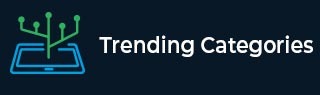
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

1K+ Views
To join strings in Java, use the String.join() method. The delimiter set as the first parameter in the method is copied for each element.Let’s say we want to join the strings “Demo” and “Text”. With that, we want to set a delimeter $. For that, use the join() method as shown below −String.join("$","Demo","Text");The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = String.join("$","Demo","Text"); System.out.println("Joined strings: "+str); } }OutputJoined strings: Demo$Text

974 Views
The following is our string.String str = "pqrst";In the above string, we want to search for the following set of characters.// set of characters to be searched char[] chSearch = {'p', 'q', r'};For this, loop through the length of the string and check every character in the string “str”. If the matching character from “chSearch” is found in the string, then it would be a success.The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "pqrst"; // characters to be searched char[] ... Read More

572 Views
Here, we have a string.String str = "abcde";In the above string, we want to search for the following set of characters.// set of characters to be searched char[] chSearch = {'b', 'c'};For this, loop through the length of the string and check every character in the string str. If the matching character from “chSearch” is found in the string, then it would be success.The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "abcde"; // set of characters to be searched char[] ... Read More

1K+ Views
Let’s say the following is our string.String myStr = "";Now, we will check whether the above string is whitespace, empty ("") or null.if(myStr != null && !myStr.isEmpty() && !myStr.trim().isEmpty()) { System.out.println("String is not null or not empty or not whitespace"); } else { System.out.println("String is null or empty or whitespace"); }The following is an example that checks for an empty string.Example Live Demopublic class Demo { public static void main(String[] args) { String myStr = ""; if(myStr != null && !myStr.isEmpty() && !myStr.trim().isEmpty()) { System.out.println("String is not null ... Read More

16K+ Views
To remove newline, space and tab characters from a string, replace them with empty as shown below.replaceAll("[\t ]", "");Above, the new line, tab, and space will get replaced with empty, since we have used replaceAll()The following is the complete example.Example Live Demopublic class Demo { public static void main(String[] args) { String originalStr = "Demo\tText"; System.out.println("Original String with tabs, spaces and newline: "+originalStr); originalStr = originalStr.replaceAll("[\t ]", ""); System.out.println("String after removing tabs, spaces and new line: "+originalStr); } }OutputOriginal String with tabs, spaces and newline: Demo Text ... Read More

9K+ Views
The MMMM format for months is like entire month name: January, February, March, etc. We will use it like this.SimpleDateFormat("MMM");Let us see an example.// displaying month in MMMM format SimpleDateFormat simpleformat = new SimpleDateFormat("MMMM"); String strMonth= simpleformat.format(new Date()); System.out.println("Month in MMMM format = "+strMonth);Above, we have used the SimpleDateFormat class, therefore the following package is imported.import java.text.SimpleDateFormat;The following is an example.Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); ... Read More

4K+ Views
The MMM format for months is the short name i.e. Jan, Feb, Mar, Apr, etc. Here, we will use the following.SimpleDateFormat("MMM");Let us see an example.// displaying month in MMM format SimpleDateFormat simpleformat = new SimpleDateFormat("MMM"); String strMonth= simpleformat.format(new Date()); System.out.println("Month in MMM format = "+strMonth);Above, we have used the SimpleDateFormat class, therefore the following package is imported.import java.text.SimpleDateFormat;The following is an example.Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Calendar; public class Demo { public static void main(String[] args) throws Exception { // displaying current date and time Calendar cal = Calendar.getInstance(); ... Read More

116 Views
The following is our string.String str = "Jack";Now take a StringBuilder object.StringBuilder strBuilder = new StringBuilder();Perform left padding and extend the string length to 20. The string that will be padded comes on the left.while (strBuilder.length() + str.length() < 20) { strBuilder.append("demo"); }The following is an example wherein we have left padded a string with another string “demo”Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Jack"; StringBuilder strBuilder = new StringBuilder(); // left padding with a string while (strBuilder.length() + str.length() ... Read More

3K+ Views
Strings have no newlines. We can form them into two lines by concatenating a newline string. Use System lineSeparator to get a platform-dependent newline string.The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "one" + System.lineSeparator() + "two"; System.out.println(str); } }Outputone twoLet us see another example. On Linux based system, the program will work correctly.Example Live Demopublic class Demo { public static void main(String[] args) { String str = System.lineSeparator(); System.out.println((int) str.charAt(0)); } }Output10

133 Views
To format a string, use the String.format() method in Java. The following is an example that formats a string %s.Example Live Demopublic class Demo { public static void main(String []args) { String str = String.format("%s %s", "demo", "text"); System.out.print("String: "+str); } }OutputString: demo textLeft pad a stringTo left pad a string, use the String.format and set the spaces.String.format("|%20s|", "demotext")If you add 30 above, it will display the first string after 30 spaces from the beginning.String.format("|%30s|", "demotext")The following is an example.Example Live Demopublic class Demo { public static void main(String []args) { ... Read More