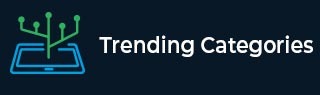
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

7K+ Views
A pangram is a string that contains all the letters of the English alphabet. An example of a pangram is "The quick brown fox jumps over the lazy dog". Problem Statement Given a string, write a Java program to check whether it is pangram or not.Consider the following example - Input The quick brown fox jumps over the lazy dog. Output String: The quick brown fox jumps over the lazy dog. The above string is a pangram. Algorithm The algorithm below is focusing to check if the string is pangram. Step 1: Create a boolean array `alphaList` ... Read More

1K+ Views
A ternary operator uses 3 operands and it can be used to replace the if else statement. This can be done to make the code simpler and more compact.The syntax of the ternary operator is given as follows −Expression ? Statement 1 : Statement 2In the above syntax, the expression is a conditional expression that results in true or false. If the value of the expression is true, then statement 1 is executed otherwise statement 2 is executed.A program that demonstrates the ternary operator in Java is given as follows.Example Live Demopublic class Example { public static void main(String[] args) ... Read More

205 Views
A diamond shape can be printed by printing a triangle and then an inverted triangle. An example of this is given as follows −* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *A program that demonstrates this is given as follows.Example Live Demopublic class Example { public static void main(String[] args) { int n = 6; int s = n - 1; ... Read More

17K+ Views
A basic calculator is able to add, subtract, multiply or divide two numbers. This is done using a switch case. A program that demonstrates this is given as follows −Exampleimport java.util.Scanner; public class Calculator { public static void main(String[] args) { double num1; double num2; double ans; char op; Scanner reader = new Scanner(System.in); System.out.print("Enter two numbers: "); num1 = reader.nextDouble(); num2 = reader.nextDouble(); System.out.print("Enter an operator (+, -, *, /): "); ... Read More

938 Views
The common elements in three sorted arrays are the elements that occur in all three of them. An example of this is given as follows −Array1 = 1 3 5 7 9 Array2 = 2 3 6 7 9 Array3 = 1 2 3 4 5 6 7 8 9 Common elements = 3 7 9A program that demonstrates this is given as follows −Examplepublic class Example { public static void main(String args[]) { int arr1[] = {1, 4, 25, 55, 78, 99}; int arr2[] = {2, 3, 4, 34, 55, 68, 75, 78, 100}; int arr3[] = {4, 55, ... Read More

11K+ Views
The number of times a word occurs in a string denotes its occurrence count. An example of this is given as follows −String = An apple is red in colour. Word = red The word red occurs 1 time in the above string.A program that demonstrates this is given as follows.Example Live Demopublic class Example { public static void main(String args[]) { String string = "Spring is beautiful but so is winter"; String word = "is"; String temp[] = string.split(" "); int count = 0; for (int i = 0; i < temp.length; i++) { if (word.equals(temp[i])) count++; } System.out.println("The string ... Read More

394 Views
Extraction of k bits from the given position in a number involves converting the number into its binary representation. An example of this is given as follows −Number = 20 Binary representation = 10100 k = 3 Position = 2 The bits extracted are 010 which represent 2.A program that demonstrates this is given as follows.Example Live Demopublic class Example { public static void main (String[] args) { int number = 20, k = 3, pos = 2; int exNum = ((1 > (pos - 1)); System.out.println("Extract " + k + ... Read More

1K+ Views
Two matrices are identical if their number of rows and columns are equal and the corresponding elements are also equal. An example of this is given as follows.Matrix A = 1 2 3 4 5 6 7 8 9 Matrix B = 1 2 3 4 5 6 7 8 9 The matrices A and B are identicalA program that checks if two matrices are identical is given as follows.Example Live Demopublic class Example { public static void main (String[] args) { int A[][] = { {7, 9, 2}, {3, 8, 6}, {1, 4, 2} }; ... Read More

12K+ Views
A string is a class in Java that stores a series of characters enclosed within double quotes. Those characters act as String-type objects. The aim of this article is to write Java programs that count words in a given string. Counting words of the given strings can be solved using the string manipulation techniques. In Java interviews, questions from string manipulation are very frequent, hence, it is important to understand this problem properly. Java Program to Count Words in a given String Before jumping to the example programs, let's understand the problem statement with the help of an example. Input ... Read More

5K+ Views
Two variables can be swapped in one line in Java. This is done by using the given statement. x = x ^ y ^ (y = x); where x and y are the 2 variables. A program that demonstrates this is given as follows − Example Live Demo public class Example { public static void main (String[] args) { int x = 12, y = 25; System.out.println("Original values of x and y"); System.out.println("x = " ... Read More