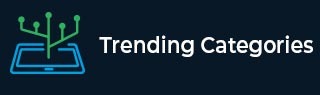
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

502 Views
HashMap, TreeMap and LinkedHashMap all implements java.util.Map interface and following are their characteristics.HashMapHashMap has complexity of O(1) for insertion and lookup.HashMap allows one null key and multiple null values.HashMap does not maintain any order.TreeMapTreeMap has complexity of O(logN) for insertion and lookup.TreeMap does not allow null key but allow multiple null values.TreeMap maintains order. It stores keys in sorted and ascending order.LinkedHashMapLinkedHashMap has complexity of O(1) for insertion and lookup.LinkedHashMap allows one null key and multiple null values.LinkedHashMap maintains order in which key-value pairs are inserted.Example Live Demoimport java.util.HashMap; import java.util.Hashtable; import java.util.LinkedHashMap; import java.util.Map; import java.util.TreeMap; public class Tester { ... Read More

4K+ Views
A helper class serve the following purposes.Provides common methods which are required by multiple classes in the project.Helper methods are generally public and static so that these can be invoked independently.Each methods of a helper class should work independent of other methods of same class.Following example showcases one such helper class.Examplepublic class Tester { public static void main(String[] args) { int a = 37; int b = 39; System.out.println(a + " is prime: " + Helper.isPrime(a)); System.out.println(b + " is prime: " + Helper.isPrime(b)); } } ... Read More

222 Views
An immutable class object's properties cannot be modified after initialization. For example String is an immutable class in Java. We can create a immutable class by following the given rules below.Make class final − class should be final so that it cannot be extended.Make each field final − Each field should be final so that they cannot be modified after initialization.Create getter method for each field. − Create a public getter method for each field. fields should be private.No setter method for each field. − Do not create a public setter method for any of the field.Create a parametrized constructor ... Read More

2K+ Views
In order to find the count of matching characters in two Java strings the approach is to first create character arrays of both the strings which make comparison simple.After this put each unique character into a Hash map.Compare each character of other string with created hash map whether it is present or not in case if present than put that character into other hash map this is to prevent duplicates.In last get the size of this new created target hash map which is equal to the count of number of matching characters in two given strings.Example Live Demoimport java.util.HashMap; public class ... Read More

169 Views
Class declarationpublic class CopyOnWriteArrayList extends Object implements List, RandomAccess, Cloneable, SerializableCopyOnWriteArrayList is a thread-safe variant of Arraylist where operations which can change the arraylist (add, update, set methods) creates a clone of the underlying array.CopyOnWriteArrayList is to be used in Thread based environment where read operations are very frequent and update operations are rare.Iterator of CopyOnWriteArrayList will never throw ConcurrentModificationException.Any type of modification to CopyOnWriteArrayList will not reflect during iteration since the iterator was created.List modification methods like remove, set and add are not supported in iteration. These method will throw UnsupportedOperationException.null can be added to the list.CopyOnWriteArrayList MethodsFollowing is ... Read More

272 Views
We can compare two strings lexicographically using following ways in Java.Using String.compareTo(String) method. It compares in case sensitive manner.Using String.compareToIgnoreCase(String) method. It compares in case insensitive manner.Using String.compareTo(Object) method. It compares in case sensitive manner.These methods returns the ascii difference of first odd characters of compared strings.Example Live Demopublic class Tester { public static void main(String args[]) { String str = "Hello World"; String anotherString = "hello world"; Object objStr = str; System.out.println( str.compareTo(anotherString) ); System.out.println( str.compareToIgnoreCase(anotherString) ); System.out.println( str.compareTo(objStr.toString())); } }Output-32 0 0

1K+ Views
TestedThe benefit of overriding is ability to define a behavior that's specific to the subclass type, which means a subclass can implement a parent class method based on its requirement.In object-oriented terms, overriding means to override the functionality of an existing method.Example Live Democlass Animal { public void move() { System.out.println("Animals can move"); } } class Dog extends Animal { public void move() { System.out.println("Dogs can walk and run"); } } public class TestDog { public static void main(String args[]) { Animal a = new Animal(); // ... Read More

228 Views
Method overloading is a type of static polymorphism. In Method overloading, we can define multiple methods with the same name but with different parameters. Consider the following example program.Example Live Demopublic class Tester { public static void main(String args[]) { Tester tester = new Tester(); System.out.println(tester.add(1, 2)); System.out.println(tester.add(1, 2, 3)); } public int add(int a, int b) { return a + b; } public int add(int a, int b, int c) { return a + b + c; } }Output3 ... Read More

2K+ Views
In Serialization when inheritance is introduced then on the basis of superclass and subclass certain cases have been defined which make the understanding of Serialization in each case much simpler. The fundamental rules which should be followed are as below.1. When super class is implements Serializable interface and subclass is not.In this case the object of subclass get serialized by default when superclass get serialize, even if subclass doesn't implements Serializable interface.Examplepublic class TestSerialization { public static void main(String[] args) throws IOException, ClassNotFoundException { B obj = new B(); FileOutputStream fos = new ... Read More

2K+ Views
In java constructor is something which is responsible for object creation of a particular class.Along with other functions of constructor it also instantiate the properties/instances of its class.In java by default super() method is used as first line of constructor of every class, here the purpose of this method is to invoke constructor of its parent class so that the properties of its parent get well instantiated before subclass inherits them and use.The point that should remember here is when you create a object the constructor is called but it is not mandatory that whenever you called a constructor of ... Read More