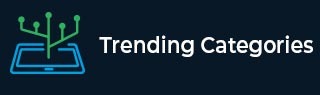
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

4K+ Views
Call by Value means calling a method with a parameter as value. Through this, the argument value is passed to the parameter.While Call by Reference means calling a method with a parameter as a reference. Through this, the argument reference is passed to the parameter.In call by value, the modification done to the parameter passed does not reflect in the caller's scope while in the call by reference, the modification done to the parameter passed are persistent and changes are reflected in the caller's scope.But Java uses only call by value. It creates a copy of references and pass them ... Read More

20K+ Views
HashMap is non-syncronized and is not thread safe while HashTable is thread safe and is synchronized. HashMap allows one null key and values can be null whereas HashTable doesn't allow null key or value. HashMap is faster than HashTable. HashMap iterator is fail-safe where HashTable iterator is not fail-safe. HashMap extends AbstractMap class where HashTable extends Dictionary class.
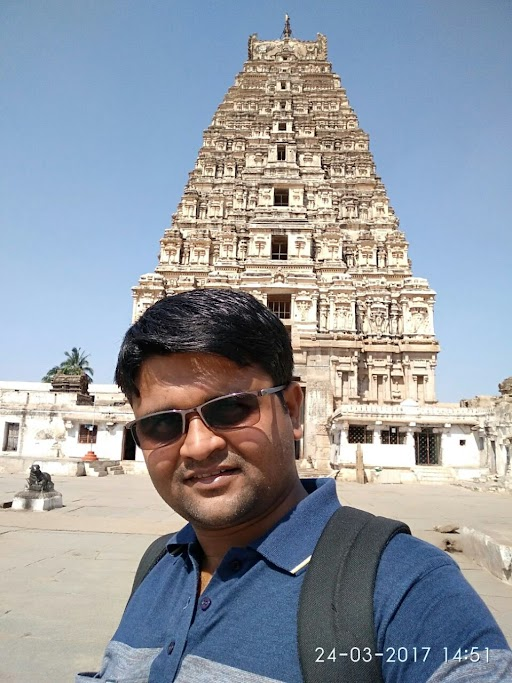
1K+ Views
Classes and objects are considered as the building blocks of object-oriented programming. Every entity with state and behavior is an object. Collection of these similar kinds of objects is a class. A class can be only accessed by its objects hence securing data in it. Read this article to learn more about objects and classes in Java and how they are different from each other. What are Classes in Java? A class is a user-defined data type that acts as a blueprint for designing the objects in it. It is said to be a container that stores objects of similar ... Read More

13K+ Views
A command-line argument is an information that directly follows the program's name on the command line when it is executed. To access the command-line arguments inside a Java program is quite easy. They are stored as strings in the String array passed to main( ).ExampleThe following program displays all of the command-line arguments that it is called with -public class CommandLine { public static void main(String args[]) { for(int i = 0; i

505 Views
Following program uses few of the important tags available for documentation comments. You can make use of other tags based on your requirements.The documentation about the AddNum class will be produced in HTML file AddNum.html but at the same time, a master file with a name index.html will also be created.import java.io.*; /** * Add Two Numbers! * The AddNum program implements an application that * simply adds two given integer numbers and Prints * the output on the screen. * * Note: Giving proper comments in your program makes it more ... Read More

2K+ Views
strictfp is used to ensure that floating points operations give the same result on any platform. As floating points precision may vary from one platform to another. strictfp keyword ensures the consistency across the platforms.strictfp can be applied to class, method or on interfaces but cannot be applied to abstract methods, variable or on constructors.ExampleFollowing are the valid examples of strictfp usage.strictfp class Test { } strictfp interface Test { } class A { strictfp void Test() { } }Following are the invalid strictfp usage.class A { strictfp float a; } class A { strictfp abstract void test(); } class A { strictfp A() { } }

49K+ Views
Call by Value means calling a method with a parameter as value. Through this, the argument value is passed to the parameter.While Call by Reference means calling a method with a parameter as a reference. Through this, the argument reference is passed to the parameter.In call by value, the modification done to the parameter passed does not reflect in the caller's scope while in the call by reference, the modification done to the parameter passed are persistent and changes are reflected in the caller's scope.Following is the example of the call by value −The following program shows an example of ... Read More

715 Views
Following is a simple example of a single dimensional array.Example Live Demopublic class Tester { public static void main(String[] args) { double[] myList = {1.9, 2.9, 3.4, 3.5}; // Print all the array elements for (double element: myList) { System.out.print(element + " "); } } }Output1.9 2.9 3.4 3.5

198 Views
Java supports single dimensional and multidimensional arrays. See the example below:Example Live Demopublic class Tester { public static void main(String[] args) { double[] myList = {1.9, 2.9, 3.4, 3.5}; // Print all the array elements for (double element: myList) { System.out.print(element + " "); } System.out.println(); int[][] multidimensionalArray = { {1,2},{2,3}, {3,4} }; for(int i = 0 ; i < 3 ; i++) { //row for(int j = 0 ; j < 2; j++) { System.out.print(multidimensionalArray[i][j] + " "); } System.out.println(); } } }Output1.9 2.9 3.4 3.5 1 2 2 3 3 4