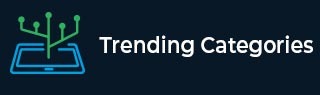
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

182 Views
Block SearchJust like Binary Search, Block Search is also a searching algorithm for sorted arrays. The basic idea is to check fewer elements (than linear search) by jumping ahead by fixed steps or skipping some elements in place of searching all elements.For exampleSuppose we have an array arr of length n and block (to be jumped) of size m. Then we search at the indexes arr[0], arr[m], arr[2 * m], ..., arr[k * m] and so on.Once we find the interval arr[k * m] < x < arr[(k+1) * m], we perform a linear search operation from the index k ... Read More

1K+ Views
Hamming DistanceThe Hamming distance between two strings of equal length is the number of positions at which the corresponding symbols are different.For example, consider the following strings −const str1 = 'delhi'; const str2 = 'delph';The Hamming Distance of these strings is 2 because the fourth and the fifth characters of the strings are different. And obviously in order to calculate the Hamming Distance we need to have two strings of equal lengths.Therefore, we are required to write a JavaScript function that takes in two strings, let’s say str1 and str2, and returns their hamming distance.ExampleFollowing is the code −const str1 ... Read More

186 Views
Cartesian Product Inset theory a Cartesian product is a mathematical operation that returns a set (or product set or simply product) from multiple sets.That is, for sets A and B, the Cartesian product A × B is the set of all ordered pairs (a, b) where a ∈ A and b ∈ B.We are required to write a JavaScript function that takes in two arrays let us call them arr1 and arr2, they both represent two distinct sets.The function should construct a 2-D array that contains the cartesian product of those two sets and finally return that array.ExampleFollowing is the code ... Read More

418 Views
The least common multiple, (LCM) of two integers a and b, is the smallest positive integer that is divisible by both a and b.For example −LCM of 4 and 6 is 12 because 12 is the smallest number that is exactly divisible by both 4 and 6.We are required to write a JavaScript function that takes in two numbers, computes and returns the LCM of those numbers.ExampleFollowing is the code −const num1 = 4; const num2 = 6; const findLCM = (num1, num2) => { let hcf; for (let i = 1; i

416 Views
Suppose we are given a set of candidate numbers (without duplicates) and a target number (target).We are required to write a function that finds all unique combinations in candidates where the candidate numbers sum to the target.The same repeated number may be chosen from candidates an unlimited number of times.Note −All numbers (including target) will be positive integers.The solution set must not contain duplicate combinations.For example −If the inputs are −candidates = [2, 3, 6, 7], target = 7, The solution to this can be −[ [7], [2, 2, 3] ];Since the problem is to get all the ... Read More

188 Views
Suppose we are given an input string str and a pattern p, we are required to implement regular expression matching with support for. and *.The functions of these symbols should be −. --> Matches any single character.* --> Matches zero or more of the preceding elements.The matching should cover the entire input string (not partial).Notestr could be empty and contains only lowercase letters a-z.p could be empty and contains only lowercase letters a-z, and characters like. or *.For example −If the input is −const str = 'aa'; const p = 'a';Then the output should be false because a does not ... Read More

288 Views
We are required to write a JavaScript function that takes in a number and determines whether or not it is a power of two.For example −f(23) = false f(16) = true f(1) = true f(1024) = trueApproach −Powers of two in binary form always have just one bit. Like this −1: 0001 2: 0010 4: 0100 8: 1000Therefore, after checking that the number is greater than zero, we can use a bitwise hack to test that one and only one bit is set. The same is shown below −num & (num - 1)ExampleFollowing is the code −const num1 = 256; ... Read More

1K+ Views
In mathematics, Euclid's algorithm, is a method for computing the greatest common divisor (GCD) of two numbers, the largest number that divides both of them without leaving a remainder.The Euclidean algorithm is based on the principle that the greatest common divisor of two numbers does not change if the larger number is replaced by its difference with the smaller number.For example, 21 is the GCD of 252 and 105 (as 252 = 21 × 12 and 105 = 21 × 5), and the same number 21 is also the GCD of 105 and 252 − 105 = 147.Since this replacement ... Read More

192 Views
A prime number (or a prime) is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. All other natural numbers greater than 1 are called composite numbers. A primality test is an algorithm for determining whether an input number is prime.We are required to write a JavaScript function that takes in a number and checks whether it is a prime or not.ExampleFollowing is the code −const findPrime = (num = 2) => { if (num % 1 !== 0) { return false; } if (num

126 Views
We will be writing two JavaScript functions, the job of both the functions will be to take in a number and return its factorial.The first function should make use of a for loop or while loop to compute the factorial. Whereas the second function should compute the factorial using a recursive approach.Lastly, we should compare the times taken by these functions over a large number of iterations.ExampleFollowing is the code −const factorial = (num = 1) => { let result = 1; for (let i = 2; i { if(num > 1){ return ... Read More