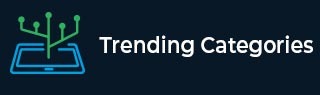
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

122 Views
You can compare Strings using compareTo() method or, equals() method or, or == operator. Following example demonstrates how to initialize and compare strings in Java. Example Live Demo public class StringDemo { public static void main(String[] args) { String str1 = "tutorials"; String str2 = "point"; // comparing str1 and str2 int retval = str1.compareTo(str2); // prints the return value of the comparison if (retval < 0) { System.out.println("str1 is greater than str2"); } else if (retval == 0) { System.out.println("str1 is equal to str2"); } else { System.out.println("str1 is less than str2"); } } } Output str1 is less than str2

1K+ Views
The append() method in the StringBuffer class adds the specified String to the contents of the current String buffer. Using this method you can append a single character to a string or char array in java.Examplepublic class Test { public static void main(String args[]) { StringBuffer sb = new StringBuffer("Hi hello how are yo"); sb.append("u"); System.out.println(sb); } }OutputHi hello how are you

332 Views
Java memory pool is divided into 5 parts namely −Method area − The method area stores the class code − code of the variables and methods.Heap−The Java objects are created in this area.Java Stack− While running methods the results are stored in the stack memory.PC registers− These contain the address of the instructions of the methods.Native method stacks− Similar to Java stack, native methods are executed on the Native method stacks.

3K+ Views
Java Virtual Machine is a program/software which takes Java bytecode (.class files)and converts the byte code (line by line) into machine understandable code.JVM contains a module known as a class loader. A class loader in JVM loads, links and, initializes a program. It−Loads the class into the memory. Verifies the byte code instructions.Allocates memory for the program.The memory in the JVM is divided into five different parts namely− Method area− The method area stores the class code − code of the variables and methods. Heap − The Java objects are created in this area. Java Stack− While running methods the results are stored in ... Read More

160 Views
Following is an example which compares Strings and portion of strings in Java? Explain with an example.ExampleLive Demopublic class StringDemo { public static void main(String[] args) { String str1 = "tutorials point"; String str2 = str1.substring(10); int result = str1.compareTo(str2); // prints the return value of the comparison if (result < 0) { System.out.println("str1 is greater than str2"); } else if (result == 0) { System.out.println("str1 is equal to str2"); } else { System.out.println("str1 is less than str2"); } } }Outputstr1 is less than str2

2K+ Views
The equals() method compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.Example Live Demopublic class Sample { public static void main(String []args) { String s1 = "tutorialspoint"; String s2 = "tutorialspoint"; String s3 = new String ("Tutorials Point"); System.out.println(s1.equals(s2)); System.out.println(s2.equals(s3)); } }Outputtrue falseYou can also compare two strings using == operator. But, it compares references of the given ... Read More

224 Views
You can compare two Strings in Java using the compareTo() method, equals() method or == operator.The compareTo() method compares two strings. The comparison is based on the Unicode value of each character in the strings. The character sequence represented by this String object is compared lexicographically to the character sequence represented by the argument string. The result is a negative integer if this String object lexicographically precedes the argument string.The result is a positive integer if this String object lexicographically follows the argument string. The result is zero if the strings are equal, compareTo returns 0 exactly when the equals(Object) method would ... Read More

1K+ Views
We all know that the String class in Java is mutable i.e. once we create a String variable we cannot modify its data or do any manipulations.But, there may be scenarios where we need to modify the data of String variables. In such cases, we could use StringBuffer class.This class −is like a String, but can be modified. It contains some particular sequence of characters, but the length and content of the sequence can be changed through certain method calls.Is safe for use by multiple threads.

2K+ Views
The compareTo() method compares two strings lexicographically. The comparison is based on the Unicode value of each character in the strings. The character sequence represented by this String object is compared lexicographically to the character sequence represented by the argument string.The result is a negative integer if this String object lexicographically precedes the argument string. The result is a positive integer if this String object lexicographically follows the argument string. The result is zero if the strings are equal, compareTo returns 0 exactly when the equals(Object) method would return true.ExampleLive Demopublic class StringDemo { public static void main(String[] args) { ... Read More

15K+ Views
You can check the equality of two Strings in Java using the equals() method. This method compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.Exampleimport java.lang.* public class StringDemo { public static void main(String[] args) { String str1 = "Tutorialspoint"; String str2 = "Tutorialspoint"; String str3 = "Hi"; // checking for equality boolean retval1 = ... Read More