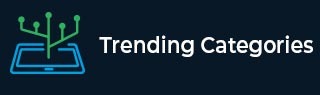
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 9326 Articles for Object Oriented Programming

88 Views
The BigInteger.not() method returns a BigInteger whose value is (~this). This method returns a negative value if and only if this BigInteger is non-negative.The following is an example −Example Live Demoimport java.math.*; public class Demo { public static void main(String[] args) { BigInteger one, two, three; one = new BigInteger("6"); two = one.not(); System.out.println("Result (not operation): " +two); } }OutputResult (not operation): -7Let us see another example −Example Live Demoimport java.math.*; public class Demo { public static void main(String[] args) { BigInteger bi1, bi2, ... Read More

112 Views
The java.math.BigInteger.and(BigInteger val) returns a BigInteger whose value is (this & val). This method returns a negative BigInteger if and only if this and val are both negative. Here, “val” is the value to be AND'ed with this BigInteger.First, create BigInteger objects −BigInteger one, two, three; one = new BigInteger("12"); two = new BigInteger("6");Perform AND operation on first and second object −three = one.and(two);The following is an example −Example Live Demoimport java.math.*; public class Demo { public static void main(String[] args) { BigInteger one, two, three; one = new BigInteger("12"); two ... Read More

135 Views
The java.math.BigInteger.xor(BigInteger val) returns a BigInteger whose value is (this ^ val). This method returns a negative BigInteger if and only if exactly one of this and val are negative. Here, “val” is the value to be XOR'ed with this BigInteger.Firstly, create two BigInteger objects −one = new BigInteger("6"); two = new BigInteger("-5");Now, perform XOR −three = one.xor(two);The following is an example −Example Live Demoimport java.math.*; public class Demo { public static void main(String[] args) { BigInteger one, two, three; one = new BigInteger("6"); two = new BigInteger("-5"); ... Read More

149 Views
To shift right in a BigInteger, use the shiftRight() method.The java.math.BigInteger.shiftRight(int n) returns a BigInteger whose value is (this >> n). Sign extension is performed. The shift distance, n, may be negative, in which case this method performs a left shift. It computes floor(this / 2n).The following is an example −Example Live Demoimport java.math.*; public class Demo { public static void main(String[] args) { BigInteger one; one = new BigInteger("25"); one = one.shiftRight(3); System.out.println("Result: " +one); } }OutputResult: 3

209 Views
BigInteger class is used for big integer calculations which are outside the limit of the primitive data types. It provides operations for modular arithmetic, GCD calculation, primality testing, prime generation, bit manipulation, and a few other miscellaneous operations.Let us work with the testBit() method in Java to perform Bitwise operation. The java.math.BigInteger.testBit(int n) returns true if and only if the designated bit is set −The following is an example −Example Live Demoimport java.math.*; public class BigIntegerDemo { public static void main(String[] args) { BigInteger one; Boolean two; one = new BigInteger("5"); ... Read More

172 Views
Use the BigInteger pow() method in Java to calculate the power on a BigInteger.First, let us create some objects.BigInteger one, two; one = new BigInteger("5");Perform the power operation and assign it to the second object −// power operation two = one.pow(3);The following is an example −Example Live Demoimport java.math.*; public class BigIntegerDemo { public static void main(String[] args) { BigInteger one, two; one = new BigInteger("5"); System.out.println("Actual Value: " +one); // power operation two = one.pow(3); System.out.println("Result: " +two); } }OutputActual Value: 5 Negated Value: 125

138 Views
Use the BigInteger negate() method in Java to negate a BigInteger.First, let us create an object −BigInteger one, two; one = new BigInteger("200");Negate the above and assign it to the second object −two = one.negate();The following is an example −Example Live Demoimport java.math.*; public class BigIntegerDemo { public static void main(String[] args) { BigInteger one, two; one = new BigInteger("200"); System.out.println("Actual Value: " +one); // negate two = one.negate(); System.out.println("Negated Value: " +two); } }OutputActual Value: 200 Negated Value: -200

101 Views
Use the BigInteger divide() method in Java to divide one BigInteger from another.First, let us create some objects.BigInteger one, two, three; one = new BigInteger("200"); two = new BigInteger("100");Divide the above and assign it to the third object −three = one.divide(two);The following is an example −Example Live Demoimport java.math.*; public class BigIntegerDemo { public static void main(String[] args) { BigInteger one, two, three; one = new BigInteger("200"); two = new BigInteger("100"); // division three = one.divide(two); String res = one + " / " + two + " = " +three; System.out.println("Result: " +res); } }OutputResult: 200 / 100 = 2

101 Views
Use the BigInteger subtract() method in Java to Subtract one BigInteger from another.First, let us create some objects −BigInteger one, two, three; one = new BigInteger("200"); two = new BigInteger("150");Subtract the above and assign it to the third object −three = one.subtract(two);The following is an example −Example Live Demoimport java.math.*; public class BigIntegerDemo { public static void main(String[] args) { BigInteger one, two, three; one = new BigInteger("200"); two = new BigInteger("150"); three = one.subtract(two); String res = one + " - " + two + " = " +three; System.out.println("Subtraction: " +res); } }OutputSubtraction: 200 - 150 = 50

379 Views
BigInteger class is used for big integer calculations which are outside the limit of the primitive data types. It provides operations for modular arithmetic, GCD calculation, primality testing, prime generation, bit manipulation, and a few other miscellaneous operations.To multiply one BigInteger to another, use the BigInteger multiply() method.First, let us create some objects −BigInteger one, two, three; one = new BigInteger("2"); two = new BigInteger("8");Multiply the above and assign it to the third object −three = one.multiply(two);The following is an example −Example Live Demoimport java.math.*; public class BigIntegerDemo { public static void main(String[] args) { BigInteger one, ... Read More