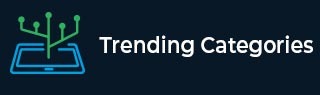
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

171 Views
Import the following package for to work with Calendar class in Java, import java.util.Calendar;Create a calendar class now.Calendar cal = Calendar.getInstance();To display entire time information, use the following fields.cal.get(Calendar.HOUR_OF_DAY) cal.get(Calendar.HOUR) cal.get(Calendar.MINUTE) cal.get(Calendar.SECOND) cal.get(Calendar.MILLISECOND)The following is the final example.Example Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar cal = Calendar.getInstance(); // current date and time System.out.println(cal.getTime().toString()); // time information System.out.println("Hour (24 hour format) : " + cal.get(Calendar.HOUR_OF_DAY)); System.out.println("Hour (12 hour format) : " + cal.get(Calendar.HOUR)); ... Read More

3K+ Views
For using Calendar class, import the following package.import java.util.Calendar;Using the Calendar class, create an object.Calendar calendar = Calendar.getInstance();Now, create a string array of the month names.String[] month = new String[] {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December" };Display the month name.month[calendar.get(Calendar.MONTH)]The following is an example.Example Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); String[] month = new String[] {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December" }; System.out.println("Current Month = " + month[calendar.get(Calendar.MONTH)]); ... Read More

3K+ Views
For using Calendar class, import the following package.import java.util.Calendar;Using the Calendar class, create an object.Calendar calendar = Calendar.getInstance();Now, create a string array of the day names.String[] days = new String[] { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" };Display the day name.days[calendar.get(Calendar.DAY_OF_WEEK) - 1]The following is the final example.Example Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); System.out.println("Day: " + (calendar.get(Calendar.DATE))); System.out.println("Month: " + (calendar.get(Calendar.MONTH) + 1)); System.out.println("Year: " + (calendar.get(Calendar.YEAR))); String[] days = new String[] ... Read More

10K+ Views
To get the day of the week, use Calendar.DAY_OF_WEEK.Firstly, declare a calendar object and get the current date and time.Calendar calendar = Calendar.getInstance(); System.out.println(calendar.getTime().toString());Now, fetch the day of the week in an integer variable.int day = calendar.get(Calendar.DAY_OF_WEEK);The following is the final example.Example Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); System.out.println(calendar.getTime().toString()); int day = calendar.get(Calendar.DAY_OF_WEEK); System.out.println("Day: " + day); int hour = calendar.get(Calendar.HOUR_OF_DAY); System.out.println("Hour: " + hour); int minute = ... Read More

776 Views
To display the current time in another time zone, use the TimeZone class. To use it, import the following package.import java.util.TimeZone;Firstly, set the TimeZone.cal.setTimeZone(TimeZone.getTimeZone("Europe/Sofia"));Now, display the date using the Calendar object.cal.get(Calendar.HOUR_OF_DAY) cal.get(Calendar.MINUTE) cal.get(Calendar.SECOND) cal.get(Calendar.MILLISECOND)The following is the final example.Example Live Demoimport java.util.Calendar; import java.util.TimeZone; public class Demo { public static void main(String[] args) { Calendar cal = Calendar.getInstance(); System.out.println("Europe/Sofia TimeZone..."); cal.setTimeZone(TimeZone.getTimeZone("Europe/Sofia")); System.out.println("Hour = " + cal.get(Calendar.HOUR_OF_DAY)); System.out.println("Minute = " + cal.get(Calendar.MINUTE)); System.out.println("Second = " + cal.get(Calendar.SECOND)); System.out.println("Millisecond = " ... Read More

1K+ Views
For using Calendar class, import the following package.import java.util.Calendar;Now, let us create an object of Calendar class.Calendar calendar = Calendar.getInstance();Set the date, month and year.calendar.set(Calendar.YEAR, 2018); calendar.set(Calendar.MONTH, 11); calendar.set(Calendar.DATE, 18);Create a Date object using Calendar class.java.util.Date dt = calendar.getTime();The following is an example.Example Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); // Set year, month and date calendar.set(Calendar.YEAR, 2018); calendar.set(Calendar.MONTH, 11); calendar.set(Calendar.DATE, 18); // util date object java.util.Date dt = ... Read More

380 Views
To get the day of the week, use the Calendar.DAY_OF_WEEK.Firstly, let us get the current date.java.util.Date utilDate = new java.util.Date(); java.sql.Date dt = new java.sql.Date(utilDate.getTime());Now, using GregorianCalendar, set the time.java.util.GregorianCalendar cal = new java.util.GregorianCalendar(); cal.setTime(dt);The last step would display the day of the week as shown in the following example.Example Live Demoimport java.text.ParseException; public class Demo { public static void main(String[] args) throws ParseException { java.util.Date utilDate = new java.util.Date(); java.sql.Date dt = new java.sql.Date(utilDate.getTime()); System.out.println("Today's date: "+dt); java.util.GregorianCalendar cal = new java.util.GregorianCalendar(); cal.setTime(dt); ... Read More

3K+ Views
Import the following package to work with Date class.import java.util.Date;No create a Date object.Date d = new Date();Let us convert the current date to milliseconds.d.getTime()The following is an example.Example Live Demoimport java.util.Date; public class Demo { public static void main(String[] args) { Date d = new Date(); System.out.println("Date = " + d); System.out.println("Milliseconds since January 1, 1970, 00:00:00 GMT = " + d.getTime()); } }OutputDate = Mon Nov 19 06:30:11 UTC 2018 Milliseconds since January 1, 1970, 00:00:00 GMT = 1542609011369

452 Views
Firstly, create a Calendar class object.Calendar calendar = Calendar.getInstance();Now, import the following package.import java.sql.Date;Using a Date class now and creating an object would belong to the above package. Convert the current time to the java.sql.Date Object.Date sqlDate = new Date((calendar.getTime()).getTime());The following is an example.Example Live Demoimport java.util.Calendar; import java.sql.Date; import java.text.ParseException; public class Demo { public static void main(String[] args) throws ParseException { Calendar calendar = Calendar.getInstance(); // object Date sqlDate = new Date((calendar.getTime()).getTime()); System.out.println(sqlDate); } }Output2018-11-19Read More

455 Views
First, let us create a java.util.Date object.// util object java.util.Date utilObj = new java.util.Date();Now, create a java.sql.Date object.java.sql.Date sqlObj = new java.sql.Date();Converting from a java.util.Date object to a java.sql.Date object.java.sql.Date sqlObj = new java.sql.Date(utilObj.getTime());The following is an example.Example Live Demoimport java.util.Date; public class Example { public static void main(String args[]) { // util object java.util.Date utilObj = new java.util.Date(); // sql object java.sql.Date sqlObj = new java.sql.Date(utilObj.getTime()); System.out.println("Util Date = " + utilObj); System.out.println("SQL Date = " + sqlObj); } }OutputUtil ... Read More