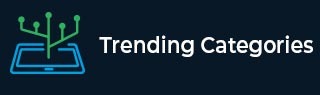
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

609 Views
We are required to write a JavaScript function that takes in a string as the only argument. The function should generate an array of strings that contains all possible contiguous substrings that exist in the array.ExampleFollowing is the code −const str = 'Delhi'; const allCombinations = (str1 = '') => { const arr = []; for (let x = 0, y=1; x < str1.length; x++,y++) { arr[x]=str1.substring(x, y); }; const combination = []; let temp= ""; let len = Math.pow(2, arr.length); for (let i = 0; i < len ; i++){ temp= ""; for (let j=0;j

2K+ Views
We are required to write a JavaScript function that takes in a floating-point number up to 2 digits of precision.The function should convert that number into the Indian current text.For example −If the input number is −const num = 12500Then the output should be −const output = 'Twelve Thousand Five Hundred';ExampleFollowing is the code −const num = 12500; const wordify = (num) => { const single = ["Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"]; const double = ["Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"]; const tens = ["", "Ten", "Twenty", "Thirty", ... Read More

538 Views
We are required to write a JavaScript function that takes in two numbers. The first number represents the length of the base of a right triangle and the second is perpendicular. The function should then compute the length of the hypotenuse based on these values.For example −If base = 8, perpendicular = 6Then the output should be 10ExampleFollowing is the code −const base = 8; const perpendicular = 6; const findHypotenuse = (base, perpendicular) => { const bSquare = base ** 2; const pSquare = perpendicular ** 2; const sum = bSquare + pSquare; const hypotenuse = Math.sqrt(sum); return hypotenuse; }; console.log(findHypotenuse(base, perpendicular)); console.log(findHypotenuse(34, 56));OutputFollowing is the output on console −10 65.5133574166368

401 Views
We are required to write a JavaScript function that takes in a string of characters. The function should construct a new string in which all the non-alphabetic characters from the original string are removed and return that string. If the string contains spaces, then it should not be removed.For example −If the input string is −const str = 'he@656llo wor?ld';Then the output string should be −const str = 'he@656llo wor?ld';ExampleFollowing is the code −const str = 'he@656llo wor?ld'; const isAlphaOrSpace = char => ((char.toLowerCase() !== char.toUpperCase()) || char === ' '); const removeSpecials = (str = '') => { ... Read More

606 Views
We are required to write a JavaScript function that takes in a string that represents a ASCII number. The function should convert the number into its corresponding hexadecimal code and return the hexadecimal.For example −f the input ASCII string is −const str = '159';Then the hexadecimal code for this should be 313539.ExampleFollowing is the code −const str = '159'; const convertToHexa = (str = '') =>{ const res = []; const { length: len } = str; for (let n = 0, l = len; n < l; n ++) { const hex = ... Read More

942 Views
Union SetUnion Set is the set made by combining the elements of two sets. Therefore, the union of sets A and B is the set of elements in A, or B, or both.For example −If we have two sets denoted by two arrays like this −const arr1 = [1, 2, 3]; const arr2 = [100, 2, 1, 10];Then the union set will be −const union = [1, 2, 3, 10, 100];We are required to write a JavaScript function that takes in two such arrays of literals and returns their union array.ExampleFollowing is the code −const arr1 = [1, 2, 3]; ... Read More

305 Views
We are required to write a JavaScript function that takes in a nested array of literals as the only argument. The function should construct a new array that contains all the literal elements present in the input array but without nesting.For example −If the input array is −const arr = [ 1, 3, [5, 6, [7, [6, 5], 4], 3], [4] ];Then the output array should be −const output = [1, 3, 5, 6, 7, 6, 5, 4, 3, 4];ExampleFollowing is the code −const arr = [ 1, 3, [5, 6, [7, [6, 5], 4], 3], [4] ]; ... Read More

148 Views
We are required to write a JavaScript function that takes in an array of Numbers as the only argument. The function should calculate the sum of all numbers in the array and the product of all numbers. Then the function should return the absolute difference between the sum and the product.ExampleFollowing is the code −const arr = [1, 4, 1, 2, 1, 6, 3]; const sumProductDifference = (arr = []) => { const creds = arr.reduce((acc, val) => { let { sum, product } = acc; sum += val; product *= val; return { sum, product }; }, { sum: 0, product: 1 }); const { sum, product } = creds; return Math.abs(sum - product); }; console.log(sumProductDifference(arr));OutputFollowing is the output on console −126

138 Views
We are required to write a JavaScript function that takes in an array of sorted numbers. The function should calculate the mean and the mode of the dataset. Then if the mean and mode are equal, the function should return true, false otherwise.For example −If the input array is −const arr = [5, 3, 3, 3, 1];Then the output for this array should be true because both the mean and median of this array are 3.ExampleFollowing is the code −const arr = [5, 3, 3, 3, 1]; mean = arr => (arr.reduce((a, b) => a + b))/(arr.length); mode = arr ... Read More

356 Views
We are required to write a JavaScript function that takes in a string as the only argument. The function should then iterate through the string and find and return the longest word from the string.For example −If the input string is −const str = 'Coding in JavaScript is really fun';Then the output string should be −const output = 'JavaScript';ExampleFollowing is the code −const str = 'Coding in JavaScript is really fun'; const findLongest = (str = '') => { const strArr = str.split(' '); const word = strArr.reduce((acc, val) => { let { length: len ... Read More