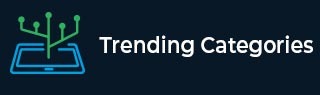
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

101 Views
To delete all whitespaces from a string, use the replaceAll() method and replace every whitespace with empty.Let’s say the following is our string.String str = "This is it!";Now, let us replace the whitespaces that will eventually delete them.String res = str.replaceAll("\s+","");Example Live Demopublic class Demo { public static void main(String []args) { String str = "This is it!"; System.out.println("String: "+str); String res = str.replaceAll("\s+",""); System.out.println("String after deleting whitespace: "+res); } }OutputString: This is it! String after deleting whitespace: Thisisit!

12K+ Views
To swap the case of a string, use.toLowerCase() for title case string. toLowerCase() for uppercase stringtoUpperCase() for lowercase stringLoop through what we discussed above for all the characters in the sting.for (int i = 0; i > len; i++) { c = str.charAt(i); // title case converted to lower case if (Character.isTitleCase(c)) { c = Character.toLowerCase(c); } // upper case converted to lower case if (Character.isUpperCase(c)) { c = Character.toLowerCase(c); } // lower case converted to upper case if (Character.isLowerCase(c)) { c ... Read More

410 Views
To construct on string from another, firstly take a charcter array for the first string.char ch[] = { 'A', 'M', 'I', 'T' }; String str1 = new String(ch);The above forms first string. Now, let us created another string from the first string.String str2 = new String(str1);In this way, we can easily construct one string from another.Example Live Demopublic class Demo { public static void main(String[] args) { char ch[] = { 'A', 'M', 'I', 'T' }; String str1 = new String(ch); String str2 = new String(str1); String str3 ... Read More

3K+ Views
To extract a substring as an array of characters in Java, use the getChars() method.Let’s say the following is our string and character array.String str = "World is not enough!"; char[] chArr = new char[10];Now, use the getChars() method to extract a substring.str.getChars(13, 19, chArr, 0);The above substring is an array of characters which can be displayed as shown in the complete example below −Example Live Demopublic class Demo { public static void main(String[] args) { String str = "World is not enough!"; char[] chArr = new char[10]; str.getChars(13, 19, chArr, ... Read More

344 Views
To get a string from a subset of the character array elements, use the copyValueOf() method. This method returns a String that represents the character sequence in the array specified.Here is our character array.char[] ch = { 'T', 'E', 'S', 'T', 'I', 'N', 'G'};Now, let us create a string from the subset of the above array elements.String str = String.copyValueOf(ch, 4, 2);Example Live Demopublic class Demo { public static void main(String[] args) { char[] ch = { 'T', 'E', 'S', 'T', 'I', 'N', 'G'}; String str = String.copyValueOf(ch, 4, 2); System.out.println(str); } }OutputIN

79 Views
Here is our character array.char[] ch = { 'T', 'E', 'S', 'T', 'I', 'N', 'G'};Create string object from some part of a string using the following String constructor. Through this we are fetching substring “IN” from the character array.String str = new String(ch, 4, 2);Example Live Demopublic class Demo { public static void main(String[] args) { char[] ch = { 'T', 'E', 'S', 'T', 'I', 'N', 'G'}; String str = new String(ch, 4, 2); System.out.println(str); } }OutputIN

466 Views
Here is our character array.char[] ch = { 'T', 'E', 'S', 'T'};To create string object from the above character array is quite easy. Add the array to the string parameter as shown below −String str = new String(ch);Example Live Demopublic class Demo { public static void main(String[] args) { char[] ch = { 'T', 'E', 'S', 'T'}; String str = new String(ch); System.out.println(str); } }OutputTEST

437 Views
Argument indices allow programmers to reorder the output. Let us see an example.Example Live Demopublic class Demo { public static void main(String[] args) { System.out.printf("Before reordering = %s %s %s %s %s %s", "one", "two", "three", "four", "five", "six" ); System.out.printf("After reordering = %6$s %5$s %4$s %3$s %2$s %1$s", "one", "two", "three", "four", "five", "six" ); System.out.printf("Before reordering = %d %d %d", 100, 200, 300); System.out.printf("After reordering = %2$d %3$d %1$d", 100, 200, 300); } }OutputBefore reordering = one two three four five six After reordering = ... Read More

83 Views
To display localized method name in Java, use the ‘B’ conversion character.System.out.printf("Localized month : %TB", d);To display method name in lowercase, use the “%tb”System.out.printf("Localized month : %tB", d);Example Live Demoimport java.util.Date; public class Demo { public static void main(String[] args) { Date d = new Date(); System.out.printf("Morning/afternoon indicator: %tp", d); System.out.printf("Morning/afternoon indicator: %Tp", d); System.out.printf("Localized month : %tB", d); System.out.printf("Localized month : %TB", d); } }OutputMorning/afternoon indicator: pm Morning/afternoon indicator: PM Localized month : November Localized month : NOVEMBERRight justify and left justify values ... Read More

67 Views
Locale-specific morning/afternoon indicator is the AM/PM marker indicator.Use the ‘p’ conversion character to display AM/PM.System.out.printf("Morning/afternoon indicator: %tp",d);Example Live Demoimport java.util.Date; public class Demo { public static void main(String[] args) { Date d = new Date(); System.out.printf("Morning/afternoon indicator: %tp",d); System.out.printf("Morning/afternoon indicator: %Tp",d); } }OutputMorning/afternoon indicator: pm Morning/afternoon indicator: PM