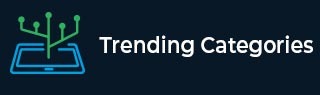
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

72 Views
The sort(Object[]) method of the java.util.Arrays class sorts the specified array of Objects into ascending order according to the natural ordering of its elements.Exampleimport java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { Object ob[] = {27, 11, 44}; for (Object number : ob) { System.out.println("Number = " + number); } Arrays.sort(ob); System.out.println("The sorted Object array is:"); for (Object number : ob) { System.out.println("Number = " + number); } } }OutputNumber = 27 Number = 11 Number = 44 The sorted Object array is: Number = 11 Number = 27 Number = 44

159 Views
The instanceof operator is used only for object reference variables. The operator checks whether the object is of a particular type (class type or interface type).Examplepublic class Test { public static void main(String args[]) { String name = "James"; boolean result = name instanceof String; System.out.println(result); } }Outputtrue

64 Views
The sort(int[]) method of the java.util.Arrays class sorts the specified array of integer values into ascending numerical order.Exampleimport java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { int iArr[] = {2, 1, 9, 6, 4}; for (int number : iArr) { System.out.println("Number = " + number); } Arrays.sort(iArr); System.out.println("The sorted int array is:"); for (int number : iArr) { System.out.println("Number = " + number); } } }Output Number = 2 Number = 1 Number = 9 Number = 6 Number = 4 The sorted int array is: Number = 1 Number = 2 Number = 4 Number = 6 Number = 9

13K+ Views
The conditional operator is also known as the ternary operator. This operator consists of three operands and is used to evaluate Boolean expressions. The goal of the operator is to decide; which value should be assigned to the variable. The operator is written as:variable x = (expression)? value if true: value if falseExamplepublic class Test { public static void main(String args[]) { int a, b; a = 10; b = (a == 1) ? 20: 30; System.out.println("Value of b is: " + b); b = (a == 10) ? 20: 30; System.out.println(“Value of b is: " + b); } }OutputValue of b is: 30 Value of b is: 20

2K+ Views
This article will help you understand all about Bitwise Zero Fill Right Shift Zero Operators in Java. Note that Bitwise Zero Fill Right Shift Zero Operator is same as Bitwise Zero Fill Right Shift Operator. Before understanding right shift operators, let us revise about Operators. OPERATORS In computer programming we often need to perform some arithmetical or logical operations. In such circumstances, we need operators to perform these tasks. Thus, an Operator is basically a symbol or token, which performs arithmetical or logical operations and gives us meaningful result. The values involved in the operation are called Operands. In this ... Read More

71 Views
The hashCode(int[]) method of the java.util.Arrays class returns a hash code based on the contents of the specified array. For any two non-null int arrays a and b such that Arrays.equals(a, b), it is also the case that Arrays.hashCode(a) == Arrays.hashCode(b).Exampleimport java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { int[] ival = new int[] { 3, 5 }; int retval = ival.hashCode(); System.out.println("The hash code of value1 is: " + retval); ival = new int[] { 19, 75 }; retval = ival.hashCode(); ... Read More

85 Views
The hashCode(Object[]) method of the java.util.Arrays class returns a hash code based on the contents of the specified array. If the array contains other arrays of elements, the hash code is based on their identities rather than their contents. For any two arrays a and b such that Arrays.equals(a, b), it is also the case that Arrays.hashCode(a) == Arrays.hashCode(b). Example import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { Object[] ob = new Object[] { 22, 7 }; ... Read More

74 Views
The remove(Object) method of the class java.util.ArrayList removes the first occurrence of the specified element from this list, if it is present. If the list does not contain the element, it is unchanged.Exampleimport java.util.ArrayList; public class ArrayListDemo { public static void main(String[] args) { ArrayList arrlist = new ArrayList(5); arrlist.add("G"); arrlist.add("E"); arrlist.add("F"); arrlist.add("M"); arrlist.add("E"); System.out.println("Size of list: " + arrlist.size()); for (String value : arrlist) { System.out.println("Value = " + ... Read More