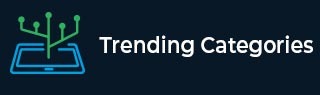
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
Binary search is a fast search algorithm with run-time complexity of Ο(log n). This search algorithm works on the principle of divide and conquer. For this algorithm to work properly, the data collection should be in the sorted form.The binary search looks for a particular item by comparing the middle most item of the collection. If a match occurs, then the index of the item is returned. If the middle item is greater than the item, then the item is searched in the sub-array to the left of the middle item. Otherwise, the item is searched for in the sub-array to the right ... Read More

684 Views
This is an in-place comparison-based sorting algorithm. Here, a sub-list is maintained which is always sorted. For example, the lower part of an array is maintained to be sorted. An element which is to be inserted in this sorted sub-list has to find its appropriate place and then it has to be inserted there. Hence the name, insertion sort.The array is searched sequentially and unsorted items are moved and inserted into the sorted sub-list (in the same array).Algorithm1.If it is the first element, it is already sorted. return 1; 2.Pick next element 3.Compare with all elements in the sorted sub-list ... Read More

3K+ Views
Selection sort is a simple sorting algorithm. This sorting algorithm is an in-place comparison-based algorithm in which the list is divided into two parts, the sorted part at the left end and the unsorted part at the right end. Initially, the sorted part is empty and the unsorted part is the entire list.The smallest element is selected from the unsorted array and swapped with the leftmost element, and that element becomes a part of the sorted array. This process continues moving unsorted array boundary from one element to the right.Algorithm1.Set MIN to location 0 2.Search the minimum element in the ... Read More

2K+ Views
Fibonacci Series generates subsequent number by adding two previous numbers. Fibonacci series starts from two numbers − F0 & F1. The initial values of F0 & F1 can be taken 0, 1 or 1, 1 respectively.Fn = Fn-1 + Fn-2Algorithm1. Take integer variable A, B, C 2. Set A = 1, B = 1 3. DISPLAY A, B 4. C = A + B 5. DISPLAY C 6. Set A = B, B = C 7. REPEAT from 4 - 6, for n timesExampleLive Demopublic class FibonacciSeries2{ public static void main(String args[]) { int a, b, c, i, n; n = 10; a = b = 1; System.out.print(a+" "+b); for(i = 1; i

116 Views
The example works correctly. The compareTo() method is called by a string object and takes another string object as argument. The return value is integer and is difference in Unicode values of characters of respective strings when they are not equal. The value can be -ve, 0 or +ve.

111 Views
Even if removing the color by code:mycanvas.clearColor(d[1],d[2],d[3],2.0); mycanvas.clear(can.COLOR_BUFFER_BIT );The screen gets cleared at beginning of next draw cycle.To create WebGLRenderingContext, previous drawing buffer can be preserved.gl = someCanvas.getContext("webgl", { preserveDrawingBuffer: true }); The default is preserveDrawingBuffer: false by making this property true, previous drawing can be easily preserved

279 Views
the main() method in Java code is itself inside a class. The static keyword lets the main() method which is the entry point of execution without making an object but you need to write a class. In C++, main() is outside the class and writing class it self is not mandatory. Hence, C++ is not a pure object oriented language bu Java is a completely object oriented language.

96 Views
The classList property returns the class name(s) of an element, as a DOMTokenList object. The classList property is read-only, however, you can modify it by using the add() and remove() methods.The classListproperty ensures that duplicate classes are not unnecessarily added to the element. In order to keep this functionality, if you dislike the longhand versions or jQuery version, I’d suggest adding addMany function and removeMany to DOMTokenListThese would then be useable like so −DOMTokenList.prototype.addMany = function(classes) { var arr = classes.split(' '); for (var j = 0, length = arr.length; j < length; j++) { this.add(array[j]); ... Read More

82 Views
1) take a string from the user and check contains atleast one digit or not:Extract character array from string using toCharArray() method. Run a for loop over each character in array and test if it is a digit by static method isDigit() of character classpublic static boolean chkdigit(String str) { char arr[]=str.toCharArray(); for (char ch:arr) { if (Character.isDigit(ch)) { return true; } } return false; }2.) take ... Read More