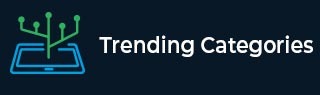
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

4K+ Views
Escape sequences are a unique kind of character that are used to indicate a different way of interpreting a group of characters. An escape sequence in Java is a character that is preceded by a backslash (). An escape sequence is treated by the Java compiler as a single character with unique meaning. Java frequently employs the following escape sequences: \t: Adds a new tab : Adds a new line \r: Adds a carriage return ': Adds a single quote ": Adds a double quote \: Adds a backslash These escape sequences can be used to control the ... Read More

811 Views
Let’s say we have the following string.String str = "{Java is a programming language} {James Gosling developed it.}";Above, the string is enclosed with parentheses. We can split a string with these parentheses using the split() method.String[] strSplit = str.split("[{}]");The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) throws Exception { String str = "{Java is a programming language} {James Gosling developed it.}"; String[] strSplit = str.split("[{}]"); System.out.println("Splitting String..."); for (int i = 0; i < strSplit.length; i++) System.out.println(strSplit[i]); ... Read More

411 Views
Let’s say the following is our string.String str = "Java is a programming language. James Gosling developed it.";We will now see how to split a string using the split() method. Include the delimiter as the parameter.String[] strSplit = str.split("\.");Above, we have split the string with dot as you can see under the split methods parameter.The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Java is a programming language. James Gosling developed it."; System.out.println("String: "+str); String[] strSplit = str.split("\."); ... Read More

5K+ Views
The following are our strings with single and double quote.String str1 = "This is Jack's mobile"; String str2 = "\"This is it\"!";Above, for single quote, we have to mention it normally like.This is Jack's mobileHowever, for double quotes, use the following and add a slash in the beginning as well as at the end.String str2 = "\"This is it\"!";The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str1 = "This is Jack's mobile"; String str2 = "\"This is it\"!"; System.out.println("Displaying Single Quote: "+str1); ... Read More

262 Views
The following is our string −String str = "";We will check whether it is empty or not using the isEmpty() method. The result will be a boolean −str.isEmpty()The following is an example −Example Live Demopublic class Demo { public static void main(String[] args) { String str = ""; System.out.println("String is empty: "+str.isEmpty()); } }OutputString is empty: true

271 Views
Let’s say we have the following string.String str = "Learning never ends! Learning never stops!";In the above string, we need to find out how many times the substring “Learning” appears.For this, loop until the index is not equal to 1 and calculate.while ((index = str.indexOf(subString, index)) != -1) { subStrCount++; index = index + subString.length(); }The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Learning never ends! Learning never stops!"; System.out.println("String: "+str); int subStrCount = 0; String subString ... Read More

5K+ Views
We have the following string with a separator.String str = "David-Warner";We want the substring before the last occurrence of a separator. Use the lastIndexOf() method.For that, you need to get the index of the separator using indexOf()String separator ="-"; int sepPos = str.lastIndexOf(separator); System.out.println("Substring before last separator = "+str.substring(0, sepPos));The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "David-Warner"; String separator ="-"; int sepPos = str.lastIndexOf(separator); if (sepPos == -1) { System.out.println(""); ... Read More

774 Views
We have the following string with two separators.String str = "Tom-Hank-s";We want the index of the first occurrence of the separator.For that, you need to get the index of the separator using indexOf()String separator ="-"; int sepPos = str.indexOf(separator);The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Tom-Hank-s"; String separator ="-"; System.out.println("String: "+str); int sepPos = str.indexOf(separator); System.out.println("Separator's first occurrence: "+sepPos); } }OutputString: Tom-Hank-s Separator's first occurrence: 3

8K+ Views
We have the following string with a separator.String str = "Tom-Hanks";We want the substring after the first occurrence of the separator i.e.HanksFor that, first you need to get the index of the separator and then using the substring() method get, the substring after the separator.String separator ="-"; int sepPos = str.indexOf(separator); System.out.println("Substring after separator = "+str.substring(sepPos + separator.length()));The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String str = "Tom-Hanks"; String separator ="-"; int sepPos = str.indexOf(separator); if (sepPos == -1) ... Read More

252 Views
To format date time with Join, set the date as a string and do not forget to add the delimeter.For delimeter “/” in the dateString.join("/","11","11","2018");For delimeter “:” in the date.String.join(":", "10","20","20");The following is an example.Example Live Demopublic class Demo { public static void main(String[] args) { String d = String.join("/","11","11","2018"); System.out.print("Date: "+d); String t = String.join(":", "10","20","20"); System.out.println("Time: "+t); } }OutputDate: 11/11/2018 Time: 10:20:20