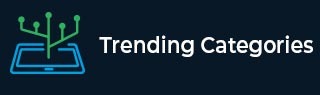
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

589 Views
The public modifier has the widest scope. When a class or its members declared public they are accessible from everywhere. A default class or its members are available to any other class in the same package. However, if the public class we are trying to access is in a different package, then the public class still needs to be imported. Because of class inheritance, all public methods and variables of a class are inherited by its subclasses. Example The following function uses public access control − public static void main(String[] arguments) { // ... } ... Read More

629 Views
Local variables are declared in methods, constructors, or blocks. Local variables are created when the method, constructor or block is entered and the variable will be destroyed once it exits the method, constructor, or block. A local variable is the one which is declared within a method. The scope of this variable is within the method. Example public abstract class Sample { public static void main(String args[]){ int data = 4044; System.out.println(data); } } Output 4044

2K+ Views
When we haven’t initialized the instance variables compiler initializes them with default values.For boolean type, the default value is false, for float and double types default values are 0.0 and for remaining primitive types default value is 0.ExampleLive Demopublic class Sample { int varInt; float varFloat; boolean varBool; long varLong; byte varByte; short varShort; double varDouble; public static void main(String args[]){ Sample obj = new Sample(); System.out.println("Default int value ::"+obj.varInt); System.out.println("Default float value ::"+obj.varFloat); System.out.println("Default boolean value ::"+obj.varBool); ... Read More

942 Views
No, local variables do not have default values. Once we create a local variable we must initialize it before using it. Since the local variables in Java are stored in stack in JVM there is a chance of getting the previous value as default value. Therefore, In Java default values for local variables are not allowed. Example public class Sample { public static void main(String args[] ){ int data; System.out.println(data); } } Error C:\Sample>javac Sample.java Sample.java:4: error: variable data might not have been initialized System.out.println(data); ^ 1 error

33K+ Views
A variable provides us with named storage that our programs can manipulate. Java provides three types of variables. Class variables − Class variables also known as static variables are declared with the static keyword in a class, but outside a method, constructor or a block. There would only be one copy of each class variable per class, regardless of how many objects are created from it. Instance variables − Instance variables are declared in a class, but outside a method. When space is allocated for an object in the heap, a slot for each instance variable value is created. ... Read More

34K+ Views
Following are the notable differences between Class (static) and instance variables. Instance variables Static (class) variables Instance variables are declared in a class, but outside a method, constructor or any block. Class variables also known as static variables are declared with the static keyword in a class, but outside a method, constructor or a block. Instance variables are created when an object is created with the use of the keyword 'new' and destroyed when the object is destroyed. Static variables are created when the program starts and destroyed when the program stops. ... Read More

938 Views
String is not a primitive data type. Java.lang package provides the String class therefore, it is an object type. You can create a string variable directly like any other variables as −String s = "myString";(or)By instantiating the string class using the new keyword as −String s = new String("myString");Example Live Demoimport java.util.Scanner; public class StringExample { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("Enter a sting value:"); String str = sc.nextLine(); System.out.println(str.getClass()); } }OutputEnter a sting value: hello class java.lang.String

1K+ Views
Boolean literals represent only two values true or false. And in Java the value of 1 is assumed as true and the value of 0 is assumed as false. Example Live Demo public class Test{ public static void main(String args[]) throws Exception{ boolean bool1 = true; boolean bool2 = false; boolean bool = (25==(100/4)); System.out.println(bool1); System.out.println(bool2); System.out.println(bool); } } Output true false true

668 Views
Character literals represents alphabets (both cases), numbers (0 to 9), special characters (@, ?, & etc.) and escape sequences like , \b etc. Whereas, the String literal represents objects of String class. Example Live Demo public class LiteralsExample { public static void main(String args[]){ char ch = 'H'; String str = "Hello"; System.out.println("Value of character: "+ch); System.out.println("Value of string: "+str); } } Output Value of character: H Value of string: Hello