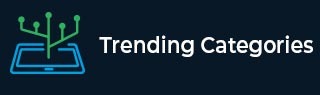
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

222 Views
To work with the GregorianCalendar class, import the following package −import java.util.GregorianCalendar;To get the day of week in month, use the following field −cal.get(Calendar.DAY_OF_WEEK_IN_MONTH)Above, cal is the GregorianCalendar object we created before −GregorianCalendar cal = new GregorianCalendar();The following is an example −Example Live Demoimport java.util.GregorianCalendar; import java.util.Calendar; import java.util.Date; public class Demo { public static void main(String[] args) { GregorianCalendar cal = new GregorianCalendar(); // date information System.out.println("Date Information.........."); System.out.println("Year = " + cal.get(Calendar.YEAR)); System.out.println("Month = " + (cal.get(Calendar.MONTH) + 1)); System.out.println("Date ... Read More

300 Views
For GregorianCalendar class, import the following package.import java.util.GregorianCalendar;Create an object.GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance();Now, use the following field and add() method with a negative one (-1) to display the previous day.cal.add((GregorianCalendar.DATE), -1)Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] a) { GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); System.out.println("Current date: " + cal.getTime()); // past date cal.add((GregorianCalendar.DATE), -1); System.out.println("Modified date (Previous Day): " + cal.getTime()); } }OutputCurrent date: Mon Nov 19 18:12:53 UTC 2018 Modified date (Previous Day): Sun Nov ... Read More

2K+ Views
For GregorianCalendar class, import the following package.import java.util.GregorianCalendar;Create an object.GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance();Now, use the following field and add() method with a negative one (-1) to display the previous month.cal.add((GregorianCalendar.MONTH), -1)Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] a) { GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); System.out.println("Current date: " + cal.getTime()); // past month cal.add((GregorianCalendar.MONTH), -1); System.out.println("Modified date (Previous Month): " + cal.getTime()); } }OutputCurrent date: Mon Nov 19 18:07:03 UTC 2018 Modified date (Previous Month): Fri Oct ... Read More

215 Views
Use the getMinimum() method in Java to returns the minimum value for the given calendar field. We will use it to set the minute, second and millisecond.Let us first declare a calendar object.Calendar dateNoon = Calendar.getInstance();Now, we will set the hour, minute, second and millisecond to get the closest possible millisecond of the day.dateNoon.set(Calendar.HOUR_OF_DAY, 12); dateNoon.set(Calendar.MINUTE, dateNoon.getMinimum(Calendar.MINUTE)); dateNoon.set(Calendar.SECOND, dateNoon.getMinimum(Calendar.SECOND)); // millisecond dateNoon.set(Calendar.MILLISECOND, dateNoon.getMinimum(Calendar.MILLISECOND));Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] argv) throws Exception { Calendar dateNoon = Calendar.getInstance(); // hour, minute and second dateNoon.set(Calendar.HOUR_OF_DAY, 12); ... Read More

171 Views
To convert String to Float Object, you can use a method Float.valueOf() method or you can even achieve this without using the in-built method.Let us see both the examples.The following is an example that converts String to Float Object using Float.valueOf() method.Example Live Demopublic class Demo { public static void main(String args[]) { Float f = Float.valueOf("30.67"); System.out.println(f); } }Output30.67Let us see another example.Example Live Demopublic class Demo { public static void main(String args[]) { Float f = new Float("45.88"); System.out.println(f); } }Output45.88

339 Views
Let us first declare a Float object.Float ob = new Float("29.35");Now, let us convert it to numeric primitive data type short using shortValue()short val1 = ob.shortValue();In the same way, we can convert it to int using intValue() method.short val1 = ob.intValue();The following is an example that converts Float to other numeric primitive data types as well.Example Live Demopublic class Demo { public static void main(String args[]) { Float ob = new Float("29.35"); // numeric primitive data types short val1 = ob.shortValue(); System.out.println(val1); int val2 = ... Read More

91 Views
The Float.valueOf() method is used in Java to convert string to float.The following are our string values.String str1 = "100.5"; String str2 = "200.5";To convert the string value to float, try the method valueOf()float val1 = (Float.valueOf(str1)).floatValue(); float val2 = (Float.valueOf(str2)).floatValue();The following is the complete example with output.Example Live Demopublic class Demo { public static void main(String args[]) throws Exception { String str1 = "100.5"; String str2 = "200.5"; float val1 = (Float.valueOf(str1)).floatValue(); float val2 = (Float.valueOf(str2)).floatValue(); System.out.println("Multiplication = " + (val1 * val2)); } }OutputMultiplication = 20150.25

3K+ Views
To display the month number, use the SimpleDateFormat(“M”)// displaying month number Format f = new SimpleDateFormat("M"); String strMonth = f.format(new Date()); System.out.println("Month Number = "+strMonth);Since, we have used the Format and SimpleDateFormat class above, therefore import the following packages. With that, we have also used the Date.import java.text.Format; import java.text.SimpleDateFormat; import java.util.Date;Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); SimpleDateFormat simpleformat = new SimpleDateFormat("dd/MMMM/yyyy hh:mm:s"); ... Read More

15K+ Views
Let us see how we can format date with SimpleDateFormat('MM/dd/yy')// displaying date Format f = new SimpleDateFormat("MM/dd/yy"); String strDate = f.format(new Date()); System.out.println("Current Date = "+strDate);Since, we have used the Format and SimpleDateFormat class above, therefore import the following packages. With that, we have also used the Date.import java.text.Format; import java.text.SimpleDateFormat; import java.util.Date;Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); SimpleDateFormat simpleformat = new SimpleDateFormat("dd/MMMM/yyyy hh:mm:s"); ... Read More

4K+ Views
To display the full day name, use the SimpleDateFormat(“EEEE”) as shown below −// displaying full-day name f = new SimpleDateFormat("EEEE"); String str = f.format(new Date()); System.out.println("Full Day Name = "+str);Since, we have used the Format and SimpleDateFormat class above, therefore import the following packages. With that, we have also used the Date.import java.text.Format; import java.text.SimpleDateFormat; import java.util.Date;Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); SimpleDateFormat simpleformat = ... Read More