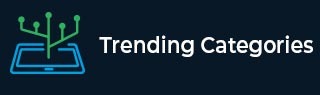
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

216 Views
To parse and format to hexadecimal in Java, we have used the BigInteger class. The java.math.BigInteger class provides operations analogues to all of Java's primitive integer operators and for all relevant methods from java.lang.Math.In the below example, we have taken BigInteger and created an object. With that, some values have been set by us in the arguments as the hexadecimal and the radix i.e. 16 for hexadecimal.BigInteger bi = new BigInteger("2ef", 16);Let us see an example.Example Live Demoimport java.math.*; public class Demo { public static void main( String args[] ) { BigInteger bi = new BigInteger("2ef", 16); ... Read More

308 Views
To parse and format a number to decimal, try the following code.Example Live Demopublic class Demo { public static void main( String args[] ) { int val = Integer.parseInt("5"); String str = Integer.toString(val); System.out.println(str); } }Output5In the above program, we have used the Integer.parseInt() and Integer.toString() method to convert a number to decimal.int val = Integer.parseInt("5"); String str = Integer.toString(val);The toString() method above represents a value in string and formats.

365 Views
Every Java primitive data type has a class dedicated to it. These classes wrap the primitive data type into an object of that class. Therefore, it is known as wrapper classes.The following is the program that displays a Primitive DataType in a Wrapper Object.Example Live Demopublic class Demo { public static void main(String[] args) { Boolean myBoolean = new Boolean(true); boolean val1 = myBoolean.booleanValue(); System.out.println(val1); Character myChar = new Character('a'); char val2 = myChar.charValue(); System.out.println(val2); Short myShort ... Read More

176 Views
Underflow occurs when the given value is less than the maximum prescribed size of a data type. The underflow condition can result to an error or now the implementation of the programming language handles it on its own.To display underflow of datatypes, I have taken an example of double datatype. Double data type is a single-precision 64-bit IEEE 754 floating point.The following program display underflow of datatype in Java.Example Live Demopublic class Demo { public static void main(String[] args) { System.out.println("Displaying Underflow... "); double val1 = 3.2187E-320; System.out.println(val1/1000000); } }OutputDisplaying ... Read More

518 Views
To parse and format a number to octal, try the following code −Example Live Demopublic class Demo { public static void main( String args[] ) { int val = Integer.parseInt("150", 8); System.out.println(val); String str = Integer.toString(val, 8); System.out.println(str); } }Output104 150In the above program, we have used the Integer.parseInt() and Integer.toString() method to convert a number to octal.int val = Integer.parseInt("150", 8); String str = Integer.toString(val, 8);The toString() method above represents a value in string and formats.We have set the radix as 8, since octal is represented by radix 8.

154 Views
To convert an Integer to a boolean, we have taken the following Integer objects.Integer one = 1; Integer two = 1; Integer three = 0;We have taken nested if-else statement to display the true or false values. Here, the value “one” is the same as “two” i.e. 1; therefore, the following else-if works.else if (one.equals(two)) { System.out.println(true); }The above display “true” and in this way we converted Integer to boolean.Let us now see the complete example to learn how to convert an Integer to Boolean.Example Live Demopublic class Demo { public static void main(String[] args) { ... Read More

238 Views
Use the parseBoolean() method to create a boolean variable from a string. Here for boolean variable, firstly take a boolean and pass the string to it using Boolean.parseBoolean().boolean val1 = Boolean.parseBoolean("TRUE");The parseBoolean() parses the string argument as a boolean. The boolean returned represents the value true if the string argument is not null and is equal, ignoring case, to the string "true".Do the same for FALSE as well.boolean val2 = Boolean.parseBoolean("FALSE");Let us now see the complete example to form a boolean variable from string.Example Live Demopublic class Demo { public static void main(String[] args) { boolean val1 ... Read More

3K+ Views
Use the valueOf() method to convert boolean value to Boolean. Firstly, let us take a boolean value.boolean val = false;Now, to convert it to Boolean object, use the valueOf() method.Boolean res = Boolean.valueOf(val);Let us now see the complete example to convert boolean value to Boolean.Example Live Demopublic class Demo { public static void main(String[] args) { boolean val = false; System.out.println("Boolean Value = "+val); Boolean res = Boolean.valueOf(val); System.out.println("Boolean = " + res); if (res.equals(Boolean.TRUE)) { System.out.println("Boolean = " + res); ... Read More

12K+ Views
To convert boolean to integer, let us first declare a variable of boolean primitive. boolean bool = true; Now, to convert it to integer, let us now take an integer variable and return a value “1” for “true” and “0” for “false”. int val = (bool) ? 1 : 0; Let us now see the complete example to convert boolean to integer in Java. Example public class Demo { public static void main(String[] args) { // boolean boolean bool = true; System.out.println("Boolean Value: "+bool); int val = (bool) ? 1 : 0; // Integer System.out.println("Integer value: "+val); } }OutputBoolean Value: true Integer value: 1

1K+ Views
To convert a string to a number in Java, use the Integer.parseInt() method. First, let use create a String and set value.String str = "45";Now, take an Integer and use the Integer.parseInt() method.Integer i = Integer.parseInt(str);Let us see the complete example.Example Live Demopublic class Demo { public static void main( String args[] ) { String str = "45"; Integer i = Integer.parseInt(str); System.out.println("Num: " + i); } }OutputNum: 45