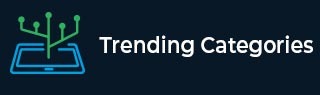
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

2K+ Views
To get the days remaining in the current year, find the difference between the total days in the current year with the total number of days passed.First, calculate the day of year.Calendar calOne = Calendar.getInstance(); int dayOfYear = calOne.get(Calendar.DAY_OF_YEAR);Now, calculate the total days in the current year 2018.int year = calOne.get(Calendar.YEAR); Calendar calTwo = new GregorianCalendar(year, 11, 31); int day = calTwo.get(Calendar.DAY_OF_YEAR); System.out.println("Days in current year: "+day);The following is the final example that finds the difference between the above two to get the days remaining in the current year.ExampleLive Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static ... Read More

429 Views
To get the days until the end of the year, find the difference between the total days in the current year with the total number of passed.Firstly, calculate the day of the year.Calendar calOne = Calendar.getInstance(); int dayOfYear = calOne.get(Calendar.DAY_OF_YEAR);Now, calculate the total days in the current year 2018.int year = calOne.get(Calendar.YEAR); Calendar calTwo = new GregorianCalendar(year, 11, 31); int day = calTwo.get(Calendar.DAY_OF_YEAR); System.out.println("Days in current year: "+day);Find the difference and you will get the days until the end of the year.The following is the complete example.Example Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String ... Read More

99 Views
Use the == operator to compare two calendar objects.Let us first create the first calendar object and set date −Calendar date1 = Calendar.getInstance(); date1.set(Calendar.YEAR, 2040); date1.set(Calendar.MONTH, 10); date1.set(Calendar.DATE, 25); date1.set(Calendar.HOUR_OF_DAY, 11); date1.set(Calendar.MINUTE, 30); date1.set(Calendar.SECOND, 10);Now, the following is the second calendar object −Calendar date2 = Calendar.getInstance(); date2.set(Calendar.YEAR, 2040); date2.set(Calendar.MONTH, 10); date2.set(Calendar.DATE, 25); date2.set(Calendar.HOUR_OF_DAY, 11); date2.set(Calendar.MINUTE, 30); date2.set(Calendar.SECOND, 10);Let is now compare them using == and && operators −if(date1.get(Calendar.SECOND) == date2.get(Calendar.SECOND) && date1.get(Calendar.MINUTE) == date2.get(Calendar.MINUTE) && date1.get(Calendar.HOUR) == date2.get(Calendar.HOUR) && date1.get(Calendar.DAY_OF_YEAR) == date2.get(Calendar.DAY_OF_YEAR) && date1.get(Calendar.YEAR) == date2.get(Calendar.YEAR) ) { System.out.println("The local time for the calendar objects is same..."); } ... Read More

3K+ Views
To get the day of week for a particular date in Java, use the Calendar.DAY_OF_WEEK constant.Let us set a date first.Calendar one = new GregorianCalendar(2010, Calendar.JULY, 10);Since, we have used the Calendar as well as GregorianCalendar classes, therefore at first, import the following packages.import java.util.Calendar; import java.util.GregorianCalendar;Now, we will find the day of week.int day = one.get(Calendar.DAY_OF_WEEK);The following is an exampleExample Live Demoimport java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] argv) throws Exception { Calendar one = new GregorianCalendar(2010, Calendar.JULY, 10); int day = one.get(Calendar.DAY_OF_WEEK); System.out.println(day); ... Read More

149 Views
To compare dates if a date is before another date, use the Calendar.before() method.The Calendar.before() method returns whether this Calendar's time is before the time represented by the specified Object. First, let us set a date which is before the current dateCalendar date1 = Calendar.getInstance(); date1.set(Calendar.YEAR, 2010); date1.set(Calendar.MONTH, 11); date1.set(Calendar.DATE, 20);Here is our current dateCalendar date = Calendar.getInstance();Now, use the after() method to compare both the dates as shown in the following exampleThe following is an exampleExample Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar date1 = Calendar.getInstance(); ... Read More

547 Views
In the world of Java programming, there are a few scenarios where we are required to deal with the date and time such as while developing a calendar application, attendance management system in Java and checking age of two persons. Also, the date is a way of keeping track of our time as it is an integral part of our daily lives. Therefore, Java provides classes like Date and LocalDate to work with date and time. And, to compare and check if a date is after another or not, it provides a few useful built-in methods like 'compareTo()' and 'after()'. ... Read More

1K+ Views
Firstly, you need to import the following package for Calendar class in Javaimport java.util.Calendar;Create a Calendar object and display the current date and timeCalendar calendar = Calendar.getInstance(); System.out.println("Current Date and Time = " + calendar.getTime());Now, let us subtract 1 year using the calendar.add() method and Calendar.YEAR constant. Set a negative value since we are decrementing here −calendar.add(Calendar.YEAR, -1);The following is an exampleExample Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); System.out.println("Current Date = " + calendar.getTime()); // Subtract 1 year from the ... Read More

632 Views
Firstly, you need to import the following package for Calendar class in Javaimport java.util.Calendar;Create a Calendar object and display the current date and timeCalendar calendar = Calendar.getInstance(); System.out.println("Current Date and Time = " + calendar.getTime());Now, let us add 3 months using the calendar.add() method and Calendar.MONTH constant −calendar.add(Calendar.MONTH, 3);The following is an exampleExample Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); System.out.println("Current Date = " + calendar.getTime()); // Add 3 months to the Calendar calendar.add(Calendar.MONTH, 3); ... Read More

1K+ Views
Firstly, you need to import the following package for Calendar class in Javaimport java.util.Calendar;Create a Calendar object and display the current date and timeCalendar calendar = Calendar.getInstance(); System.out.println("Current Date and Time = " + calendar.getTime());Now, let us decrement the year using the calendar.add() method and Calendar.YEAR constant. Set a negative value since we are decrementing herecalendar.add(Calendar.YEAR, -20);The following is an exampleExample Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); System.out.println("Current Date = " + calendar.getTime()); // Subtract 20 Years ... Read More

910 Views
Firstly, you need to import the following package for Calendar class in Javaimport java.util.Calendar;Create a Calendar object and display the current date and timeCalendar calendar = Calendar.getInstance(); System.out.println("Current Date and Time = " + calendar.getTime());Now, let us add year using the calendar.add() method and Calendar.YEAR constantcalendar.add(Calendar.YEAR, 20);The following is an exampleExample Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); System.out.println("Current Date = " + calendar.getTime()); // Add 20 Years calendar.add(Calendar.YEAR, 20); System.out.println("Updated Date = " + ... Read More