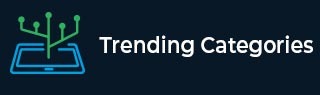
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

117 Views
To display milliseconds since the epoch, use the ‘Q’ Date and Time conversion specifier.System.out.printf("Milliseconds since epoch = %TQ", d);The above would display milliseconds since.1970-01-01 00:00:00 GMTExample Live Demoimport java.util.Date; public class Demo { public static void main(String[] args) { Date d = new Date(); System.out.printf("Nanoseconds = %tN", d); System.out.printf("Seconds since epoch = %ts", d); System.out.printf("Milliseconds since epoch = %TQ", d); } }OutputNanoseconds = 050000000 Seconds since epoch = 1543241478 Milliseconds since epoch = 1543241478050

345 Views
To display seconds since the epoch, use the ‘s’ Date and Time conversion specifier.System.out.printf("Seconds since epoch = %ts", d);The above would display seconds since.1970-01-01 00:00:00 GMTExample Live Demoimport java.util.Date; public class Demo { public static void main(String[] args) { Date d = new Date(); System.out.printf("Nanoseconds = %tN", d); System.out.printf("Seconds since epoch = %ts", d); } }OutputNanoseconds = 364000000 Seconds since epoch = 1543241402

750 Views
To display nanoseconds, use the ‘N’ Date and Time conversion specifier.System.out.printf("Nanoseconds = %tN", d);Example Live Demoimport java.util.Date; public class Demo { public static void main(String[] args) { Date d = new Date(); System.out.printf("Nanoseconds = %tN", d); } }OutputNanoseconds = 092000000

4K+ Views
To display number with thousands separator, set a comma flag.System.out.printf( "%,d",78567);The above would result.78, 567Let’s check for bigger numbers.System.out.printf( "%,d", 463758);The above would result.463,758Example Live Demopublic class Demo { public static void main( String args[] ) { System.out.printf( "%,d", 95647 ); System.out.printf( "%,d", 687467 ); System.out.printf( "%,.2f", 7546.21 ); System.out.printf( "%,.2f", 463758.787 ); System.out.printf( "%,.2f", 123456.5 ); } }Output95,647 687,467 7,546.21 463,758.79 123,456.50

122 Views
The following are the conversion characters for date-time −CharacterDescriptioncComplete date and timeFISO 8601 dateDU.S. formatted date (month/day/year)T24-hour timer12-hour timeR24-hour time, no secondsYFour-digit year (with leading zeroes)yLast two digits of the year (with leading zeroes)CFirst two digits of the year (with leading zeroes)BFull month namebAbbreviated month namemTwo-digit month (with leading zeroes)dTwo-digit day (with leading zeroes)eTwo-digit day (without leading zeroes)AFull weekday nameaAbbreviated weekday namejThree-digit day of year (with leading zeroes)HTwo-digit hour (with leading zeroes), between 00 and 23kTwo-digit hour (without leading zeroes), between 0 and 23ITwo-digit hour (with leading zeroes), between 01 and 12lTwo-digit hour (without leading zeroes), between 1 and 12MTwo-digit ... Read More

277 Views
Floating-point conversion characters include the following.CharacterDescription%edecimal number in computerized scientific notation%Edecimal number in computerized scientific notation%fdecimal number%gbased on computerized scientific notation or decimal format, %Gbased on computerized scientific notation or decimal format, Example Live Demopublic class Demo { public static void main(String[] args) throws Exception { System.out.printf("Integer conversions..."); System.out.printf( "Integer: %d", 889 ); System.out.printf( "Negative Integer: %d", -78 ); System.out.printf( "Octal: %o", 677 ); System.out.printf( "Hexadecimal: %x", 56 ); System.out.printf( "Hexadecimal: %X", 99 ); System.out.printf("Floating-point conversions..."); ... Read More

106 Views
Intergral conversion characters include the following.CharacterDescription%dInteger%oOctal%xHexadecimal%XHexadecimalExample Live Demopublic class Demo { public static void main(String[] args) throws Exception { System.out.printf( "Integer: %d", 889 ); System.out.printf( "Negative Integer: %d", -78 ); System.out.printf( "Octal: %o", 677 ); System.out.printf( "Hexadecimal: %x", 56 ); System.out.printf( "Hexadecimal: %X", 99 ); } }OutputInteger: 889 Negative Integer: -78 Octal: 1245 Hexadecimal: 38 Hexadecimal: 63

383 Views
Use the ‘c’ date conversion character to display UNIX date format in Java.System.out.printf("Unix date format: %tc",d);Above, d is a date object.Date d = new Date();Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); System.out.printf("Unix date format: %tc",d); System.out.printf("Unix date format: %Tc",d); } }OutputUnix date format: Mon Nov 26 12:24:10 UTC 2018 Unix date format: MON NOV 26 12:24:10 UTC 2018

742 Views
Use the ‘F’ date conversion character to display ISO 8601 standard date.System.out.printf("ISO 8601 standard date = %tF", d);Above, d is a date object.Date d = new Date();Example Live Demoimport java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] args) throws Exception { Date d = new Date(); System.out.printf("Four-digit Year = %TY",d); System.out.printf("Two-digit Year = %ty",d); System.out.printf("ISO 8601 standard date = %tF", d); } }OutputFour-digit Year = 2018 Two-digit Year = 18 ISO 8601 standard date = 2018-11-26

845 Views
Let’s say we have the following string.String str = "Demo Text!";To copy some part of the above string, use the getChars() method.// copy characters from string into chArray =str.getChars( 0, 5, chArray, 0 );The following is an example to copy characters from string into char Array in Java.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Demo Text!"; char chArray[] = new char[ 5 ]; System.out.println("String: "+str); // copy characters from string into chArray str.getChars( 0, 5, chArray, 0 ... Read More