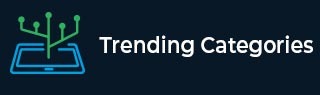
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

5K+ Views
Mean is an average value of given set of numbers. It is calculated similarly to that of the average value. Adding all given number together and then dividing them by the total number of values produces mean.For Example Mean of 3, 5, 2, 7, 3 is (3 + 5 + 2 + 7 + 3) / 5 = 4AlgorithmTake an integer set A of n values.Add all values of A together.Divide result of Step 2 by n.The result is mean of A's values.Programpublic class CaculatingMean { public static void main(String args[]){ float mean; int ... Read More

11K+ Views
An average of a set of numbers is their sum divided by their quantity. It can be defined as −average = sum of all values / number of valuesHere we shall learn how to programmatically calculate average.Algorithm1. Collect integer values in an array A of size N. 2. Add all values of A. 3. Divide the output of Step 2 with N. 4. Display the output of Step 3 as average.ExampleLive Demopublic class AverageOfNNumbers { public static void main(String args[]){ int i,total; int a[] = {0,6,9,2,7}; int n = 5; total = 0; for(i=0; i

1K+ Views
Following is an example to find the cube root of a given number.Programimport java.util.Scanner; public class FindingCubeRoot { public static void main(String args[]){ double i, precision = 0.000001; System.out.println("Enter a number ::"); Scanner sc = new Scanner(System.in); int num = sc.nextInt(); for(i = 1; (i*i*i)

12K+ Views
Cube of a value is simply three times multiplication of the value with self.For example, cube of 2 is (2*2*2) = 8.AlgorithmSteps to find a cube of a given number in Java programming:Take integer variable A.Multiply A three times.Display result as Cube.Exampleimport java.util.Scanner; public class FindingCube { public static void main(String args[]){ int n = 5; System.out.println("Enter a number ::"); Scanner sc = new Scanner(System.in); int num = sc.nextInt(); System.out.println("Cube of the given number is "+(num*num*num)); } }OutputEnter a number :: 5 Cube of the given number is 125

12K+ Views
In statistics math, a mode is a value that occurs the highest numbers of time. For Example, assume a set of values 3, 5, 2, 7, 3. The mode of this value set is 3 as it appears more than any other number.Algorithm1.Take an integer set A of n values. 2.Count the occurrence of each integer value in A. 3.Display the value with the highest occurrence.ExampleLive Demopublic class Mode { static int mode(int a[], int n) { int maxValue = 0, maxCount = 0, i, j; for (i = 0; i < ... Read More

217 Views
Permutation refers a number of ways in which set members can be arranged or ordered in some fashion. The formula of permutation of arranging k elements out of n elements is −nPk = n! / (n - k)!Algorithm1. Define values for n and r. 2. Calculate factorial of n and (n-r). 3. Divide factorial(n) by factorial(n-r). 4. Display result as a permutation.Exampleimport java.util.Scanner; public class Permutation { static int factorial(int n) { int f; for(f = 1; n > 1; n--){ f *= n; ... Read More

1K+ Views
The process of finding the square root of a number can be divided into two steps. One step is to find integer part and the second one is for fraction part.AlgorithmDefine value n to find the square root of.Define variable i and set it to 1. (For integer part)Define variable p and set it to 0.00001. (For fraction part)While i*i is less than n, increment i.Step 4 should produce the integer part so far.While i*i is less than n, add p to i.Now i have the square root value of n.ExampleLive Demopublic class SquareRoot { public static void main(String ... Read More

5K+ Views
To find the Frequency of a character in a given String Read a string from the user. Read the character. Create an integer variable initialize it with 0. Compare each character in the given string with the entered character increment the above created integer variable each time a match occurs.Exampleimport java.util.Scanner; public class FrequencyOfACharacter { public static void main(String args[]){ System.out.println("Enter a string value ::"); Scanner sc = new Scanner(System.in); String str = sc.nextLine(); System.out.println("Enter a particular character ::"); char character = sc.nextLine().charAt(0); int count = 0; for (int i=0; i

744 Views
Scanner class of the util package class is used to read data from user The nextInt() method of this class reads an integer from the user.Programimport java.util.Scanner; public class PrintInteger { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("Enter an integer:"); int num = sc.nextInt(); System.out.print("Given integer is :: "+num); } }OutputEnter an integer: 123 Given integer is :: 123

11K+ Views
Roots of a quadratic equation are determined by the following formula:$$x = \frac{-b\pm\sqrt[]{b^2-4ac}}{2a}$$Algorithm To calculate the rootsCalculate the determinant value (b*b)-(4*a*c).If determinant is greater than 0 roots are [-b +squareroot(determinant)]/2*a and [-b -squareroot(determinant)]/2*a.If determinant is equal to 0 root value is (-b+Math.sqrt(d))/(2*a)Example to find the roots of a quadratic equationBelow is an example to find the roots of a quadratic equation in Java programming.import java.util.Scanner; public class RootsOfQuadraticEquation { public static void main(String args[]){ double secondRoot = 0, firstRoot = 0; Scanner sc = new Scanner(System.in); System.out.println("Enter the value of ... Read More