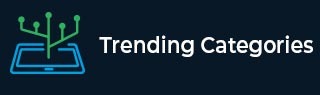
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

5K+ Views
To convert decimal to hexadecimal, use any of the two methods i.e.Integer.toHexString() − It returns a string representation of the integer argument as an unsigned integer in base 16.Integer.parseInt() − It allows you to set the radix as well, for example, for hexadecimal set it as 16.Let us see an example now to convert decimal to hexadecimal using Integer.toHexString() method.Example Live Demopublic class Demo { public static void main( String args[] ) { int dec = 158; System.out.println(Integer.toHexString(dec)); } }Output9eLet us see an example now to convert decimal to hexadecimal using Integer.parseInt() method.Example Live ... Read More

279 Views
Use the toHexString() method to convert decimal to hexadecimal. The method returns a string representation of the integer argument as an unsigned integer in base 16. The following characters are used as hexadecimal digits:0123456789abcdef.The following is the syntax.String toHexString(int i)It has only single parameter.i − This is an integer to be converted to a string.Example Live Demopublic class Demo { public static void main( String args[] ) { int dec = 45; System.out.println("Decimal = "+dec); // converting to hex System.out.println(Integer.toHexString(dec)); } }OutputDecimal = 45 2d

651 Views
To check for Integer overflow, we need to check the Integer.MAX_VALUE with the added integers result, Here, Integer.MAX_VALUE is the maximum value of an integer in Java.Let us see an example wherein integers are added and if the sum is more than the Integer.MAX_VALUE, then an exception is thrown.Example Live Demopublic class Demo { public static void main(String[] args) { int a = 9897988; int b = 8798798; System.out.println("Value1: "+a); System.out.println("Value2: "+b); long sum = (long)a + (long)b; if (sum > Integer.MAX_VALUE) ... Read More

222 Views
Use the parseInt() method with the second parameter as 8 since it is the radix value. The parseInt() method has the following two forms.static int parseInt(String s) static int parseInt(String s, int radix)To convert octal to decimal, use the 2nd syntax and add radix as 8, since octal radix is 8.Integer.parseInt("25", 8)The following is an example.Example Live Demopublic class Demo { public static void main( String args[] ) { // converting to decimal System.out.println(Integer.parseInt("25", 8)); } }Output21

297 Views
Use the parseInt() method with the second parameter as 16 since it is the radix value. The parseInt() method has the following two forms.static int parseInt(String s) static int parseInt(String s, int radix)To convert hex string to decimal, use the 2nd syntax and add radix as 16, since hexadecimal radix is 16.Integer.parseInt("12", 16)Example Live Demopublic class Demo { public static void main( String args[] ) { // converting to decimal System.out.println(Integer.parseInt("444", 16)); } }Output1092

24K+ Views
To concatenate a string to an int value, use the concatenation operator.Here is our int.int val = 3;Now, to concatenate a string, you need to declare a string and use the + operator.String str = "Demo" + val;Let us now see another example.Example Live Demoimport java.util.Random; public class Demo { public static void main( String args[] ) { int val = 3; String str = "" + val; System.out.println(str + " = Rank "); } }Output3 = Rank

287 Views
To convert a String to int in Java, we can use the two built-in methods namely parseInt() and valueOf(). These static methods belong to the Integer class of java.lang package and throws a NumberFormatException if the string is not a valid representation of an integer. In Java, String is a class of 'java.lang' package that stores a series of characters enclosed within double quotes. And, an integer is a primitive datatype that stores numerical values. In this article, we will discuss a few Java programs that illustrate how to convert a given String to integer. Java Program to Convert a ... Read More

10K+ Views
To check two numbers for equality in Java, we can use the Equals() method as well as the == operator.Firstly, let us set Integers.Integer val1 = new Integer(5); Integer val2 = new Integer(5);Now, to check whether they are equal or not, let us use the == operator.(val1 == val2)Let us now see the complete example.Example Live Demopublic class Demo { public static void main( String args[] ) { Integer val1 = new Integer(5); Integer val2 = new Integer(5); Integer val3 = new Integer(10); System.out.println("Integer 1 = "+val1); ... Read More

7K+ Views
The Equals() method compares this object to the specified object. The result is true if and only if the argument is not null and is an Integer object that contains the same int value as this object.Let us first set Integer object.Integer val1 = new Integer(30); Integer val2 = new Integer(60); Integer val3 = new Integer(55); Integer val4 = new Integer(30);Now let us check their equality using the Equals() method.val1.equals(val2);In the same way, check it for different Integers.Let us see the complete example.Example Live Demoimport java.util.Random; public class Demo { public static void main( String args[] ) { ... Read More

2K+ Views
To generate random numbers in Java, use.import java.util.Random;Now, take Random class and create an object.Random num = new Random();Now, in a loop, use the nextInt() method since it is used to get the next random integer value. You can also set a range, like for 0 to 20, write it as.nextInt( 20 );Let us see the complete example wherein the range is 1 to 10.Example Live Demoimport java.util.Random; public class Demo { public static void main( String args[] ) { Random num = new Random(); int res; for ( int i = 1; i