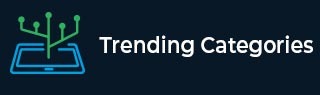
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

8K+ Views
To count the number of consonants in a given sentence: Read a sentence from the user. Create a variable (count) initialize it with 0; Compare each character in the sentence with the characters {'a', 'e', 'i', 'o', 'u' } If match doesn't occurs increment the count. Finally print count.ExampleBelow is an example to count the number of consonants in a given sentence in Javaimport java.util.Scanner; public class CountingConsonants { public static void main(String args[]){ int count = 0; System.out.println("Enter a sentence :"); Scanner sc = new Scanner(System.in); String sentence = sc.nextLine(); for (int i=0 ; i

1K+ Views
Read a character from user verify whether it lies between a and z (both small and capital). If it does it is an alphabet.Exampleimport java.util.Scanner; public class AlphabetOrNot { public static void main(String args[]){ System.out.println("Enter a character :: "); Scanner sc = new Scanner(System.in); char ch = sc.next().charAt(0); if(((ch >= 'A' && ch = 'a' && ch

449 Views
The conditional operator is also known as the ternary operator. This operator consists of three operands and is used to evaluate Boolean expressions. The goal of the operator is to decide, which value should be assigned to the variable. The operator is written as −variable x = (expression) ? value if true : value if falseExampleLive Demopublic class LargestOf3Nums_TernaryOperator { public static void main(String args[]) { int a, b, c, temp, result; a = 10; b = 20; c = 30; temp = a < b ? a:b; result = c < temp ? c:temp; System.out.println("Largest number is ::"+result); } }OutputLargest number is ::30

728 Views
The conditional operator is also known as the ternary operator. This operator consists of three operands and is used to evaluate Boolean expressions. The goal of the operator is to decide, which value should be assigned to the variable. The operator is written as −variable x = (expression) ? value if true: value if falseExampleLive Demopublic class SmallestOf3NumsUsingTernary { public static void main(String args[]) { int a, b, c, temp, result; a = 10; b = 20; c = 30; temp = a > b ? a:b; result = c > temp ? c:temp; System.out.println("Smallest number is ::"+result); } }OutputSmallest number is ::10

13K+ Views
The following program accepts average from the user, calculates the grade and prints it.Examplepublic class CalculateStudentGrades { public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.println("Enter average of your marks (less than 100)::"); int average = sc.nextInt(); char grade; if(average>=80){ grade = 'A'; }else if(average>=60 && average=40 && average

9K+ Views
The following program accepts two integer variables, takes an operator regarding the operation. According to the selected operator, the program performs the respective operation and print the result.Exampleimport java.util.Scanner; public class ab39_CalculatorUsingSwitch { public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.println("Enter value of 1st number ::"); int a = sc.nextInt(); System.out.println("Enter value of 2nd number ::"); int b = sc.nextInt(); System.out.println("Select operation"); System.out.println("Addition-a: Subtraction-s: Multiplication-m: Division-d: "); char ch ... Read More

628 Views
Stack is an Abstract Data Type (ADT), commonly used in most programming languages. It is named stack as it behaves like a real-world stack, for example – a deck of cards or a pile of plates, etc.A stack is first in first out, it has two main operations push and pop. Push inserts data into it and pop retrieves data from it.To reverse an array using stack initially push all elements into the stack using the push() method then, retrieve them back using the pop() method into another array.Exampleimport java.util.Arrays; import java.util.Stack; public class ab38_ReverseOfArray { public ... Read More

2K+ Views
A switch statement allows a variable to be tested for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each case. To verify whether given character is a vowel read a character from the user into a variable (say ch).Define a boolean bool variable and initialize it with false.Define cases for character ch with vowel characters, both capital and small ('a', 'e', 'i', 'o', 'u' ) without break statements.For all these assigns make the bool variable true.Finally, if the value of the bool variable is true given character ... Read More

4K+ Views
Comparing three integer variables is one of the simplest programs you can write at ease. Take two integer variables, say A, B& C Assign values to variables If A is greater than B & C, Display A is the largest value If B is greater than A & C, Display B is the largest value If C is greater than A & B, Display A is the largest value Otherwise, Display A, B & C are not unique valuesExampleimport java.util.Scanner; public class LargestOfThreeNumbers { public static void main(String args[]){ Scanner sc =new Scanner(System.in); System.out.println("Enter 1st number :"); ... Read More

2K+ Views
Fibonacci Series generates subsequent number by adding two previous numbers. Fibonacci series starts from two numbers − F0 & F1. The initial values of F0 & F1 can be taken 0, 1 or 1, 1 respectively.ExampleLive Demopublic class FibonacciSeriesWithWhileLoop{ public static void main(String args[]) { int a, b, c, i = 1, n; n = 10; a = b = 1; System.out.print(a+" "+b); while(i