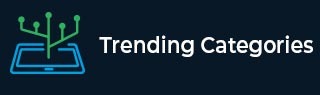
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

136 Views
The java.math.BigInteger class provides operations analogues to all of Java's primitive integer operators and for all relevant methods from java.lang.Math.BigInteger class is used for big integer calculations which are outside the limit of the primitive data types. It provides operations for modular arithmetic, GCD calculation, primality testing, prime generation, bit manipulation, and a few other miscellaneous operations.The following is an example that displays how we can work with BigInteger values.Example Live Demoimport java.math.BigInteger; public class BigIntegerDemo { public static void main(String[] args) { BigInteger bi1, bi2, bi3; // assign values to bi1, bi2 ... Read More

1K+ Views
BigInteger class provides operations for modular arithmetic, GCD calculation, primality testing, prime generation, bit manipulation, and a few other miscellaneous operations.Let’s say the following is our byte array −byte[] arr = new byte[] { 0x1, 0x00, 0x00 };We will now convert them to BigInteger −BigInteger bInteger = new BigInteger(arr);The following is an example that creates BigInteger from a byte array in Java.Example Live Demoimport java.math.BigInteger; public class Demo { public static void main(String[] argv) throws Exception { byte[] arr = new byte[] { 0x1, 0x00, 0x00 }; BigInteger bInteger = new BigInteger(arr); System.out.println(bInteger); } }Output65536

397 Views
BigInteger class is used for big integer calculations which are outside the limit of the primitive data types. It provides operations for modular arithmetic, GCD calculation, primality testing, prime generation, bit manipulation, and a few other miscellaneous operations.Firstly, set a long value −Long l = 198L;Now, create a new object for BigInteger and pass the above value −BigInteger bInteger = new BigInteger(l);The following is an example −Example Live Demoimport java.math.BigInteger; public class Demo { public static void main(String[] argv) throws Exception { Long l = 198L; BigInteger bInteger = BigInteger.valueOf(l); System.out.println(bInteger); } }Output198

107 Views
BigInteger class is used for big integer calculations which are outside the limit of the primitive data types. It provides operations for modular arithmetic, GCD calculation, primality testing, prime generation, bit manipulation, and a few other miscellaneous operations.Firstly, set a string −String str = "268787878787687";Now, create a new object for BigInteger and pass the above string −BigInteger bInteger = new BigInteger(str);The following is an example −Example Live Demoimport java.math.BigInteger; public class Demo { public static void main(String[] argv) throws Exception { String str = "268787878787687"; BigInteger bInteger = new BigInteger(str); System.out.println(bInteger); } }Output268787878787687

158 Views
Enum in Java contains a fixed set of constants. They can have fields, constructors and method. It enhances type safety in Java.The following is an example wherein we are implementing Switch statement on Enumeration in Java −Example Live Demopublic class Demo { public static void main(String[] args) { Laptop l = Laptop.Inspiron; switch(l){ case Inspiron: System.out.println("Laptop for home and office use!"); break; case XPS: System.out.println("Laptop for the ultimate experience!"); break; case Alienware: System.out.println("Laptop for high-performance gaming"); break; } } } enum Laptop { Inspiron, XPS, Alienware; }OutputLaptop for home and office use!

104 Views
We can use the == operator to compare enums in Java.Let’s say we have the following enum.enum Devices { LAPTOP, MOBILE, TABLET; }Here are some of the objects and we have assigned some values as well −Devices d1, d2, d3; d1 = Devices.LAPTOP; d2 = Devices.LAPTOP; d3 = Devices.TABLET;Let us now see an example wherein we will compare them using == operator −Example Live Demopublic class Demo { enum Devices { LAPTOP, MOBILE, TABLET; } public static void main(String[] args) { Devices d1, d2, d3; d1 = Devices.LAPTOP; ... Read More

168 Views
To compare enumeration values, use the equals() method.Our Devices enum is having some objects with values assigned to them.Devices d1, d2, d3; d1 = Devices.LAPTOP; d2 = Devices.LAPTOP; d3 = Devices.TABLET;Let us compare them −if(d3.equals(Devices.TABLET)) System.out.println("Devices are same."); else System.out.println("Devices are different.");The following is an example −Example Live Demopublic class Demo { enum Devices { LAPTOP, MOBILE, TABLET; } public static void main(String[] args) { Devices d1, d2, d3; d1 = Devices.LAPTOP; d2 = Devices.LAPTOP; d3 = Devices.TABLET; if(d1.equals(d2)) ... Read More

112 Views
We have the Devices Enum with four constants.enum Devices { LAPTOP, MOBILE, TABLET, DESKTOP; }We have created some objects and assigned them with the constants.Let us compare them with both equals() and ==. Firstly, begin with equals() −if(d1.equals(d2)) System.out.println("Devices are the same."); else System.out.println("Devices are different.");Now, let us move forward and check for ==if(d1 == d3) System.out.println("Devices are the same."); else System.out.println("Devices are different.");The following is the final example demonstrating both equals() and == operator −Example Live Demopublic class Demo { enum Devices { LAPTOP, MOBILE, TABLET, DESKTOP; } public static void main(String[] args) { ... Read More

2K+ Views
To set enum for days of the week, set them as constantsenum Days { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday }Now create objects and set the above constants −Days today = Days.Wednesday; Days holiday = Days.Sunday;The following is an example −Example Live Demopublic class Demo { enum Days { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday } public static void main(String[] args) { Days today = Days.Wednesday; Days holiday = Days.Sunday; System.out.println("Today = " + today); System.out.println(holiday+ " is holiday"); } }OutputToday = Wednesday Sunday is holiday

466 Views
Firstly, set the day of year using DAY_OF_YEAR constant.Calendar cal = Calendar.getInstance(); cal.set(Calendar.YEAR, 2018); cal.set(Calendar.DAY_OF_YEAR, 320);Now, get the day of month −int res = cal.get(Calendar.DAY_OF_MONTH);The following is an example −Example Live Demoimport java.util.Calendar; public class Demo { public static void main(String[] args) { Calendar cal = Calendar.getInstance(); cal.set(Calendar.YEAR, 2018); cal.set(Calendar.DAY_OF_YEAR, 320); System.out.println("Date = " + cal.getTime()); int res = cal.get(Calendar.DAY_OF_MONTH); System.out.println("Day of month = " + res); } }OutputDate = Fri Nov 16 07:54:55 UTC 2018 Day of month = 16Read More