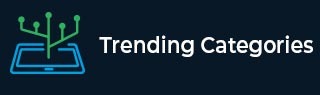
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
A string is a class in Java that stores a series of characters enclosed within double quotes and a continuous sequence of characters within that string is termed as substring. Those characters are actually String-type objects. This article aims to write Java programs to check if a string contains a substring or not. To check whether the given string contains a substring or not, we can use indexOf(), contains() and substring() methods along with conditional blocks. Java Program to Check if a String Contains a Substring We are going to use the following built-in methods in our Java programs to ... Read More

964 Views
While displaying a long input string, we are sometimes required to process them in smaller units. At that time, converting that string into the InputStream will be helpful. To convert a given string into the InputStream, Java provides ByteArrayInputStream class that is used along with a built method named getBytes(). In this article, we will learn how we can use the ByteArrayInputStream class to convert a string into an InputStream with the help of example programs. Java Program to Convert a String into the InputStream This section will introduce a few concepts that will help us to understand the Java ... Read More

249 Views
LinkedList is a generic class of Java Collection Framework that implements three interfaces namely List, Deque, and Queue. It provides the features of a LinkedList data structure which is a linear data structure where each element are linked with each other. There are several operations we can perform on LinkedList including appending, removing and traversing of elements. To add elements to a LinkedList Collection we can use various built-in methods such as add(), addFirst() and addLast(). We are going to explore how we can use these methods to add elements to a LinkedList. Adding elements to a LinkedList in Java ... Read More

6K+ Views
A string is a class of 'java.lang' package that stores a series of characters. Those characters are actually String-type objects. We must enclose the value of string within double quotes. Generally, we can represent characters in lowercase and uppercase in Java. And, it is also possible to convert lowercase characters into uppercase. This article aims to discuss a Java program to convert the first character of each word into uppercase in a string. Java program to Capitalize the first character of each word in a String Before making a Java program to convert the first lowercase character of a ... Read More

8K+ Views
In this article, we will understand how to print X star pattern. The pattern is formed by using multiple for-loops and print statements. Below is a demonstration of the same: − Input Suppose our input is − Enter the number : 8 Output The desired output would be − The X star pattern : X X X X X X X X X X ... Read More

879 Views
In this article, we will understand how to print half diamond star pattern. The pattern is formed by using multiple for-loops and print statements.Below is a demonstration of the same −InputSuppose our input is −Enter the number of rows : 8OutputThe desired output would be −The half diamond pattern : * ** *** **** ***** ****** ******* ******** ******* ****** ***** **** *** ** *AlgorithmStep 1 - START Step 2 - Declare three integer values namely i, j and my_input Step 3 - Read the required values from the user/ define the values Step 4 - We iterate through two ... Read More

4K+ Views
In this article, we will understand how to print hollow right triangle star pattern. The pattern is formed by using multiple for-loops and print statements. For the pyramid at the first line it will print one star, and at the last line it will print n number of stars. For other lines it will print exactly two stars at the start and end of the line, and there will be some blank spaces between these two starts.Below is a demonstration of the same −InputSuppose our input is −Enter the size : 8OutputThe desired output would be −The hollow pyramid triangle ... Read More

1K+ Views
In this article, we will understand how to print inverted star pattern. The pattern is formed by using multiple for-loops and print statements.Below is a demonstration of the same −InputSuppose our input is −Enter the size : 8OutputThe desired output would be −The inverted star pattern *************** ************* *********** ********* ******* ***** *** *AlgorithmStep 1 - START Step 2 - Declare four integer values namely i, j, k and my_input. Step 3 ... Read More

611 Views
In this article, we will understand how to print 8-star pattern. The pattern is formed by using multiple for-loops and print statements.Below is a demonstration of the same −InputSuppose our input is −Enter the number : 8OutputThe desired output would be −The 8 pattern : ****** * * * * * * * * * * * * ****** * * * * * * * * * * * ... Read More

454 Views
If someone wants to build a strong foundation in Java programming language. Then, it is necessary to understand the working of loops. Also, solving the pyramid pattern problems is the best way to enhance the fundamentals of Java as it includes extensive use of the for and while loops. This article aims to provide a few Java programs to print pyramid patterns with the help of different types of loops available in Java. Java Program to Create Pyramid Patterns We are going to print the following Pyramid Patterns through Java programs − Inverted Star Pyramid Star Pyramid Numeric Pyramid ... Read More