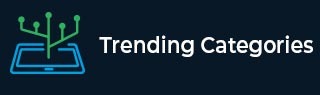
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

282 Views
An abstract keyword is used to declare methods abstract methods and abstract classes. Once a method is declared abstract we should not specify body for those. Example public abstract class Sample{ public abstract demo(){ } } Error Sample.java:2: error: invalid method declaration; return type required public abstract demo(){ ^ 1 error And once a class is declared abstract it cannot be instantiated. Example public abstract class Sample{ public abstract void demo(); public static void main(String args[]){ new Sample(); } } Error C:\Sample>javac Sample.java Sample.java:4: error: Sample is abstract; cannot be instantiated new Sample(); ^ 1 error

994 Views
The synchronized keyword used to indicate that a method can be accessed by only one thread at a time. The synchronized modifier can be applied with any of the four access level modifiers. Example Live Demo public class TestThread { public static Object Lock1 = new Object(); public static Object Lock2 = new Object(); public static void main(String args[]) { ThreadDemo1 T1 = new ThreadDemo1(); ThreadDemo2 T2 = new ThreadDemo2(); ... Read More

446 Views
We can declare an array as a local variable or method parameter but, an array cannot be static. Example public class Test{ public void sample(){ static int[] myArray = {20, 30}; System.out.println(); } public static void main(String args[]){ Test t = new Test(); t.sample(); } } Error C:\Sample>javac Test.java Test.java:3: error: illegal start of expression static int[] myArray = {20, 30}; ^ 1 error

283 Views
The abstract keyword is used to declare methods abstract methods and abstract classes. Once a method is declared abstract we should not specify body for those. And once a class is declared abstract it cannot be instantiated. Example Live Demo abstract class Employee { private String name; private String address; private int number; public Employee(String name, String address, int number) { System.out.println("Constructing an Employee"); this.name = name; ... Read More

3K+ Views
You can pass final variables as the parameters to methods in Java. A final variable can be explicitly initialized only once. A reference variable declared final can never be reassigned to refer to a different object. However, the data within the object can be changed. So, the state of the object can be changed but not the reference. With variables, the final modifier often is used with static to make the constant a class variable. Example Live Demo public class Test{ public void sample(final int data){ System.out.println(data); ... Read More

829 Views
The scope of the private modifier lies with in the class. Members that are declared private cannot be accessed outside the class. Private access modifier is the most restrictive access level. Class and interfaces cannot be private. Variables that are declared private can be accessed outside the class, if public getter methods are present in the class. Using the private modifier is the main way that an object encapsulates itself and hides data from the outside world. Example The following class uses private access control public class Logger { private String format; ... Read More

990 Views
A final variable can be explicitly initialized only once. A reference variable declared final can never be reassigned to refer to a different object. However, the data within the object can be changed. So, the state of the object can be changed but not the reference. With variables, the final modifier often is used with static to make the constant a class variable. Therefore, once we declare a final variable it is mandatory to initialize the final variable at the time of declaration or using constructor. If not, a compile time error may occur saying “The blank final field num ... Read More

980 Views
Default access modifier means we do not explicitly declare an access modifier for a class, field, method, etc. The scope of the default access modifier lies within the package. When a class or its members associated with default access modifier then. Example Variables and methods can be declared without any modifiers, as in the following examples: String version = "1.5.1"; boolean processOrder() { return true; }

2K+ Views
When a variable, method or constructor that are declared protected in a superclass can be accessed only by the subclasses in other package or any class within the package of the protected members' class. The protected access modifier cannot be applied to class and interfaces. Methods, fields can be declared protected, however methods and fields in a interface cannot be declared protected. Protected access gives the subclass a chance to use the helper method or variable, while preventing a nonrelated class from trying to use it. Example The following parent class uses protected access control, to allow its child class ... Read More

1K+ Views
Class variables also known as static variables are declared with the static keyword There would only be one copy of each class variable per class, regardless of how many objects are created from it. You can access a class variable without instantiation using the class name as className.variableName. Example Live Demo public class Test{ static int num = 92; public static void main(String args[]) throws Exception { System.out.println(Test.num); } } Output 92