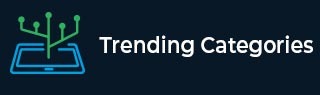
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

421 Views
To concatenate integers with a string value, you need to use the + operator.Let’s say the following is the string.String str = "Demo Text";Now, we will concatenate integer values with the above string.String res = str + 1 + 2 + 3 + 4 + 5;Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Demo Text"; System.out.println("String = "+str); String res = 99 + 199 + 299 + 399 + str; System.out.println(res); } }OutputString = Demo Text 996Demo Text

3K+ Views
To concatenate null to a string, use the + operator.Let’s say the following is our string.String str = "Demo Text";We will now see how to effortlessly concatenate null to string.String strNULL = str + "null";The following is the final example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Demo Text"; System.out.println("String = "+str); String strNULL = str + "null"; System.out.println("String concatenated with NULL: "+strNULL); } }OutputString = Demo Text String concatenated with NULL: Demo Textnull

613 Views
Let’s say the following is our string.String str = "DEMO98 TE4567XT";To display only the digits from the above string, we have used the replaceAll() method and replace all the characters with empty.str.replaceAll("\D", ""))The following is the final example that displays only digits from the string.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "DEMO98 TE4567XT"; System.out.println("String = "+str); System.out.println("Displaying digits: "+str.replaceAll("\D", "")); } }OutputString = DEMO98 TE4567XT Displaying digits: 984567

8K+ Views
To replace the first occurrence of a character in Java, use the replaceFirst() method.Here is our string.String str = "The Haunting of Hill House!";Let us replace the first occurrence of character “H”str.replaceFirst("(?:H)+", "B");The following is the complete example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "The Haunting of Hill House!"; System.out.println("String: "+str); String res = str.replaceFirst("(?:H)+", "B"); System.out.println("String after replacing a character's first occurrence: "+res); } }OutputString: The Haunting of Hill House! String after replacing a character's first occurance: The Baunting of Hill House!Read More

7K+ Views
To replace a character in a String, without using the replace() method, try the below logic.Let’s say the following is our string.String str = "The Haunting of Hill House!";To replace character at a position with another character, use the substring() method login. Here, we are replacing 7th position with character ‘p’int pos = 7; char rep = 'p'; String res = str.substring(0, pos) + rep + str.substring(pos + 1);The following is the complete example wherein a character at position 7 is replaced.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "The Haunting of Hill House!"; System.out.println("String: "+str); ... Read More

2K+ Views
Use replace() method to replace all occurrences of a given character in a string.Here is our string.String str = "THIS IS DEMO LINE $$$ NEW LINE";Let us now replace all occurrences of character $ with *str.replace("$", "*")The following is the complete example that replace all occurrences of $ with *Example Live Demopublic class Demo { public static void main(String[] args) { String str = "THIS IS DEMO LINE $$$ NEW LINE"; System.out.println("String = "+str); System.out.println("Replacing all occurrence of given character..."); System.out.println("Updated string = "+str.replace("$", "*")); } }OutputString = THIS IS DEMO LINE $$$ NEW LINE Replacing ... Read More

202 Views
User replaceAll() method to replace all occurrences of a given string. Let’s say the following is our string.String str = "THIS IS DEMO LINE AND NEW LINE!";Here, we are replacing all occurrences of string “LINE” to “TEXT”str.replaceAll("LINE", "TEXT");The following is the final example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "THIS IS DEMO LINE AND NEW LINE!"; Sytem.out.println("String = "+str); System.out.println("Replacing all occurrence of string LINE..."); System.out.println("Updated string = "+str.replaceAll("LINE", "TEXT")); } }OutputString = THIS IS DEMO LINE AND NEW LINE! Replacing all occurrence of string LINE... Updated string = ... Read More

2K+ Views
To check the end of a string, use the endsWith() method.Let’s say the following is our string.String str = "demo";Now, check for the substring “mo” using the endsWith() method in an if-else condition.if(str.endsWith("mo")) { System.out.println("The string ends with the word mo"); } else { System.out.println("The string does not end with the word mo"); }The following is the complete example with output.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "demo"; if(str.endsWith("mo")) { System.out.println("The string ends with the word mo"); } else { System.out.println("The string does not ... Read More

155 Views
A string is a class in Java that stores a series of characters enclosed within double quotes. Those characters are actually String-type objects. The string class is available in the 'java.lang' package. Suppose we have given a string and our task is to find the beginning of that string. Let's assume the beginning as the first two or three characters of that string. To check the beginning of a string, we can use several built-in methods available in Java including substring() and charAt(). Java Program to Check the Beginning of a String To check the beginning of a string we ... Read More

657 Views
To remove a character at a specified position, use the following logic −Let’s say the following is our string.String str = "The Haunting of Hill House!";We want to remove a character at position 7. For that, use the below logic.// removing character at position 7 int pos = 7; String res = str.substring(0, pos) + str.substring(pos + 1);The following is the complete example with output.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "The Haunting of Hill House!"; System.out.println("String: "+str); // removing character at position 7 int pos = 7; String res = str.substring(0, pos) + str.substring(pos + 1); ... Read More