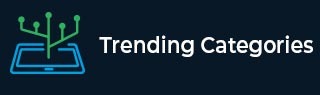
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

4K+ Views
The split(String regex) method of the String class splits this string around matches of the given regular expression.This method works in the same way as invoking the method i.e split(String regex, int limit) with the given expression and a limit argument of zero. Therefore, trailing empty strings are not included in the resulting array.ExampleLive Demoimport java.lang.*; public class StringDemo { public static void main(String[] args) { String str = "a d, m, i.n"; String delimiters = "\s+|, \s*|\.\s*"; // analysing the string String[] tokensVal = str.split(delimiters); ... Read More

880 Views
The StringTokenizer class allows an application to break a string into tokens.This class is a legacy class that is retained for compatibility reasons although its use is discouraged in new code.ExampleLive Demoimport java.util.*; public class Sample { public static void main(String[] args) { // creating string tokenizer StringTokenizer st = new StringTokenizer("Come to learn"); // counting tokens System.out.println("Total tokens : " + st.countTokens()); // checking tokens while (st.hasMoreTokens()) { System.out.println("Next token : " + st.nextToken()); ... Read More

105 Views
We can verify whether the given string is empty using the isEmpty() method of the String class. This method returns true only if length() is 0.ExampleLive Demoimport java.lang.*; public class StringDemo { public static void main(String[] args) { String str = "tutorialspoint"; // prints length of string System.out.println("length of string = " + str.length()); // checks if the string is empty or not System.out.println("is this string empty? = " + str.isEmpty()); } }Outputlength of string = 14 is this string empty? = false

982 Views
The replace method of the String class accepts two characters and it replaces all the occurrences of oldChar in this string with newChar.Exampleimport java.io.*; public class Test { public static void main(String args[]) { String Str = new String("Welcome to Tutorialspoint.com"); System.out.print("Return Value :" ); System.out.println(Str.replace('o', 'T')); System.out.print("Return Value :" ); System.out.println(Str.replace('l', 'D')); } }OutputReturn Value :WelcTme tT TutTrialspTint.cTm Return Value :WeDcome to TutoriaDspoint.comThe replaceAll() method replaces each substring of this string that matches the given regular expression with the given replacement.Exampleimport java.io.*; ... Read More

12K+ Views
We can check whether a particular String is empty or not, using isBlank() method of the StringUtils class. This method accepts an integer as a parameter and returns true if the given string is empty, or false if it is not. Example Live Demo import org.apache.commons.lang3.StringUtils; public class Sample { public static void main(String args[]) { String str = ""; Boolean bool = StringUtils.isBlank(str); System.out.println(bool); } } Output true

212 Views
The replace method of the String class accepts two characters and it replaces all the occurrences of oldChar in this string with newChar.Example Live Demoimport java.io.*; public class Test { public static void main(String args[]) { String Str = new String("Welcome to Tutorialspoint.com"); System.out.print("Return Value :" ); System.out.println(Str.replace('o', 'T')); System.out.print("Return Value :" ); System.out.println(Str.replace('l', 'D')); } }OutputReturn Value :WelcTme tT TutTrialspTint.cTm Return Value :WeDcome to TutoriaDspoint.com

217 Views
The isEmpty() method of the String class returns true if the length of the current string is 0.ExampleLive Demoimport java.lang.*; public class StringDemo { public static void main(String[] args) { String str = "tutorialspoint"; //prints length of string System.out.println("length of string = " + str.length()); //checks if the string is empty or not System.out.println("is this string empty? = " + str.isEmpty()); } }Outputlength of string = 14 is this string empty? = false

445 Views
We can verify whether the given string is empty using the isEmpty() method of the String class. This method returns true only if length() is 0.ExampleLive Demoimport java.lang.*; public class StringDemo { public static void main(String[] args) { String str = "tutorialspoint"; // prints length of string System.out.println("length of string = " + str.length()); // checks if the string is empty or not System.out.println("is this string empty? = " + str.isEmpty()); } }Outputlength of string = 14 is this string empty? = false

44 Views
This method has two variants. The first variant converts all of the characters in this String to upper case using the rules of the given Locale. This is equivalent to calling toUpperCase(Locale.getDefault()).The second variant takes a locale as an argument to be used while converting into upper case.ExampleLive Demoimport java.io.*; public class Test { public static void main(String args[]) { String Str = new String("Welcome to Tutorialspoint.com"); System.out.print("Return Value :" ); System.out.println(Str.toUpperCase() ); } }OutputReturn Value :WELCOME TO TUTORIALSPOINT.COM

79 Views
The contains() method of the String class returns true if and only if this string contains the specified sequence of char values.Example Live Demoimport java.lang.*; public class StringDemo { public static void main(String[] args) { String str1 = "tutorials point", str2 = "http://"; CharSequence cs1 = "int"; //string contains the specified sequence of char values boolean retval = str1.contains(cs1); System.out.println("Method returns : " + retval); //string does not contain the specified sequence of char value retval = str2.contains("_"); System.out.println("Methods returns: " + retval); } }OutputMethod returns : true Methods returns: false