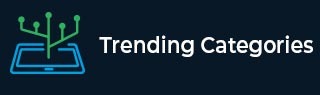
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

141 Views
To define a variable in C++, you need to use the following syntax −Syntaxdatatype variable_name;You need to know what type of data is your variable is going to hold and what it will be called. The variable name has constraints on what you can name it. Following are the rules for naming variables −Variable names in C++ can range from 1 to 255 characters.All variable names must begin with a letter of the alphabet or an underscore(_).After the first initial letter, variable names can also contain letters and numbers. Variable names are case sensitive.No spaces or special characters are allowed.You ... Read More

383 Views
A string literal or anonymous string is a type of literal in programming for the representation of a string value within the source code. More simply put, a string literal is a bit of text between double quotes. For example,const char* var = "Hello";In this definition of var, "Hello" is a string literal. Using const in this way means you can use var to access the string but not to change it. A C++ compiler handles it in the same way as it would handle a character array.

1K+ Views
Character constants are one or more members of the “source character set, ” the character set in which a program is written, surrounded by single quotation marks ('). They are used to represent characters in the “execution character set, ” the character set on the machine where the program executes. These are sometimes also called character literals.In C++, A character literal is composed of a constant character. It is represented by the character surrounded by single quotation marks. There are two kinds of character literals − Narrow-character literals of type char, for example, 'a' Wide-character literals of type wchar_t, for example, L'a'The ... Read More

2K+ Views
Floating-point constants specify values that must have a fractional part.Floating-point constants have a "mantissa, " which specifies the value of the number, an "exponent, " which specifies the magnitude of the number, and an optional suffix that specifies the constant's type(double or float).The mantissa is specified as a sequence of digits followed by a period, followed by an optional sequence of digits representing the fractional part of the number. For example −24.25 12.00These values can also contain exponents. For example, 24.25e3 which is equivalent to 24250In C++ you can use the following code to create a floating point constant −ExampleLive ... Read More

2K+ Views
Integer constants are constant data elements that have no fractional parts or exponents. They always begin with a digit. You can specify integer constants in decimal, octal, or hexadecimal form. They can specify signed or unsigned types and long or short types.In C++ you can use the following code to create an integer constant −#include using namespace std; int main() { const int x = 15; // 15 is decimal integer constant while x is a constant int. int y = 015; // 15 is octal integer constant while y is an int. return 0; }You can ... Read More

578 Views
A literal is a value that is expressed as itself. For example, the number 25 or the string "Hello World" are both literals.A constant is a data type that substitutes a literal. Constants are used when a specific, unchanging value is used various times during the program. For example, if you have a constant named PI that you'll be using at various places in your program to find the area, circumference, etc of a circle, this is a constant as you'll be reusing its value. But when you'll be declaring it as −const float PI = 3.141;The 3.141 is a ... Read More

402 Views
A literal is any notation for representing a value within the source code. They just exist in your source code and do not have any reference a value in memory. Contrast this with identifiers, which refer to a value in memory.There are several types of literals in C++. Some of the examples of literals are −"Hello" (a string)3.141 (a float/double)true (a boolean)3 (an integer)'c' (a character)Things that are not literals −bar = 0; (a statement)3*5-4 (an expression)std::cin (an identifier)

1K+ Views
Variable and constant are two commonly used mathematical concepts. Simply put, a variable is a value that is changing or that have the ability to change. A constant is a value which remains unchanged.For example, if you have a program that has a list of 10 radii and you want to calculate the area for all of these circles. To find the area of these circles, you'll write a program that will have a variable that will store the value of PI and this value will not change throughout the program. Such values can be declared as a constant.In the ... Read More

577 Views
There are no types of constants in C++. It's just that you can declare any data type in C++ to be a constant. If a variable is declared as constant using the const keyword, you cannot reassign its value. Example#include using namespace std; int main() { const int i = 5; // Now all of these operations are illegal and // will cause an error: i = 10; i *= 2; i++; i--; //... return 0; }