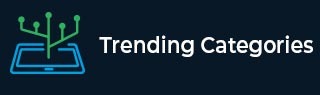
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

476 Views
To display the day in week, use the SimpleDateFormat(“E”) as shown below −Format f = new SimpleDateFormat("E"); String strDayinWeek = f.format(new Date()); System.out.println("Day in week = "+strDayinWeek);Since, we have used the Format and SimpleDateFormat class above, therefore import the following packages. With that, we have also used the Date.import java.text.Format; import java.text.SimpleDateFormat; import java.util.Date;Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); SimpleDateFormat simpleformat = new SimpleDateFormat("dd/MMMM/yyyy hh:mm:s"); ... Read More

881 Views
To display two-digit day number, use the SimpleDateFormat('dd') as shown below −// displaying two-digit day number f = new SimpleDateFormat("dd"); String strDay = f.format(new Date()); System.out.println("Day Number = "+strDay);Since, we have used the Format and SimpleDateFormat class above, therefore import the following packages. With that, we have also used the Date.import java.text.Format; import java.text.SimpleDateFormat; import java.util.Date;The following is an example.Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); ... Read More

2K+ Views
In Java, verifying whether a string is a valid number is a common requirement in various applications. This process ensures that the string can be safely converted to a numerical type without causing runtime exceptions. To validate if a string can be converted to these types, we use the Integer.parseInt() and Float.parseFloat() methods, respectively. Problem Statement Given a string that may contain an integer or a float value, write a Java program to check whether it is valid number or not. Input "100" //string value Output 100 // ... Read More

2K+ Views
Float is a wrapper class provided to wrap float primitive value.Let us create a Float object with float primitive.// float primitive float myFloat = 24.22f; // Float object Float obj1 = new Float(myFloat);Let us now create a Float object from string.Float obj2 = new Float("39.87");The following is an example that displays both the ways discussed above with output.Example Live Demoimport java.lang.*; public class Demo { public static void main(String args[]) { float myFloat = 24.22f; Float obj1 = new Float(myFloat); System.out.println(obj1); Float obj2 = new Float("39.87"); System.out.println(obj2); } }Output24.22 39.87

126 Views
The isNan() method returns true if the Float value is a Not-a-Number (NaN). Let’s say we have the following Float values.Float f1 = new Float(5.0/0.0); Float f2 = new Float(10.2/0.0); Float f3 = new Float(0.0/0.0);Check with isNaN() method.f1.isNaN (); f2.isNaN (); f3.isNaN ();The following is the complete example with output.Example Live Demoimport java.lang.*; public class Demo { public static void main(String args[]) { Float f1 = new Float(5.0/0.0); Float f2 = new Float(10.2/0.0); Float f3 = new Float(0.0/0.0); System.out.println(f1.isNaN()); System.out.println(f2.isNaN()); System.out.println(f3.isNaN()); ... Read More

258 Views
To convert decimal integer to hexadecimal, use the Integer.toHexString() method. Let’s say the following is our decimal integer.int decInt = 25;The following is the usage of the Integer.toHexString() method to convert decimal integer to hexadecimal number.String myHex = Integer.toHexString(decInt);The following the complete example.Example Live Demopublic class Demo { public static void main(String []args) { int decInt = 25; System.out.println("Decimal Integer = "+decInt); String myHex = Integer.toHexString(decInt); System.out.println("Hexadecimal = "+myHex); } }OutputDecimal Integer = 25 Hexadecimal = 19

560 Views
To convert octal to decimal number, use the Integer.parseInt() method with radix 8. Here, the radix is for Octal i.e. 8.Let’s say we have the following octal string.String myOctal = "25";To convert it to decimal, use the Integer.parseInt() method.int val = Integer.parseInt(myOctal, 8);The following is the complete example.Example Live Demopublic class Demo { public static void main(String []args) { String myOctal = "25"; System.out.println("Octal = "+myOctal); int val = Integer.parseInt(myOctal, 8); System.out.println("Decimal = "+val); } }OutputOctal = 25 Decimal = 21

397 Views
The method Integer.decode() decodes a string to an integer. It returns an Integer object holding the int value represented by the passed string value.Note − It accepts decimal, hexadecimal, and octal number.Let’s say the following is our string.String val = "2";The following is how you can decode the string to integer.Integer myInt = Integer.decode(val);Example Live Demopublic class Demo { public static void main(String []args) { String val = "2"; Integer myInt = Integer.decode(val); System.out.println("Integer = " + myInt); } }OutputInteger = 2

200 Views
To convert String to long, the following are the two methods.Method 1The following is an example wherein we use parseLong() method.Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = "5"; Long myLong = Long.parseLong(myStr); System.out.println("Long: "+myLong); } }OutputLong: 5Method 2The following is an example wherein we have used valueOf() and longValue() method to convert String to long.Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = "20"; long res = Long.valueOf(myStr).longValue(); System.out.println("Long: "+res); } }OutputLong: 20

1K+ Views
To check for Long overflow, we need to check the Long.MAX_VALUE with the multiplied long result, Here, Long.MAX_VALUE is the maximum value of Long type in Java.Let us see an example wherein long values are multiplied and if the result is more than the Long.MAX_VALUE, then an exception is thrown.The following is an example showing how to check for Long overflow.Example Live Demopublic class Demo { public static void main(String[] args) { long val1 = 6999; long val2 = 67849; System.out.println("Value1: "+val1); System.out.println("Value2: "+val2); long ... Read More