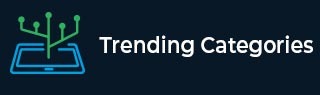
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

585 Views
ExampleLive Demoimport java.util.ArrayList; import java.util.List; import java.util.Scanner; import java.util.regex.Matcher; import java.util.regex.Pattern; public class SearchRegex { private Pattern subPattern = Pattern.compile(SUBJECT_PATTERN); private Matcher matcher; private static final String SUBJECT_PATTERN = "(?s)Subject 1:\s(.*)Subject 2:"; public static void main(String[] args) { String d = "Subject 1: Java" + "Subject 2: Python"; SearchRegex obj = new SearchRegex(); List list = obj.getSubject(d); System.out.println("Address Result : " + list); } private List getSubject(String d){ List result = new ArrayList(); matcher = subPattern.matcher(d); while (matcher.find()) { result.add(matcher.group(1)); } return result; } }OutputAddress Result : [Java]

216 Views
the syntax? i:x makes the string search case-insensitive. for egpublic class RegCaseSense { public static void main(String[] args) { String stringSearch = "HI we are at java class."; // this won't work because the pattern is in upper-case System.out.println("Try this 1: " + stringSearch.matches(".*CLASS.*")); // the magic (?i:X) syntax makes this search case-insensitive, so it returns true System.out.println("Try this 2: " + stringSearch.matches("(?i:.*CLASS.*)")); } }

727 Views
There are many sites which are a good resource for java interview questions-answers. Following is the list of most popular websites.Tutorialspointwww.Tutorialspoint.comStackOverflowwww.stackoverflow.comDZonewww.dzone.comWikipediawww.wikipedia.orgIBM Developer Workswww.ibm.com/developerworks/java/

180 Views
Widening refers to passing a lower size data type like int to a higher size data type like long. Method overloading is possible in such case. ExampleLive Demopublic class Tester { public static void main(String args[]) { Tester tester = new Tester(); short c = 1, d = 2; int e = 1, f = 2; System.out.println(tester.add(c, d)); System.out.println(tester.add(e, f)); } public int add(short a, short b) { System.out.println("short"); return a + b; } public int add(int a, int b) { System.out.println("int"); return a + b; } } OutputShort 3 Int 3

69 Views
There are three kinds of variables in Java −Local variablesInstance variablesClass/Static variablesLocal VariablesLocal variables are declared in methods, constructors, or blocks.Local variables are created when the method, constructor or block is entered and the variable will be destroyed once it exits the method, constructor, or block.Access modifiers cannot be used for local variables.Local variables are visible only within the declared method, constructor, or block.Local variables are implemented at stack level internally.There is no default value for local variables, so local variables should be declared and an initial value should be assigned before the first use.ExampleHere, age is a local variable. ... Read More

703 Views
This article will help you understand all differences between a Java method and a native method. Function / Method A program module (a part of the program) used simultaneously at different instances in a program to perform specific task is known as Method or Function. It can be regarded as a black box that is capable of returning an output (obtained as per execution of the codes written inside). Similarly, all the methods available in Java class act as a black box. On providing values (arguments) to a method, it processes the code available within it and returns the output. ... Read More

1K+ Views
A variable provides us with named storage that our programs can manipulate. Each variable in Java has a specific type, which determines the size and layout of the variable's memory; the range of values that can be stored within that memory; and the set of operations that can be applied to the variable.You must declare all variables before they can be used. Following is the basic form of a variable declaration − data type variable [ = value][, variable [ = value] ...] ;Here data type is one of Java's datatypes and variable is the name of the variable. To ... Read More

500 Views
All Java components require names. Names used for classes, variables and methods are called identifiers. In Java, there are several points to remember about identifiers. They are as follows -All identifiers should begin with a letter (A to Z or a to z), currency character ($) or an underscore (_). After the first character, identifiers can have any combination of characters.A keyword cannot be used as an identifier. Most importantly, identifiers are case sensitive. Examples of legal identifiers: age, $salary, _value, __1_value. Examples of illegal identifiers: 123abc, -salary.