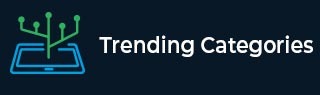
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

275 Views
The equals() methodThis method compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.Exampleimport java.lang.*; public class StringDemo { public static void main(String[] args) { String str1 = "sachin tendulkar"; String str2 = "amrood admin"; String str3 = "amrood admin"; // checking for equality boolean retval1 = str2.equals(str1); boolean retval2 = str2.equals(str3); ... Read More

116 Views
The compareTo() method in Java compares two strings lexicographically.ExampleLive Demopublic class Test { public static void main(String args[]) { String str1 = "Strings are immutable"; String str2 = new String("Strings are immutable"); String str3 = new String("Integers are not immutable"); int result = str1.compareTo( str2 ); System.out.println(result); result = str2.compareTo( str3 ); System.out.println(result); } }Output0 10

254 Views
You can compare two Strings in Java using the compareTo() method, equals() method or == operator.The compareTo() method compares two strings. The comparison is based on the Unicode value of each character in the strings. The character sequence represented by this String object is compared lexicographically to the character sequence represented by the argument string.The result is a negative integer if this String object lexicographically precedes the argument string.The result is a positive integer if this String object lexicographically follows the argument string.The result is zero if the strings are equal, compareTo returns 0 exactly when the equals(Object) method would ... Read More

233 Views
You can check the equality of two Strings in Java using the equals() method. This method compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.Exampleimport java.lang.* public class StringDemo { public static void main(String[] args) { String str1 = "Tutorialspoint"; String str2 = "Tutorialspoint"; String str3 = "Hi"; // checking for equality boolean retval1 = ... Read More

533 Views
The equals() method compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.Examplepublic class Sample{ public static void main(String []args){ String s1 = "tutorialspoint"; String s2 = "tutorialspoint"; String s3 = new String ("Tutorials Point"); System.out.println(s1.equals(s2)); System.out.println(s2.equals(s3)); } }Outputtrue falseYou can also compare two strings using == operator. But, it compares references of the given variables not values.Examplepublic class ... Read More

2K+ Views
The equalsIgnoreCase() method compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.ExampleLive Demopublic class Sample { public static void main(String args[]) { String Str1 = new String("This is really not immutable!!"); String Str2 = Str1; String Str3 = new String("THIS IS REALLY NOT IMMUTABLE!!"); boolean retVal; retVal = Str1.equalsIgnoreCase(Str2); System.out.println("Returned Value = " + retVal ); retVal = Str1.equalsIgnoreCase( Str3 ); System.out.println("Returned Value = " + retVal ); } }OutputReturned Value = true Returned Value = true

1K+ Views
The concat() method of the String class appends one String to the end of another. The method returns a String with the value of the String passed into the method, appended to the end of the String, used to invoke this method.Examplepublic class Test { public static void main(String args[]) { String s = "Strings are immutable"; s = s.concat(" all the time"); System.out.println(s); } }OutputStrings are immutable all the timeThis replace() method of the String class returns a new string resulting from replacing all occurrences of oldChar in ... Read More

3K+ Views
You can compare two strings using either equals() method or compareTo() method.Where, The equals() method compares this string to the specified object.The compareTo() method compares two strings lexicographically. The comparison is based on the Unicode value of each character in the strings.These two methods compare the given strings with respective to Case i.e. String with the different case are treated differently.ExampleFollowing example demonstrates the comparison of two strings using the equals() method.Live Demopublic class Sample{ public static void main(String args[]) { String str = "Hello World"; String anotherString = "hello world"; ... Read More

145 Views
You can concatenate two strings using the concat() method of the String class. This class concatenates the specified string to the end of this string.ExampleLive Demopublic class Test { public static void main(String args[]){ String str1 = "Krishna"; String str2 = "Kasyap"; System.out.println(str1.concat(str2)); } }OutputkrishnaKasyap

466 Views
Java String class provides different comparison methods namely:The compareTo() methodThis method compares two Strings lexicographically. This method returns a negative integer if current String object lexicographically precedes the argument string. a positive integer if current String object lexicographically follows the argument true when the strings are equal.Exampleimport java.lang.*; public class StringDemo { public static void main(String[] args) { String str1 = "tutorials", str2 = "point"; // comparing str1 and str2 int retval = str1.compareTo(str2); // prints the return value of the comparison ... Read More