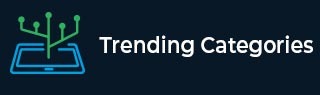
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

807 Views
You should get a start time before making a call and end time after method execution. The difference is the time taken. ExampleLive Demoimport java.util.Calendar; public class Tester { public static void main(String[] args) { long startTime = Calendar.getInstance().getTimeInMillis(); longRunningMethod(); long endTime = Calendar.getInstance().getTimeInMillis(); System.out.println("Time taken: " + (endTime - startTime) + " ms"); } public static void longRunningMethod() { try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } }OutputTime taken: 1012 ms

234 Views
NullPointerException is a runtime exception and it is thrown when the application try to use an object reference which has a null value.For example, using a method on a null reference.Object ref = null; ref.toString(); // this will throw a NullPointerException

533 Views
From Java 8 onwards, static methods are allowed in Java interfaces.An interface can also have static helper methods from Java 8 onwards. public interface vehicle { default void print() { System.out.println("I am a vehicle!"); } static void blowHorn() { System.out.println("Blowing horn!!!"); } }Default Method ExampleCreate the following Java program using any editor of your choice in, say, C:\> JAVA.Java8Tester.javaLive Demopublic class Java8Tester { public static void main(String args[]) { Vehicle vehicle = new Car(); vehicle.print(); } } interface Vehicle { default void print() { ... Read More

180 Views
Let us look at a simple code that will print the words Hello World.ExampleLive Demopublic class MyFirstJavaProgram { /* This is my first java program. * This will print 'Hello World' as the output */ public static void main(String []args) { System.out.println("Hello World"); // prints Hello World } }Let's look at how to save the file, compile, and run the program. Please follow the subsequent steps −Open notepad and add the code as above.Save the file as: MyFirstJavaProgram.java.Open a command prompt window and go to the directory where you saved the class. Assume ... Read More

373 Views
Let us look at a simple code that will print the words Hello World.ExampleLive Demopublic class MyFirstJavaProgram { /* This is my first java program. * This will print 'Hello World' as the output */ public static void main(String []args) { System.out.println("Hello World"); // prints Hello World } }Let's look at how to save the file, compile, and run the program. Please follow the subsequent steps −Open notepad and add the code as above.Save the file as: MyFirstJavaProgram.java.Open a command prompt window and go to the directory where ... Read More

8K+ Views
Following example helps to determine the rows and columns of a two-dimensional array with the use of arrayname.length.ExampleFollowing example helps to determine the upper bound of a two dimensional array with the use of arrayname.length. public class Main { public static void main(String args[]) { String[][] data = new String[2][5]; System.out.println("Dimension 1: " + data.length); System.out.println("Dimension 2: " + data[0].length); } }OutputThe above code sample will produce the following result. Dimension 1: 2 Dimension 2: 5

5K+ Views
Yes, use String.split() method to do it. See the example below −Examplepublic class Tester { public static void main(String[] args) { String text = "This,is,a,comma,seperated,string."; String[] array = text.split(","); for(String value:array) { System.out.print(value + " "); } } }OutputThis is a comma seperated string.

14K+ Views
You can simply iterate the byte array and print the byte using System.out.println() method.Examplepublic class Tester { public static void main(String[] args) { byte[] a = { 1,2,3}; for(int i=0; i< a.length ; i++) { System.out.print(a[i] +" "); } } }Output1 2 3

1K+ Views
Yes. Create a list to represent a 2D array and then use Collections.shuffle(list).Exampleimport java.util.ArrayList; import java.util.Collections; import java.util.List; public class Tester { public static void main(String[] args) { List rows = new ArrayList(); rows.add(new int[]{1,2,3}); rows.add(new int[]{4,5,6}); rows.add(new int[]{7,8,9}); System.out.println("Before Shuffle"); System.out.println("[0][0] : " + rows.get(0)[0]); System.out.println("[1][1] : " + rows.get(1)[1]); System.out.println("After Shuffle"); Collections.shuffle(rows); System.out.println("[0][0] : " + rows.get(0)[0]); System.out.println("[1][1] : " + rows.get(1)[1]); } }OutputBefore Shuffle [0][0] : 1 [1][1] : 5 After Shuffle [0][0] : 7 [1][1] : 2

445 Views
Following program shows how to initialize an array declared earlier.Examplepublic class Tester { int a[]; public static void main(String[] args) { Tester tester = new Tester(); tester.initialize(); } private void initialize() { a = new int[3]; a[0] = 0; a[1] = 1; a[2] = 2; for(int i=0; i< a.length ; i++) { System.out.print(a[i] +" "); } } }Output0 1 2