image to the ByteArrayOutputStream object created above using the write() method of the ImageIO class.Finally convert the contents of the ByteArrayOutputStream to a byte array using the toByteArray() method.Exampleimport java.io.ByteArrayOutputStream; import java.awt.image.BufferedImage; import java.io.File; import javax.imageio.ImageIO; public class ImageToByteArray { public static void main(String args[]) throws Exception{ BufferedImage bImage = ImageIO.read(new File("sample.jpg")); ... Read More

2K+ Views
To move an element from one position to other (swap) you need to –Create a temp variable and assign the value of the original position to it.Now, assign the value in the new position to original position.Finally, assign the value in the temp to the new position.ExampleLive Demoimport java.util.Arrays; public class ChangingPositions { public static void main(String args[]) { int originalPosition = 1; int newPosition = 1; int [] myArray = {23, 93, 56, 92, 39}; int temp = myArray[originalPosition]; myArray[originalPosition] = myArray[newPosition]; myArray[newPosition] = temp; System.out.println(Arrays.toString(myArray)); } }Output[23, 39, 56, 92, 93]

18K+ Views
Java provides ImageIO class for reading and writing an image. To convert a byte array to an image.Create a ByteArrayInputStream object by passing the byte array (that is to be converted) to its constructor.Read the image using the read() method of the ImageIO class (by passing the ByteArrayInputStream objects to it as a parameter).Finally, Write the image to using the write() method of the ImageIo class.Exampleimport java.io.ByteArrayOutputStream; import java.awt.image.BufferedImage; import java.io.File; import javax.imageio.ImageIO; public class ByteArrayToImage { public static void main(String args[]) throws Exception { BufferedImage bImage = ImageIO.read(new File("sample.jpg")); ... Read More

7K+ Views
You can read data from a PDF file using the read() method of the FileInputStream class this method requires a byte array as a parameter.Exampleimport java.io.File; import java.io.FileInputStream; import java.io.ByteArrayOutputStream; public class PdfToByteArray { public static void main(String args[]) throws Exception { File file = new File("sample.pdf"); FileInputStream fis = new FileInputStream(file); byte [] data = new byte[(int)file.length()]; fis.read(data); ByteArrayOutputStream bos = new ByteArrayOutputStream(); data = bos.toByteArray(); } }Sample.pdf