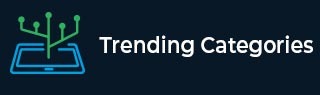
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
Narrowing conversion is needed when you convert from a larger size type to a smaller size. This is for incompatible data types, wherein automatic conversions cannot be done.Let us see an example wherein we are converting long to integer using Narrowing Conversion.Example Live Demopublic class Demo { public static void main(String[] args) { long longVal = 878; int intVal = (int) longVal; System.out.println("Long: "+longVal); System.out.println("Integer: "+intVal); } }OutputLong: 878 Integer: 878Let us see another example, wherein we are converting double to long using Narrowing Conversion.Example Live Demopublic class Demo { public static void main(String[] args) { double doubleVal = 299.89; long longVal = (long)doubleVal; ... Read More

1K+ Views
Use the substring() method to set a range of substring from a string. Let’s say the following is our string.String str = "pqrstuvw";Getting the range of string from 3 to 6String strRange = str.substring(3, 6);The following is the complete example wherein we have set a range for displaying substring from a string.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "pqrstuvw"; System.out.println("String: "+str); // range from 3 to 6 String strRange = str.substring(3, 6); System.out.println("Substring: "+strRange); } }OutputString: pqrstuvw Substring: stu

131 Views
Use the lastIndexOf() method to search for last index of a group of characters. Let’s say the following is our string.String myStr = "pqrstuvwxyzpqrst";Searching for the substring “pqrs” in the string to get the last index of its occurring in the string. We begin the search from index 3.int begnIndex = 3; strLastIndex = myStr.indexOf("pqrs", begnIndex);The following is an example, wherein we are searching for the last index of the substring “pqrs”Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = "pqrstuvwxyzpqrst"; int strLastIndex = 0; System.out.println("String: "+myStr); strLastIndex = myStr.lastIndexOf("pqrs"); System.out.println("The last index of ... Read More

270 Views
Use the indexOf() method to search for a substring from a given position.Let’s say the following is our string.String myStr = " pqrstuvwxyzpqrst";Searching for the substring “pqrs” in the string. We are beginning the index from 3 for our search.int begnIndex = 3; strLastIndex = myStr.indexOf("pqrs", begnIndex);Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = "pqrstuvwxyzpqrst"; int strLastIndex = 0; int begnIndex = 3; System.out.println("String: "+myStr); strLastIndex = myStr.indexOf("pqrs", begnIndex); System.out.println("The index of substring pqrs in the string beginning from index "+begnIndex+" = "+strLastIndex); } }OutputString: pqrstuvwxyzpqrst The index of substring pqrs ... Read More

101 Views
Use the indexOf() method to search for a character from a given position.Let’s say the following is our string.String myStr = "Amit Diwan";Here, we are searching for the character “i” in the string. We are beginning the index from 4 for our search.int begnIndex = 4; System.out.println("String: "+myStr); strLastIndex = myStr.indexOf('i', begnIndex);The following is the complete example.Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = "Amit Diwan"; int strLastIndex = 0; int begnIndex = 4; System.out.println("String: "+myStr); strLastIndex = myStr.indexOf('i', begnIndex); System.out.println("The index of character a in the string beginning from index "+begnIndex+" ="+strLastIndex); ... Read More

2K+ Views
Use the lastIndexOf() method to find the last occurrence of a character in a string in Java.Let’s say the following is our string.String myStr = "Amit Diwan";In the above string, we will find the last occurrence of character ‘i’myStr.lastIndexOf('i');The following is the complete example.Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = "Amit Diwan"; int strLastIndex = 0; System.out.println("String: "+myStr); strLastIndex = myStr.lastIndexOf('i'); System.out.println("The last index of character a in the string: "+strLastIndex); } }OutputString: Amit Diwan The last index of character a in the string: 6Read More

933 Views
Use the indexOf() method to search the index of a character in a string.Let’s say the following is our string.String myStr = "amit";Now, let us find the index of character ‘t’ in the above string.strIndex = myStr.indexOf('t');The following is the final example.Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = "amit"; int strIndex = 0; System.out.println("String: "+myStr); // finding index of character t strIndex = myStr.indexOf('t'); System.out.println("Character m found at index: "+strIndex); } }OutputString: amit Character m found at index: 3

29K+ Views
Let’s say the following is our string.String str = " This is demo text, and demo line!";To split a string with comma, use the split() method in Java.str.split("[,]", 0);The following is the complete example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "This is demo text, and demo line!"; String[] res = str.split("[,]", 0); for(String myStr: res) { System.out.println(myStr); } } }OutputThis is demo text and demo line!

11K+ Views
Let’s say the following is our string.String str = "This is demo text.This is sample text!";To split a string with dot, use the split() method in Java.str.split("[.]", 0);The following is the complete example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "This is demo text.This is sample text!"; String[] res = str.split("[.]", 0); for(String myStr: res) { System.out.println(myStr); } } }OutputThis is demo text This is sample text!

269 Views
We have the following string −String str = "This is demo text, and demo line!";To tokenize the string, let us split them after every period (.) and comma (,)String str = "This is demo text, and demo line!";The following is the complete example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "This is demo text, and demo line!"; String[] res = str.split("[, .]", 0); for(String myStr: res) { System.out.println(myStr); } } }OutputThis is demo text and demo line!