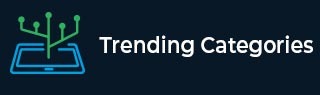
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

664 Views
The total bits in a number can be counted by using its binary representation. An example of this is given as follows − Number = 9 Binary representation = 1001 Total bits = 4 A program that demonstrates this is given as follows. Example Live Demo public class Example { public static void main(String[] arg) { int num = 10; int n = num; int count = 0; while ... Read More

6K+ Views
Each date of birth corresponds to a given Zodiac sign. A table that demonstrates these signs and their corresponding dates is given below −Zodiac SignDateAriesMarch 21 - April 19TaurusApril 20 - May 20GeminiMay 21 - June 20CancerJune 21 - July 22LeoJuly 23 - August 22VirgoAugust 23 - September 22LibraSeptember 23 - October 22ScorpioOctober 23 - November 21SagittariusNovember 22 - December 21CapricornDecember 22 - January 19AquariusJanuary 20 - February 18PiscesFebruary 19 - March 20A program that displays the Astrological sign or Zodiac sign for a given date of birth is given as follows.Example Live Demopublic class Example { public static void ... Read More

3K+ Views
Literals with a leading zero are octal literals. Any number prefixed with a 0 is considered octal. Octal numbers can only use digits 0-7, just like decimal can use 0-9, and binary can use 0-1. To define integer literals as octal value in Java is effortless. Here is the declaration and initialization. int myOct = 023; Example Live Demo public class Demo { public static void main(String []args) { int myOct = 023; System.out.println(myOct); } } Output 19 Let us see another example. Example Live Demo public class Demo { public static void main(String []args) { int myOct = 010; System.out.println(myOct); } } Output 8

866 Views
Hexadecimal literal of type long is represented as − long hexLong = 0XABL; For Hexadecimal, the 0x or 0X is to be placed in the beginning of a number. Note − Digits 10 to 15 are represented by a to f (A to F) in Hexadecimal Example Live Demo public class Demo { public static void main(String []args) { long hexLong = 0XABL; System.out.println("Hexadecimal literal of type long: "+hexLong); } } Output Hexadecimal literal of type long:171

5K+ Views
For Hexadecimal, the 0x or 0X is to be placed in the beginning of a number. Note − Digits 10 to 15 are represented by a to f (A to F) in Hexadecimal Here are some of the examples of hexadecimal integer literal declared and initialized as int. int one = 0X123; int two = 0xABC; Example Live Demo public class Demo { public static void main(String []args) { int one = 0X123; int two = 0xABC; System.out.println("Hexadecimal: "+one); System.out.println("Hexadecimal: "+two); } } Output Hexadecimal: 291 Hexadecimal: 2748

354 Views
The System.out.format is used in Java to format output. Here, let’s say the following is our long. long val = 787890; To format, we have considered the following that justifies the output. System.out.format("%d%n", val); System.out.format("%9d%n", val); System.out.format("%+9d%n", val); System.out.format("%08d%n", val); System.out.format("%, 9d%n", val); The following is the complete example that displays the difference in output as well. Example Live Demo import java.util.Locale; public class Demo { public static void main(String []args) { long val = 787890; System.out.format("%d%n", val); ... Read More

410 Views
To compare two Java long arrays in Java, use Arrays.equals() method. Let’s say we have the following long arrays. long[] arr1 = new long[] { 767, 568, 555, 897, 678 }; long[] arr2 = new long[] { 456, 756, 555, 999, 678}; long[] arr3 = new long[] { 767, 568, 555, 897, 678 }; Now, we can compare the equality of these arrays using the equals() method. Arrays.equals(arr1, arr2); Arrays.equals(arr2, arr3); Arrays.equals(arr1, arr3); The following is the complete example. Example Live Demo import java.util.*; public class Demo { public ... Read More

1K+ Views
To sort long array in Java, use the Arrays.sort() method. Let’s say the following is our long array. long[] arr = new long[] { 987, 76, 5646, 96, 8768, 8767 }; To sort the above long array is an easy task in Java. Use the following method. Arrays.sort(arr); After that, print the array and you can see that it is sorted. for (long l : arr) { System.out.println(l); } The following is the complete example. Example Live Demo import java.util.*; public class Demo { public static ... Read More

2K+ Views
The intersection of the two arrays results in those elements that are contained in both of them. If an element is only in one of the arrays, it is not available in the intersection. An example of this is given as follows − Array 1 = 1 2 5 8 9 Array 2 = 2 4 5 9 Intersection = 2 5 9 A program that demonstrates the intersection of two sorted arrays in Java is given as follows. Example Live Demo public class Example { public static void main(String args[]) ... Read More

3K+ Views
A palindrome is a sequence that is same both forwards and backwards. The binary representation of a number is checked for being a palindrome but no leading 0’s are considered. An example of this is given as follows − Number = 5 Binary representation = 101 The binary representation of 5 is a palindrome as it is the same both forwards and backwards. A program that demonstrates this is given as follows. Example Live Demo public class Example { public static void main(String argc[]) { long num ... Read More