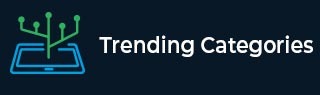
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

295 Views
The throw keyword is used to raise an exception explicitly.Examplepublic class Test { public static void main(String[] args) { throw new NullPointerException(); } }Exception in thread "main" java.lang.NullPointerException at a6.dateAndTime.Test.main(Test.java:5)The throws keywords in Java used to postpone the handling of a checked exception.public class Test { public static void main(String[] args)throws NullPointerException { throw new NullPointerException(); } }

273 Views
A catch statement involves declaring the type of exception you are trying to catch. If an exception occurs in the try block, the catch block (or blocks) that follows the try is checked. If the type of exception that occurred is listed in a catch block, the exception is passed to the catch block much as an argument is passed into a method parameter.Exampleimport java.io.File; import java.io.FileInputStream; public class Test { public static void main(String args[]) { System.out.println("Hello"); try { File file = new File("my_file"); ... Read More

407 Views
A try/catch block is placed around the code that might generate an exception. Code within a try/catch block is referred to as protected code, and the syntax for using try/catch looks like the following –Syntaxtry { // Protected code } catch (ExceptionName e1) { // Catch block }The code which is prone to exceptions is placed in the try block. When an exception raised inside a try block, instead of terminating the program JVM stores the exception details in the exception stack and proceeds to the catch block.

144 Views
This Exception happens once you associated convert a string variable in an incorrect format to a whole number (numeric format) that's not compatible with one another. Example public class Test { public static void main(String[] args) { int data = Integer.parseInt("hello"); } } Output Exception in thread "main" java.lang.NumberFormatException: For input string: "hello" at java.lang.NumberFormatException.forInputString(Unknown Source) at java.lang.Integer.parseInt(Unknown Source) at java.lang.Integer.parseInt(Unknown Source) at a6.dateAndTime.Test.main(Test.java:5)

710 Views
A checked exception is an exception that occurs at the compile time, these are also called as compile time exceptions. These exceptions cannot simply be ignored at the time of compilation; the programmer should take care of (handle) these exceptions.ExampleIf you use FileReader class in your program to read data from a file, if the file specified in its constructor doesn't exist, then a FileNotFoundException occurs, and the compiler prompts the programmer to handle the exception.import java.io.File; import java.io.FileReader; public class FilenotFound_Demo { public static void main(String args[]) { File file = new File("E://file.txt"); ... Read More

2K+ Views
An unchecked exception is the one which occurs at the time of execution. These are also called as Runtime Exceptions. These include programming bugs, such as logic errors or improper use of an API. Runtime exceptions are ignored at the time of compilation. If you have declared an array of size 5 in your program, and trying to call the 6th element of the array then an ArrayIndexOutOfBoundsExceptionexception occurs.ExampleLive Demopublic class Unchecked_Demo { public static void main(String args[]) { int num[] = {1, 2, 3, 4}; System.out.println(num[5]); } }OutputException in thread "main" java.lang.ArrayIndexOutOfBoundsException: ... Read More

749 Views
A checked exception is an exception that occurs at the time of compilation, these are also called as compile time exceptions. These exceptions cannot simply be ignored at the time of compilation; the programmer should take care of (handle) these exceptions. if you use FileReader class in your program to read data from a file, if the file specified in its constructor doesn't exist, then a FileNotFoundException occurs, and the compiler prompts the programmer to handle the exception. Example import java.io.File; import java.io.FileReader; public class FilenotFound_Demo { public static void main(String args[]) { ... Read More

474 Views
Not necessarily catch, a try must be followed by either catch or finally block. Example import java.io.File; public class Test{ public static void main(String args[]){ System.out.println("Hello"); try{ File file = new File("data"); } } } Output C:\Sample>Javac Test.java Test.java:5: error: 'try' without 'catch', 'finally' or resource declarations try{ ^ 1 error

291 Views
Compile time errors are syntactical errors in the code which hinders it from being compiled. Example public class Test{ public static void main(String args[]){ System.out.println("Hello") } } Output C:\Sample>Javac Test.java Test.java:3: error: ';' expected System.out.println("Hello") An exception (or exceptional event) is a problem that arises during the execution of a program. When an Exception occurs the normal flow of the program is disrupted and the program/Application terminates abnormally, which is not recommended, therefore, these exceptions are to be handled. Example ... Read More