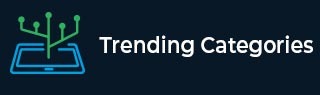
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
Firstly, import the following Java packages −import java.text.SimpleDateFormat; import java.util.Date;Now, create objects −Date dt = new Date(); SimpleDateFormat dateFormat;Displaying date in the format we wantdateFormat = new SimpleDateFormat("E MMM dd yyyy");The following is an example −Example Live Demoimport java.text.SimpleDateFormat; import java.util.Date; public class Demo { public static void main(String args[]) { Date dt = new Date(); SimpleDateFormat dateFormat; dateFormat = new SimpleDateFormat("E MMM dd yyyy"); System.out.println("Date: "+dateFormat.format(dt)); } }OutputDate: Thu Nov 22 2018

2K+ Views
Firstly, import the following Java packagesimport java.text.SimpleDateFormat; import java.util.Date;Now, create objectsDate dt = new Date(); SimpleDateFormat dateFormat;Displaying date in the format we want −dateFormat = new SimpleDateFormat("dd MMM yyyy hh:mm:ss zzz");The following is an example −Example Live Demoimport java.text.SimpleDateFormat; import java.util.Date; public class Demo { public static void main(String args[]) { Date dt = new Date(); SimpleDateFormat dateFormat; dateFormat = new SimpleDateFormat("dd MMM yyyy hh:mm:ss zzz"); System.out.println("Date: "+dateFormat.format(dt)); } }OutputDate: 22 Nov 2018 07:53:58 UTC

92 Views
For default format, use −DateFormat.getDateInstance(DateFormat.DEFAULT)To parse the string date value, use the parse() methodDate dt = DateFormat.getDateInstance(DateFormat.DEFAULT).parse("Nov 19, 2018");The following is an example −Example Live Demoimport java.text.DateFormat; import java.util.Date; public class Demo { public static void main(String[] argv) throws Exception { // parse date Date dt = DateFormat.getDateInstance(DateFormat.DEFAULT).parse("Nov 19, 2018"); System.out.println("Date: "+dt); } }OutputDate: Mon Nov 19 00:00:00 UTC 2018

315 Views
In Java, you can parse string date time value input usingSimpleDateFormat("E, dd MMM yyyy HH:mm:ss Z");We have used the above class since we imported the following package −import java.text.SimpleDateFormat;Now, we can display the date in the same format −Date dt = (Date) dateFormatter.parseObject("Tue, 20 Nov 2018 16:10:45 -0530");The following is an example −Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; public class Main { public static void main(String[] argv) throws Exception { Format dateFormatter = new SimpleDateFormat("E, dd MMM yyyy HH:mm:ss Z"); Date dt = (Date) dateFormatter.parseObject("Tue, 20 Nov 2018 16:10:45 -0530"); ... Read More

179 Views
Create a SimpleDateFormat object −SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy.MM.dd.HH.mm.ss");Do not forget to import the following package for SimpleDateFormat class −import java.text.SimpleDateFormat;Now, since we have set the format for date above, let us parse the date using parseObject() method −Date dt = (Date) dateFormat.parseObject("2018.11.22.11.50.15");The following is an example −Example Live Demoimport java.util.Date; import java.text.SimpleDateFormat; public class Demo { public static void main(String[] argv) throws Exception { SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy.MM.dd.HH.mm.ss"); // parse System.out.println("Parse Date and Time..."); Date dt = (Date) dateFormat.parseObject("2018.11.22.11.50.15"); System.out.println(dt); } }OutputParse ... Read More

145 Views
UseSimpleDateFormat('dd-MMM-yy') for string date.Format dateFormatter = new SimpleDateFormat("dd-MMM-yy");For above class, do not forget to import the following package, else an error would be visible.import java.text.SimpleDateFormat;Now, parse the date.Date dt = (Date) dateFormatter.parseObject("20-Nov-18");Example Live Demoimport java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; public class Main { public static void main(String[] argv) throws Exception { Format dateFormatter = new SimpleDateFormat("dd-MMM-yy"); // parse Date dt = (Date) dateFormatter.parseObject("20-Nov-18"); System.out.println("Date = "+dt); } }OutputDate = Tue Nov 20 00:00:00 UTC 2018

148 Views
To get the time format, use the DateFormat class and create a new object.DateFormat dateFormatter = new SimpleDateFormat("hh.mm.ss a");Now, parse the time.dateFormatter.parse("12.55.20 PM");Example Live Demoimport java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Date; public class Main { public static void main(String[] argv) throws Exception { DateFormat dateFormatter = new SimpleDateFormat("hh.mm.ss a"); System.out.println("Parse Time..."); Date dt = (Date) dateFormatter.parse("12.55.20 PM"); System.out.println(dt); } }OutputParse Time... Thu Jan 01 12:55:20 UTC 1970

251 Views
For GregorianCalendar class, import the following package.import java.util.GregorianCalendar;Here is the object.GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance();Now, let us get the past date, using the add() method with a negative value.// past date cal.add((GregorianCalendar.DATE), -1);Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] a) { GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); System.out.println("Current date: " + cal.getTime()); // past date cal.add((GregorianCalendar.DATE), -1); System.out.println("Modified date (Previous Date): " + cal.getTime()); } }OutputCurrent date: Mon Nov 19 18:01:37 UTC 2018 Modified date (Previous Month): Fri ... Read More

109 Views
For GregorianCalendar class, import the following package.import java.util.GregorianCalendar;Create an object.GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance();Now, use the following field and add() method with a negative one (-1) to display the previous year.cal.add((GregorianCalendar.YEAR), -1)Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] a) { GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); System.out.println("Current date: " + cal.getTime()); // previous year cal.add((GregorianCalendar.YEAR), -1); System.out.println("Modified date: " + cal.getTime()); } }OutputCurrent date: Mon Nov 19 18:05:49 UTC 2018 Modified date: Sun Nov 19 18:05:49 UTC 2017

685 Views
For GregorianCalendar class, import the following package.import java.util.GregorianCalendar;Firstly, let us display the current date and time.GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); System.out.println("Current date: " + cal.getTime());Now, modify the date. Here we are adding two days to the month using the add() method.cal.add((GregorianCalendar.MONTH), 2);Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] a) { GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); System.out.println("Current date: " + cal.getTime()); cal.add((GregorianCalendar.MONTH), 2); System.out.println("Modified date: " + cal.getTime()); } }OutputCurrent date: Mon Nov 19 17:52:55 UTC 2018 Modified date: Sat Jan ... Read More