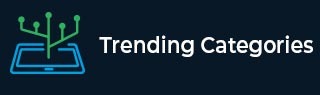
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

899 Views
In this article, we will understand how to read a number from standard input in Java. The Scanner.nextInt() method is used to read the number. The java.util.Scanner.nextInt() method Scans the next token of the input as an int. An invocation of this method of the form nextInt() behaves in exactly the same way as the invocation nextInt(radix), where radix is the default radix of this scanner.Below is a demonstration of the same −InputSuppose our input is −55OutputThe desired output would be −The input value is 55AlgorithmStep1- Start Step 2- Declare an integer: value Step 3- Prompt the user to enter ... Read More

9K+ Views
When we enter the coding world there are various mathematical operations that we learn to do through programming languages. We are expected to begin our coding journey with basic mathematical operations like adding, subtracting, multiplying and dividing. To evaluate the sum of two numbers, we use the '+' operator. However, Java also provides other approaches for adding two numbers. This article aims to explore the possible ways to add the two numbers in Java. To add the two numbers in Java, we are going to use the following approaches − By taking input of operands from user By ... Read More

15K+ Views
In this article, we will understand how to print a string in Java. A string is a pattern of characters and alphanumeric values. The easiest way to create a string is to write −String str = "Welcome to the club!!!"Whenever it encounters a string literal in your code, the Java compiler creates a String object with its value in this case, " Welcome to the club!!!'.As with any other object, you can create String objects by using the new keyword and a constructor. The String class has 11 constructors that allow you to provide the initial value of the string ... Read More

1K+ Views
We can handle authentication popup in Selenium webdriver using Java. To do this, we have to pass the user credentials within the URL. We shall have to add the username and password to the URL.Syntax −https://username:password@URL https://admin:admin@the-internet.herokuapp.com/basic_auth Here, the admin is the username and password. URL – www.the-internet.herokuapp.com/basic_auth Let us work and accept the below authentication popup.ExampleCode Implementation.import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class AuthnPopup{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); String u = "admin"; ... Read More

634 Views
We can scroll down a webpage in Selenium using Java. Selenium is unable to handle scrolling directly. It takes the help of the Javascript Executor to perform the scrolling action up to an element.First of all, we have to locate the element up to which we have to scroll. Next, we shall use the Javascript Executor to run the Javascript commands. The method executeScript is used to run Javascript commands in Selenium. We shall take the help of the scrollIntoView method in Javascript and pass true as an argument to the method.Syntax −WebElement elm = driver.findElement(By.name("name")); ((JavascriptExecutor) driver) .executeScript("arguments[0].scrollIntoView(true);", elm);Exampleimport ... Read More
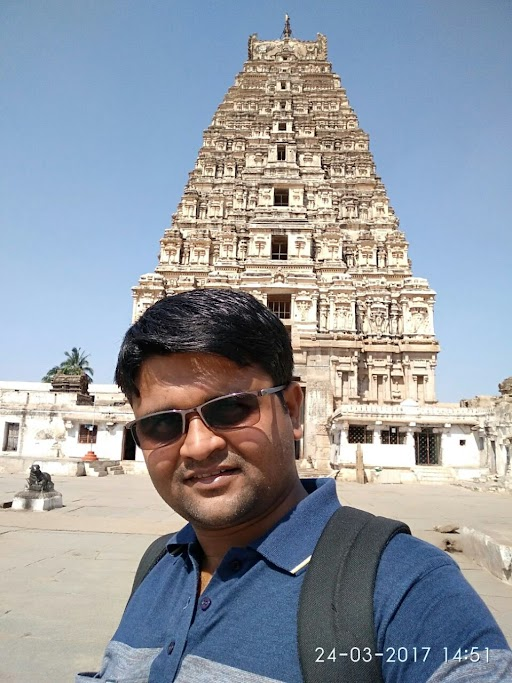
6K+ Views
In computer programming, Inheritance and Polymorphism are two important concepts. The most basic difference between inheritance and polymorphism is that "inheritance" is a concept of objectoriented programming that allows creating a new class with the help of the features of an existing class, whereas the concept "polymorphism" represents multiple forms of a single function. Read this article to learn more about Inheritance and Polymorphism and how they are different from each other. What is Inheritance? Inheritance is a concept in object-oriented programming (OOP) that refers to the process by which an object can take on the features of one or ... Read More
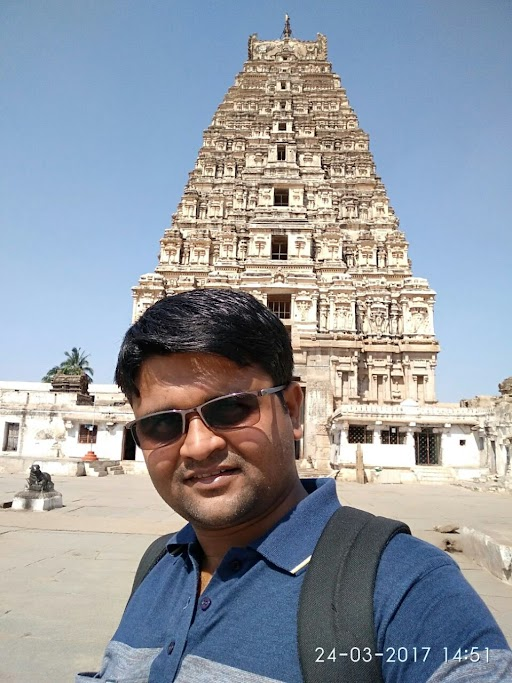
48K+ Views
In the top-down approach, a bigger module/problem is divided into smaller modules. In contrast, in the bottom-up approach, the smaller problems are solved and then they are integrated to find the solution of a bigger problem. Read this article to learn more about top-down approach and bottom-up approach and how they are different from each other. What is Top-Down Approach? Top-Down Approach is an approach to design algorithms in which a bigger problem is broken down into smaller parts. Thus, it uses the decomposition approach. This approach is generally used by structured programming languages such as C, COBOL, FORTRAN. The ... Read More

8K+ Views
Given two strings str_1 and str_2. The goal is to count the number of occurrences of substring str2 in string str1 using a recursive process.A recursive function is the one which has its own call inside it’s definition.If str1 is “I know that you know that i know” str2=”know”Count of occurences is − 3Let us understand with examples.For ExampleInputstr1 = "TPisTPareTPamTP", str2 = "TP";OutputCount of occurrences of a substring recursively are: 4ExplanationThe substring TP occurs 4 times in str1.Inputstr1 = "HiHOwAReyouHiHi" str2 = "Hi"OutputCount of occurrences of a substring recursively are: 3ExplanationThe substring Hi occurs 3 times in str1.Approach used ... Read More

686 Views
Identity MatrixAn identity Matrix is a matrix which is n × n square matrix where the diagonal consist of ones and the other elements are all zeros.For example an identity matrix of order is will be −const arr = [ [1, 0, 0], [0, 1, 0], [0, 0, 1] ];We are required to write a JavaScript function that takes in a number, say n, and returns an identity matrix of n*n order.ExampleFollowing is the code −const num = 5; const constructIdentity = (num = 1) => { const res = []; for(let i = 0; ... Read More

584 Views
We are required to write a JavaScript function that takes in a string that might contain some alphabets. The function should count and return the number of vowels that exists in the string.ExampleFollowing is the code −const str = 'this is a string'; const countVowels = (str = '') => { str = str.toLowerCase(); const legend = 'aeiou'; let count = 0; for(let i = 0; i < str.length; i++){ const el = str[i]; if(!legend.includes(el)){ continue; }; count++; }; return count; }; console.log(countVowels(str));OutputFollowing is the output on console −4