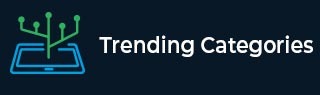
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

262 Views
We can convert any decimal number to its equivalent octal by following program.In this we reserve the reminder we get after divide the given number by 8 as it is the base of Octal and then reverse the order of reminders we have stored by multiplying each reminder by 10.Let understand by following example.Example Live Demopublic class DecimalToOctal { public static void main(String[] args) { int decimal = 84; int octalNumber = 0, i = 1; while (decimal != 0) { octalNumber += (decimal % 8) * i; decimal /= 8; i *= 10; } System.out.println("Octal of given decimal is " + octalNumber); } }OutputOctal of given decimal is 124

529 Views
In order to convert first character as upper case in a sentence we have to first separate each word of sentence and then make first character of each word in upper case.After which we have to again join each word separated by space to make again sentence.Now let take each task one by one.First in order to get each word of the sentence separately we would use Scanner class of Java and implement its hasnext method to check whether our sentence has any more word or not, in case word is present then we would get that word by using ... Read More

313 Views
In order to find that a given string contains all vowels we have to first convert given string into character array so that we can simplify the comparison of each character of given string.After this put each character into a hash map so that we can check whether our map created from given string contain all vowels or not.We took help of hash map here because there is no concrete method in character array class which could check that it contains all vowels or not.Only way to check is to iterate whole array and compare each character with each vowel ... Read More

618 Views
In Java there is no delegate library or API in order to write or read comma separated value(csv) file.So a 3rd party APIs are available for such requirements.Most popular 3rd party API is OpenCSV which is provide methods to deal with CSV file such as read csv file, write csv file, parse values of csv file, mapping of csv file values to java beans and java beans to csv file etc.In order to use/import this tool in Java project there are following approaches −Download the binaries/jars from http://sourceforge.net/projects/opencsv/Download through maven by updating pom.xml as net.sf.opencsv opencsv 2.3 ... Read More

183 Views
strictfp is used to ensure that floating points operations give the same result on any platform. As floating points precision may vary from one platform to another. strictfp keyword ensures the consistency across the platforms.strictfp can be applied to class, method or on interfaces but cannot be applied to abstract methods, variable or on constructors. Following are the valid examples of strictfp usage.Examplestrictfp class Test { } strictfp interface Test { } class A { strictfp void Test() { } }ExampleFollowing are the invalid strictfp usage.class A { strictfp float a; } class A { strictfp abstract void test(); } class A { strictfp A() { } }

175 Views
The Static ModifierStatic VariablesThe static keyword is used to create variables that will exist independently of any instances created for the class. Only one copy of the static variable exists regardless of the number of instances of the class.Static variables are also known as class variables. Local variables cannot be declared static.Static MethodsThe static keyword is used to create methods that will exist independently of any instances created for the class.Static methods do not use any instance variables of any object of the class they are defined in. Static methods take all the data from parameters and compute something from ... Read More

1K+ Views
As we know that Hash map in Java does not maintain insertion order either by key or by order.Also it does not maintain any other order while adding entries to it.But Java provide another API named as TreeMap which maintain its insertion order sorted according to the natural ordering of its keys.So in order to sort our given Hash map according to keys we would insert our map into a tree map which by default insert according to key sorting.After insertion we would transverse same tree map which is sorted and is our resultant sorted map.Example Live Demoimport java.util.HashMap; import java.util.TreeMap; ... Read More

2K+ Views
In java inheritance some of the basic rules includes −Object relation of Superclass (parent) to Subclass (child) exists while child to parent object relation never exists.This means that reference of parent class could hold the child object while child reference could not hold the parent object.In case of overriding of non static method the runtime object would evaluate that which method would be executed of subclass or of superclass.While execution of static method depends on the type of reference that object holds.Other basic rule of inheritance is related to static and non static method overriding that static method in java ... Read More

3K+ Views
Java provides two classes for having random numbers generation - SecureRandom.java and Random.java.The random numbers can be used generally for encryption key or session key or simply password on web server.SecureRandom is under java.security package while Random.java comes under java.util package.The basic and important difference between both is SecureRandom generate more non predictable random numbers as it implements Cryptographically Secure Pseudo-Random Number Generator (CSPRNG) as compare to Random class which uses Linear Congruential Generator (LCG).A important point to mention here is SecureRandom is subclass of Random class and inherits its all method such as nextBoolean(), nextDouble(), nextFloat(), nextGaussian(), nextInt() and ... Read More

1K+ Views
To find unique words in a string use Map utility of java because of its property that it does not contain duplicate keys.In order to find unique words first get all words in array so that compare each word, for this split string on the basis of space/s.If other characters such as comma(, ) or fullstop (.) are present then using required regex first replace these characters from the string.Insert each word of string as key of Map and provide initial value corresponding to each key as 'is unique' if this word does not inserted in map before.Now when a ... Read More