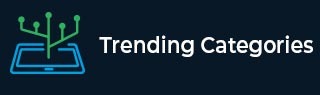
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

687 Views
To determine if a String is a legal Java Identifier, use the Character.isJavaIdentifierPart() and Character.isJavaIdentifierStart() methods.Character.isJavaIdentifierPart()The java.lang.Character.isJavaIdentifierPart() determines if the character (Unicode code point) may be part of a Java identifier as other than the first character.A character may be part of a Java identifier if any of the following are true.it is a letterit is a currency symbol (such as '$')it is a connecting punctuation character (such as '_')it is a digitit is a numeric letter (such as a Roman numeral character)Character.isJavaIdentifierStart()The java.lang.Character.isJavaIdentifierStart() determines if the character (Unicode code point) is permissible as the first character in a Java ... Read More

268 Views
To compare two Java char arrays, use the Arrays.equals() method.Let us first declare and initialize some char arrays.char[] arr1 = new char[] { 'p', 'q', 'r' }; char[] arr2 = new char[] { 'p', 'r', 's' }; char[] arr3 = new char[] { 'p', 'q', 'r' };Now let us compare any two of the above arrays.Arrays.equals(arr1, arr2));In the same way, work it for other arrays and compare them.The following is an example.Example Live Demoimport java.util.*; public class Demo { public static void main(String []args) { char[] arr1 = new char[] { 'p', 'q', 'r' }; ... Read More

3K+ Views
The following is our string.String str = "Tutorial";Now, use the toCharArray() method to convert string to char array.char[] ch = str.toCharArray();Now let us see the complete example.Example Live Demopublic class Demo { public static void main(String []args) { String str = "Tutorial"; System.out.println("String: "+str); char[] ch = str.toCharArray(); System.out.println("Character Array..."); for (int i = 0; i < ch.length; i++) { System.out.print(ch[i]+" "); } } }OutputString: Tutorial Character Array... T u t o r i a l

308 Views
To determine a Character’s Unicode Block, use the Character.UnicodeBlock.of() method in Java. The method returns the object representing the Unicode block containing the given character, or null if the character is not a member of a defined block.Let us see the syntax of Character.UnicodeBlock.of() method.Character.UnicodeBlock of(char c)Here, c is the character.The following is an example that shows how we can represent the Unicode block containing the given character.Example Live Demopublic class Demo { public static void main(String []args) { char ch = '\u5639'; System.out.println(ch); Character.UnicodeBlock block = Character.UnicodeBlock.of(ch); ... Read More

1K+ Views
To store Unicode in a char variable, simply create a char variable.char c;Now assign unicode.char c = '\u00AB';The following is the complete example that shows what will get displayed: when Unicode is stored in a char variable and displayed.Example Live Demopublic class Demo { public static void main(String []args) { int a = 79; System.out.println(a); char b = (char) a; System.out.println(b); char c = '\u00AB'; System.out.println(c); } }Output79 O «

15K+ Views
Use the valueOf() method in Java to copy char array to string. You can also use the copyValueOf() method, which represents the character sequence in the array specified. Here, you can specify the part of array to be copied.Let us first create a character array.char[] arr = { 'p', 'q', 'r', 's' };The method valueOf() will convert the entire array into a string.String str = String.valueOf(arr);The following is an example.Example Live Demopublic class Demo { public static void main(String []args) { char[] arr = { 'p', 'q', 'r', 's' }; String str = String.valueOf(arr); ... Read More

14K+ Views
To convert ASCII to string, use the toString() method. Using this method will return the associated character.Let’s say we have the following int value, which works as ASCII for us.int asciiVal = 89;Now, use the toString() method.String str = new Character((char) asciiVal).toString();Example Live Demopublic class Demo { public static void main(String []args) { int asciiVal = 87; String str = new Character((char) asciiVal).toString(); System.out.println(str); } }OutputW

776 Views
To check whether the entered character is a digit, whitespace, lowercase or uppercase, you need to check for the ASCII values.Let’s say we have a value in variable “val”, which is to be checked.For Lower Case.if(val >= 97 && val = 65 && val = 48 && val = 97 && val = 65 && val = 48 && val = 97 && val = 65 && val = 48 && val

3K+ Views
To check whether the entered value is whitespace or not in Java, use the Character.isWhitespace() method.We have a value to be checked.char val = ' ';Now let us use the Character.isWhitespace() method.if (Character.isWhitespace(val)) { System.out.println("Value is a Whitespace!"); } else { System.out.println("Value is not a Whitespace"); }Let us see the complete example now to check whether the entered value is whitespace or not in Java.Example Live Demopublic class Demo { public static void main(String []args) { char val =' '; System.out.println("Value: "+val); if (Character.isWhitespace(val)) { ... Read More

103 Views
The isLetterOrDigit() method in Java returns TRUE if the entered value is a letter or digit.We have the following character.char val ='P';Now, let us check for letter or digit using if-else decision-making statement.if (Character.isLetterOrDigit(val)) { System.out.println("Value is a letter or digit!"); } else { System.out.println("Value is neither a letter nor a digit!"); }The following is an example.Example Live Demopublic class Demo { public static void main(String []args) { char val ='P'; System.out.println("Value: "+val); if (Character.isLetterOrDigit(val)) { System.out.println("Value is a letter or digit!"); ... Read More