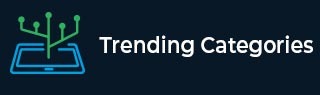
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

516 Views
The java.lang.Math.round(float a) returns the closest int to the argument. The result is rounded to an integer by adding 1/2, taking the floor of the result, and casting the result to type int. Special cases −If the argument is NaN, the result is 0.If the argument is negative infinity or any value less than or equal to the value of Integer.MIN_VALUE, the result is equal to the value of Integer.MIN_VALUE.If the argument is positive infinity or any value greater than or equal to the value of Integer.MAX_VALUE, the result is equal to the value of Integer.MAX_VALUE.Exampleimport java.util.Scanner; public class RoundingDecimalPlaces ... Read More

2K+ Views
Read integers from users using the nextInt() method of the Scanner class.To swap them −Create a variable (temp), initialize it with 0.Assign 1st number to temp.Assign 2nd number to 1st number.Assign temp to second number.Exampleimport java.util.Scanner; public class SwapTwoNumbers { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("Enter first number :: "); int num1 = sc.nextInt(); System.out.println("Enter second number :: "); int num2 = sc.nextInt(); int temp = 0; temp = num1; ... Read More

2K+ Views
Read the base and exponent values from the user. Multiply the base number by itself and multiply the resultant with base (again) repeat this n times where n is the exponent value.2 ^ 5 = 2 X 2 X 2 X 2 X 2 (5 times)Exampleimport java.util.Scanner; public class PowerOfNumber { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("Enter the base number ::"); int base = sc.nextInt(); int temp = base; System.out.println("Enter the exponent number ::"); int exp = sc.nextInt(); for (int i=1; i

1K+ Views
Following is a Java program which accepts an integer variable from user and prints the multiplication table of that particular integer.Exampleimport java.util.Scanner; public class MultiplicationTable { public static void main(String args[]) { System.out.println("Enter an integer variable :: "); Scanner sc = new Scanner(System.in); int num = sc.nextInt(); for(int i=1; i

7K+ Views
L.C.M. or Least Common Multiple of two values is the smallest positive value which the multiple of both values.For example multiples of 3 and 4 are:3 → 3, 6, 9, 12, 15 ... 4 → 4, 8, 12, 16, 20 ...The smallest multiple of both is 12, hence the LCM of 3 and 4 is 12.AlgorithmInitialize A and B with positive integers. Store maximum of A & B to the max. Check if max is divisible by A and B. If divisible, Display max as LCM. If not divisible then step increase max, go to step 3.Examplepublic class LCMOfTwoNumbers { public static void ... Read More

28K+ Views
An H.C.F or Highest Common Factor, is the largest common factor of two or more values.For example factors of 12 and 16 are −12 → 1, 2, 3, 4, 6, 12 16 → 1, 2, 4, 8, 16The common factors are 1, 2, 4 and the highest common factor is 4.AlgorithmDefine two variables - A, BSet loop from 1 to max of A, BCheck if both are completely divided by same loop number, if yes, store itDisplay the stored number is HCFExample: Using Java for loop import java.util.Scanner; public class GCDOfTwoNumbers { public static void main(String args[]){ ... Read More

15K+ Views
Read a number from user. Create an integer (count) initialize it with 0. Divide the number with 10. till the number is 0 and for each turn increment the count.Exampleimport java.util.Scanner; public class CountingDigitsInInteger { public static void main(String args[]){ Scanner sc = new Scanner(System.in); int count = 0; System.out.println("Enter a number ::"); int num = sc.nextInt(); while(num!=0){ num = num/10; count++; } System.out.println("Number of digits in the entered integer are :: "+count); } }OutputEnter a number :: 1254566 Number of digits in the entered integer are :: 7

27K+ Views
To count the number of vowels in a given sentence: Read a sentence from the user Create a variable (count) initialize it with 0; Compare each character in the sentence with the characters {'a', 'e', 'i', 'o', 'u' } If a match occurs increment the count. Finally print count. Example import java.util.Scanner; public class CountingVowels { public static void main(String args[]){ int count = 0; System.out.println("Enter a sentence :"); Scanner sc = new Scanner(System.in); String sentence = sc.nextLine(); for (int i=0 ; i

14K+ Views
An Armstrong number is a number which equals to the sum of the cubes of its individual digits. For example, 153 is an Armstrong number as −153 = (1)3 + (5)3 + (3)3 153 1 + 125 + 27 154 153Algorithm1. Take integer variable Arms. 2. Assign a value to the variable. 3. Split all digits of Arms. 4. Find cube-value of each digit. 5. Add all cube-values together. 6. Save the output to Sum variable. 7. If Sum equals to Arms print Armstrong Number. 8. If Sum does not equal to Arms print Not Armstrong Number.Example Below is an ... Read More