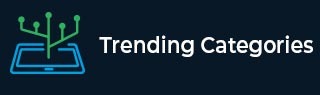
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

6K+ Views
In English alphabet the characters 'a', 'e', 'i', 'o', 'u' are vowels and remaining letters are consonants. To find whether the given letter is a vowel or consonant.Using loop and or operator verify whether given character is 'a' or 'e' or 'i' or 'o' or 'u' else it is consonant.Exampleimport java.util.Scanner; public class VowelOrConsonant { public static void main(String args[]){ System.out.println("Enter a character :"); Scanner sc = new Scanner(System.in); char ch = sc.next().charAt(0); if(ch == 'a'|| ch == 'e'|| ch == 'i' ||ch == 'o' ||ch == ... Read More

2K+ Views
A factorial of a particular number (n) is the product of all the numbers from 0 to n (including n) i.e. Factorial of the number 5 will be 1*2*3*4*5 = 120. To find the factorial of a given number. Create a variable factorial initialize it with 1. start while loop with condition i (initial value 1) less than the given number. In the loop, multiple factorials with i and assign it to factorial and increment i. Finally, print the value of factorial.Exampleimport java.util.Scanner; public class FactorialWithWhileLoop { public static void main(String args[]){ int i =1, factorial=1, number; System.out.println("Enter ... Read More

358 Views
Following is a program to swap two numbers using XOR operator.Examplepublic class ab31_SwapTwoNumberUsingXOR { public static void main(String args[]){ int a,b; Scanner sc = new Scanner(System.in); System.out.println("Enter a value :"); a = sc.nextInt(); System.out.println("Enter b value :"); b = sc.nextInt(); a = a^b; b = a^b; a = a^b; System.out.println("Value of the variable a after swapping : "+a); System.out.println("Value of the variable b after swapping : "+b); } }OutputEnter a value : 55 Enter b value : 64 Value of the variable a after swapping : 64 Value of the variable b after swapping : 55

5K+ Views
The circumference of a circle is double the product of its radius and the value of PI. Therefore, to calculate the circumference of a circleGet the radius of the circle form the user.Calculate the productPrint the final result.Example: Finding the circumference of a circle import java.util.Scanner; public class CircumfrenceOfCircle { public static void main(String args[]){ int radius; double circumference; Scanner sc = new Scanner(System.in); System.out.println("Enter the radius of the circle ::"); radius = sc.nextInt(); circumference = Math.PI*2*radius; System.out.println("Circumference ... Read More

16K+ Views
Area of a circle is the product of the square of its radius, and the value of PI, Therefore, to calculate the area of a rectangleGet the radius of the circle.Calculate the square of the radius.Calculate the product of the value of PI and the value of the square of the radius.Print the result.Exampleimport java.util.Scanner; public class AreaOfCircle { public static void main(String args[]){ int radius; double area; Scanner sc = new Scanner(System.in); System.out.println("Enter the radius of the circle ::"); radius = sc.nextInt(); ... Read More

2K+ Views
Area of a triangle is half the product of its base and height. Therefore, to calculate the area of a triangle.Get the height of the triangle form the user.Get the base of the triangle form the user. Calculate their product and divide the result with 2.Print the final result.Exampleimport java.util.Scanner; public class AreaOfTriangle { public static void main(String args[]){ int height, base, area; Scanner sc = new Scanner(System.in); System.out.println("Enter the height of the triangle ::"); height = sc.nextInt(); System.out.println("Enter the base of the triangle ::"); ... Read More

10K+ Views
Area of a rectangle is the product of its length and breadth. Therefore, to calculate the area of a rectangleGet the length of the rectangle form the user.Get the breadth of the rectangle form the user.Calculate their product.Print the product.ExampleBelow is an example to find the area of a rectangle in Java using Scanner class.import java.util.Scanner; public class AreaOfRectangle { public static void main(String args[]){ int length, breadth, area; Scanner sc = new Scanner(System.in); System.out.println("Enter the length of the rectangle ::"); length = sc.nextInt(); ... Read More

5K+ Views
A square is a rectangle whose length and breadth are same. Therefore, Area of a rectangle is the square of its length. so, to calculate the area of a squareGet the length of the square form the user.Calculate its square.Print the square.Exampleimport java.util.Scanner; public class AreaOfSquare { public static void main(String args[]){ int length, area; Scanner sc = new Scanner(System.in); System.out.println("Enter the length of the square ::"); length = sc.nextInt(); area = length* length; System.out.println("Area of the square is ::"+area); ... Read More

2K+ Views
The interface Set does not allow duplicate elements, therefore, create a set object and try to add each element to it using the add() method in case of repetition of elements this method returns false −If you try to add all the elements of the array to a Set, it accepts only unique elements so, to find duplicate characters in a given stringConvert it into a character array.Try to insert elements of the above-created array into a hash set using add method.If the addition is successful this method returns true.Since Set doesn't allow duplicate elements this method returns 0 when ... Read More

460 Views
ASCII stands for American Standard Code for Information Interchange. There are 128 standard ASCII codes, each of which can be represented by a 7-digit binary number: 0000000 through 1111111.If you try to store a character into an integer value it stores the ASCII value of the respective character.Exampleimport java.util.Scanner; public class ASCIIValue { public static void main(String args[]){ System.out.println("Enter a character ::"); Scanner sc = new Scanner(System.in); char ch = sc.next().charAt(0); int asciiValue = ch; System.out.println("ASCII value of the given character is ::"+asciiValue); ... Read More