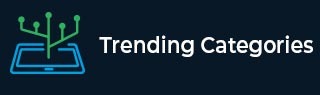
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
We are required to write a JavaScript function that takes in a string as the only argument. The function should construct a new reversed string based on the input string using the for a loop.ExampleFollowing is the code −const str = 'this is the original string'; const reverseString = (str = '') => { let reverse = ''; const { length: len } = str; for(let i = len - 1; i >= 0; i--){ reverse += str[i]; }; return reverse; }; console.log(reverseString(str));OutputFollowing is the output on console −gnirts lanigiro eht si siht

822 Views
We are required to write a JavaScript function that takes in a string that might contain some opening and closing brackets. The function should whether for all the opening brackets there exists a closing bracket or not. If the brackets are rightly matched, the function should return true, false otherwise.For example −f('(hello (world))') = true f('(hello (world)') = falseExampleFollowing is the code −const str1 = '(hello (world))'; const str2 = '(hello (world)'; const validateBrackets = (str = '') => { const strArr = str.split(''); let counter = 0; for (let i = 0, len = strArr.length; i ... Read More

1K+ Views
We are required to write a JavaScript function that takes in two strings let's call them str1 and str2.The size of str1 is guaranteed to be greater than that of str2. We are required to find the smallest substring in str1 that contains all the characters contained in str2.For example −If the input strings are −const str1 = 'abcdefgh'; const str2 = 'gedcf';Then the output should be −const output = 'cdefg';because this the smallest consecutive substring of str1 that contains all characters of str2.ExampleFollowing is the code −const str1 = 'abcdefgh'; const str2 = 'gedcf'; const subIncludesAll = (str, str2) ... Read More

117 Views
We are required to write a JavaScript function that takes in a string as the first argument and a separator character as the second argument.The first string is guaranteed to be a camelCased string. The function should convert the case of the string by separating the words by the separator provided as the second argument.For example −If the input string is −const str = 'thisIsAString'; const separator = '_';Then the output string should be −const output = 'this_is_a_string';ExampleFollowing is the code −const str = 'thisIsAString'; const separator = '_'; const separateCase = (str = '', separator = ' ') => ... Read More

435 Views
We are required to write a JavaScript function that takes in a string as the only argument.The string might contain both uppercase and lowercase alphabets.The function should construct a new string based on the input string in which all the uppercase letters are converted to lowercase and all the lowercase letters are converted to uppercase.ExampleFollowing is the code −const str = 'ThIs Is A STriNG'; const findLetter = (char = '') => { if(char.toLowerCase() === char.toUpperCase){ return char; }else if(char.toLowerCase() === char){ return char.toUpperCase(); }else{ return char.toLowerCase(); ... Read More

3K+ Views
It is a common practice that when websites display anyone's private email address they often mask it in order to maintain privacy.Therefore for example −If someone's email address is −const email = 'ramkumar@example.com';Then it is displayed like this −const masked = 'r...r@example.com';We are required to write a JavaScript function that takes in an email string and returns the masked email for that string.ExampleFollowing is the code −const email = 'ramkumar@example.com'; const maskEmail = (email = '') => { const [name, domain] = email.split('@'); const { length: len } = name; const maskedName = name[0] + '...' + ... Read More

557 Views
The partition of a positive integer n is a way of writing n as a sum of positive integers. Two sums that differ only in the order of their summands are considered the same partition.For example, 4 can be partitioned in five distinct ways −4 3 + 1 2 + 2 2 + 1 + 1 1 + 1 + 1 + 1We are required to write a JavaScript function that takes in a positive integer as the only argument. The function should find and return all the possible ways of partitioning that integer.ExampleFollowing is the code −const findPartitions = (num = 1) => { const arr = Array(num + 1).fill(null).map(() => { return Array(num + 1).fill(null); }); for (let j = 1; j

651 Views
We are required to write a JavaScript function that takes in a positive integer as the only argument. The function should find and return the square root of the number provided as the input.ExampleFollowing is the code −const squareRoot = (num, precision = 0) => { if (num deviation) { res -= ((res ** 2) - num) / (2 * res); }; return Math.round(res * (10 ** precision)) / (10 ** precision); }; console.log(squareRoot(16)); console.log(squareRoot(161, 3)); console.log(squareRoot(1611, 4));OutputFollowing is the output on console −4 12.689 40.1373

149 Views
We are required to write a JavaScript function that takes in two arrays of literals, let’s call them arr1 and arr2.The function should find the longest common streak of literals in the arrays. The function should finally return an array of those literals.For example −If the input arrays are −const arr1 = ['a', 'b', 'c', 'd', 'e']; const arr2 = ['k', 'j', 'b', 'c', 'd', 'w'];Then the output array should be −const output = ['b', 'c', 'd'];ExampleFollowing is the code −const arr1 = ['a', 'b', 'c', 'd', 'e']; const arr2 = ['k', 'j', 'b', 'c', 'd', 'w']; const longestCommonSubsequence = ... Read More

240 Views
We are required to write a JavaScript function that takes in an array of array of positive and negative integers. Since the array also contains negative elements, the sum of contiguous elements can possibly be negative or positive.Our function should pick an array of contiguous elements from the array that sums the greatest. Finally, the function should return that array.For example −If the input array is −const arr = [-2, -3, 4, -1, -2, 1, 5, -3];Then the maximum possible sum is 7 and the output subarray should be −const output = [4, -1, -2, 1, 5];ExampleFollowing is the code ... Read More